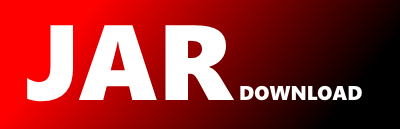
io.quarkus.redis.datasource.json.ReactiveJsonCommands Maven / Gradle / Ivy
Show all versions of quarkus-redis-client Show documentation
package io.quarkus.redis.datasource.json;
import java.util.Collections;
import java.util.List;
import io.quarkus.redis.datasource.ReactiveRedisCommands;
import io.smallrye.mutiny.Uni;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
/**
* Allows executing commands from the {@code json} group (requires the Redis stack).
* See the json command list for further information about these commands.
*
* Redis JSON lets you store, update, and retrieve JSON values in a Redis database, similar to any other Redis data type.
*
* Ths API is based on the {@link JsonObject} and {@link JsonArray} types.
* Some methods also allows direct mapping from and to objects. In the case of deserialization, the
* {@link Class Class} must be passed.
*
* @param the type of the key
*/
public interface ReactiveJsonCommands extends ReactiveRedisCommands {
/**
* Execute the command JSON.SET.
* Summary: Sets the JSON value at path in key.
* Group: json
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param value the value, encoded to JSON
* @param the type for the value
* @return A uni emitting {@code null} when the operation completes
**/
Uni jsonSet(K key, String path, T value);
/**
* Execute the command JSON.SET.
* Summary: Sets the JSON value at path in key.
* Group: json
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param json the JSON object to store, must not be {@code null}
* @return A uni emitting {@code null} when the operation completes
**/
Uni jsonSet(K key, String path, JsonObject json);
/**
* Execute the command JSON.SET.
* Summary: Sets the JSON value at path in key.
* Group: json
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param json the JSON object to store, must not be {@code null}
* @param args the extra arguments
* @return A uni emitting {@code null} when the operation completes
**/
Uni jsonSet(K key, String path, JsonObject json, JsonSetArgs args);
/**
* Execute the command JSON.SET.
* Summary: Sets the JSON value at path in key.
* Group: json
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param json the JSON array to store, must not be {@code null}
* @return A uni emitting {@code null} when the operation completes
**/
Uni jsonSet(K key, String path, JsonArray json);
/**
* Execute the command JSON.SET.
* Summary: Sets the JSON value at path in key.
* Group: json
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param json the JSON array to store, must not be {@code null}
* @param args the extra arguments
* @return A uni emitting {@code null} when the operation completes
**/
Uni jsonSet(K key, String path, JsonArray json, JsonSetArgs args);
/**
* Execute the command JSON.SET.
* Summary: Sets the JSON value at path in key.
* Group: json
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param value the value to store, encoded to JSON.
* @param args the extra arguments
* @return A uni emitting {@code null} when the operation completes
**/
Uni jsonSet(K key, String path, T value, JsonSetArgs args);
/**
* Execute the command JSON.GET.
* Summary: Returns the value at path in JSON serialized form.
* Group: json
*
* This method uses the root path ({@code $}).
* It map the retrieve JSON document to an object of type {@code }.
*
* @param key the key, must not be {@code null}
* @param clazz the type of object to recreate from the JSON content
* @return a uni emitting the object, {@code null} if it does not exist
**/
Uni jsonGet(K key, Class clazz);
/**
* Execute the command JSON.GET.
* Summary: Returns the value at path in JSON serialized form.
* Group: json
*
* This method uses the root path ({@code $}).
* Unlike {@link #jsonGet(Object, Class)}, it returns a {@link JsonObject}.
*
* @param key the key, must not be {@code null}
* @return a uni emitting the stored JSON object, {@code null} if it does not exist
**/
Uni jsonGetObject(K key);
/**
* Execute the command JSON.GET.
* Summary: Returns the value at path in JSON serialized form.
* Group: json
*
* This method uses the root path ({@code $}).
* Unlike {@link #jsonGet(Object, Class)}, it returns a {@link JsonArray}.
*
* @param key the key, must not be {@code null}
* @return a uni emitting the stored JSON array, {@code null} if it does not exist
**/
Uni jsonGetArray(K key);
/**
* Execute the command JSON.GET.
