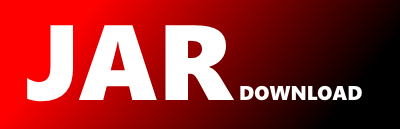
io.quarkus.redis.datasource.json.JsonSetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.json;
import java.util.ArrayList;
import java.util.List;
import io.quarkus.redis.datasource.RedisCommandExtraArguments;
public class JsonSetArgs implements RedisCommandExtraArguments {
private boolean nx = false;
private boolean xx = false;
/**
* Don't update already existing elements. Always add new elements.
*
* @return the current {@code GeoaddArgs}
**/
public JsonSetArgs nx() {
this.nx = true;
return this;
}
/**
* Only update elements that already exist. Never add elements.
*
* @return the current {@code GeoaddArgs}
**/
public JsonSetArgs xx() {
this.xx = true;
return this;
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy