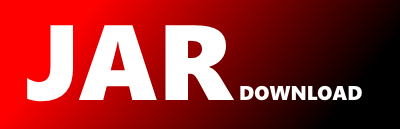
io.quarkus.redis.datasource.hash.TransactionalHashCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.hash;
import java.util.Map;
import io.quarkus.redis.datasource.TransactionalRedisCommands;
public interface TransactionalHashCommands extends TransactionalRedisCommands {
/**
* Execute the command HDEL.
* Summary: Delete one or more hash fields
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
*/
void hdel(K key, F... fields);
/**
* Execute the command HEXISTS.
* Summary: Determine if a hash field exists
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param field the value
*/
void hexists(K key, F field);
/**
* Execute the command HGET.
* Summary: Get the value of a hash field
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param field the value
*/
void hget(K key, F field);
/**
* Execute the command HINCRBY.
* Summary: Increment the integer value of a hash field by the given number
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param field the value
*/
void hincrby(K key, F field, long amount);
/**
* Execute the command HINCRBYFLOAT.
* Summary: Increment the float value of a hash field by the given amount
* Group: hash
* Requires Redis 2.6.0
*
* @param key the key
* @param field the value
*/
void hincrbyfloat(K key, F field, double amount);
/**
* Execute the command HGETALL.
* Summary: Get all the fields and values in a hash
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
*/
void hgetall(K key);
/**
* Execute the command HKEYS.
* Summary: Get all the fields in a hash
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
*/
void hkeys(K key);
/**
* Execute the command HLEN.
* Summary: Get the number of fields in a hash
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
*/
void hlen(K key);
/**
* Execute the command HMGET.
* Summary: Get the values of all the given hash fields
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param fields the fields
*/
void hmget(K key, F... fields);
/**
* Execute the command HMSET.
* Summary: Set multiple hash fields to multiple values
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param map the key/value map to set
* @deprecated Use {@link #hset(Object, Map)} with multiple field-value pairs.
*/
@Deprecated
void hmset(K key, Map map);
/**
* Execute the command HRANDFIELD.
* Summary: Get one or multiple random fields from a hash
* Group: hash
* Requires Redis 6.2.0
*
* @param key the key
*/
void hrandfield(K key);
/**
* Execute the command HRANDFIELD.
* Summary: Get one or multiple random fields from a hash
* Group: hash
* Requires Redis 6.2.0
*
* @param key the key
* @param count the number of random key to retrieve. If {@code count} is positive, the selected keys are distinct.
* If {@code count} is negative, the produced list can contain duplicated keys.
*/
void hrandfield(K key, long count);
/**
* Execute the command HRANDFIELD.
* Summary: Get one or multiple random fields and their associated values from a hash
* Group: hash
* Requires Redis 6.2.0
*
* @param key the key
* @param count the number of random key to retrieve. If {@code count} is positive, the selected keys are distinct.
* If {@code count} is negative, the produced list can contain duplicated keys. These duplicates are
* not included in the produced {@code Map}.
*/
void hrandfieldWithValues(K key, long count);
/**
* Execute the command HSET.
* Summary: Set the string value of a hash field
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param field the field
* @param value the value
*/
void hset(K key, F field, V value);
/**
* Execute the command HSET.
* Summary: Set the string value of a hash field
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param map the set of key -> value to add to the hash
*/
void hset(K key, Map map);
/**
* Execute the command HSETNX.
* Summary: Set the value of a hash field, only if the field does not exist
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
* @param field the value
* @param value the value
*/
void hsetnx(K key, F field, V value);
/**
* Execute the command HSTRLEN.
* Summary: Get the length of the value of a hash field
* Group: hash
* Requires Redis 3.2.0
*
* @param key the key
* @param field the value
*/
void hstrlen(K key, F field);
/**
* Execute the command HVALS.
* Summary: Get all the values in a hash
* Group: hash
* Requires Redis 2.0.0
*
* @param key the key
*/
void hvals(K key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy