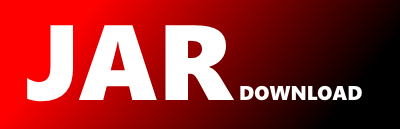
io.quarkus.redis.datasource.graph.ReactiveTransactionalGraphCommands Maven / Gradle / Ivy
Show all versions of quarkus-redis-client Show documentation
package io.quarkus.redis.datasource.graph;
import java.time.Duration;
import io.quarkus.redis.datasource.ReactiveTransactionalRedisCommands;
import io.smallrye.mutiny.Uni;
/**
* Allows executing commands from the {@code graph} group.
* These commands require the Redis Graph module to be installed in the
* Redis server.
*
* See the graph command list for further information about
* these commands.
*
* This API is intended to be used in a Redis transaction ({@code MULTI}), thus, all command methods return {@code Uni}.
*
* @param the type of the key
*/
public interface ReactiveTransactionalGraphCommands extends ReactiveTransactionalRedisCommands {
/**
* Execute the command GRAPH.DELETE.
* Summary: Completely removes the graph and all of its entities.
* Group: graph
*
* @param key the key, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction,
* a failure otherwise. In the case of failure, the transaction is discarded.
*/
Uni graphDelete(K key);
/**
* Execute the command GRAPH.EXPLAIN.
* Summary: Constructs a query execution plan but does not run it. Inspect this execution plan to better understand
* how your query will get executed.
* Group: graph
*
*
* @param key the key, must not be {@code null}
* @param query the query, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction,
* a failure otherwise. In the case of failure, the transaction is discarded.
*/
Uni graphExplain(K key, String query);
/**
* Execute the command GRAPH.LIST.
* Summary: Lists all graph keys in the keyspace.
* Group: graph
*
*
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction,
* a failure otherwise. In the case of failure, the transaction is discarded.
*/
Uni graphList();
/**
* Execute the command GRAPH.QUERY.
* Summary: Executes the given query against a specified graph.
* Group: graph
*
*
* @param key the key, must not be {@code null}
* @param query the query, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction,
* a failure otherwise. In the case of failure, the transaction is discarded.
*/
Uni graphQuery(K key, String query);
/**
* Execute the command GRAPH.QUERY.
* Summary: Executes the given query against a specified graph.
* Group: graph
*
*
* @param key the key, must not be {@code null}
* @param query the query, must not be {@code null}
* @param timeout a timeout, must not be {@code null}
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction,
* a failure otherwise. In the case of failure, the transaction is discarded.
*/
Uni graphQuery(K key, String query, Duration timeout);
}