* Summary: Returns the value at path in JSON serialized form.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @return a uni emitting the JSON array containing the different results, {@code null} if it does not exist.
**/
Uni jsonGet(K key, String path);
/**
* Execute the command JSON.GET.
* Summary: Returns the value at path in JSON serialized form.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param paths the paths, must not be {@code null}. If no path are passed, this is equivalent to
* {@link #jsonGetObject(Object)},
* if multiple paths are passed, the produced JSON object contains the result (as a json array) for each path.
* @return a uni emitting the stored JSON object, {@code null} if it does not exist.
* If no path are passed, this is equivalent to {@link #jsonGetObject(Object)}.
* If multiple paths are passed, the produced JSON object contains the result for each pass as a JSON array.
**/
Uni jsonGet(K key, String... paths);
/**
* Execute the command JSON.ARRAPPEND.
* Summary: Append the json values into the array at path after the last element in it.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param values the values to append, encoded to JSON
* @param the type of value
* @return a uni emitting a list with the new sizes of each modified array (in order) or {@code null} (instead of
* the size) if the point object was not an array.
**/
Uni> jsonArrAppend(K key, String path, T... values);
/**
* Execute the command JSON.ARRINDEX.
* Summary: Searches for the first occurrence of a scalar JSON value in an array.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param value the value to be searched, encoded to JSON
* @param start the start index
* @param end the end index
* @param the type of value
* @return a uni emitting a list with the first position in the array of each JSON value that matches the path,
* {@code -1} if not found in the array, or {@code null} if the matching JSON value is not an array.
**/
Uni> jsonArrIndex(K key, String path, T value, int start, int end);
/**
* Execute the command JSON.ARRINDEX.
* Summary: Searches for the first occurrence of a scalar JSON value in an array.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param value the value to be searched, encoded to JSON
* @param the type of value
* @return a uni emitting a list with the first position in the array of each JSON value that matches the path,
* {@code -1} if not found in the array, or {@code null} if the matching JSON value is not an array.
**/
default Uni> jsonArrIndex(K key, String path, T value) {
return jsonArrIndex(key, path, value, 0, 0);
}
/**
* Execute the command JSON.ARRINSERT.
* Summary: Inserts the json values into the array at path before the index (shifts to the right).
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path the path, must not be {@code null}
* @param index the index. The index must be in the array's range. Inserting at index 0 prepends to the array.
* Negative index values start from the end of the array.
* @param values the values to insert, encoded to JSON
* @param the type of value
* @return a uni emitting a list of integer containing for each path, the array's new size or {@code null} if the
* matching JSON value is not an array.
**/
Uni> jsonArrInsert(K key, String path, int index, T... values);
/**
* Execute the command JSON.ARRLEN.
* Summary: Reports the length of the JSON Array at path in key.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path the path, {@code null} means {@code $}
* @return a uni emitting a list of integer containing for each path, the array's length, or @{code null} if the
* matching JSON value is not an array.
**/
Uni> jsonArrLen(K key, String path);
/**
* Execute the command JSON.ARRLEN.
* Summary: Reports the length of the JSON Array at path in key.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @return a uni emitting the array's length, or @{code null} if the matching JSON value is not an array.
**/
default Uni jsonArrLen(K key) {
return jsonArrLen(key, null).map(l -> l.get(0));
}
/**
* Execute the command JSON.ARRPOP.
* Summary: Removes and returns an element from the index in the array.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param clazz the type of the popped object
* @param path path the path, defaults to root if not provided.
* @param index is the position in the array to start popping from (defaults to -1, meaning the last element).
* Out-of-range indexes round to their respective array ends.
* @return a uni emitting a list of T including for each path, an instance of T rebuilt from the JSON value, or
* {@code null} if the matching JSON value is not an array. Popping an empty array produces {@code null}.
**/
Uni> jsonArrPop(K key, Class clazz, String path, int index);
/**
* Execute the command JSON.ARRPOP.
* Summary: Removes and returns an element from the index in the array.
* Group: json
*
*
* This variant popped from the root object (must be a JSON array), and returns a single item.
*
* @param key the key, must not be {@code null}
* @param clazz the type of the popped object
* @return a uni emitting an instance of T.
**/
default Uni jsonArrPop(K key, Class clazz) {
return jsonArrPop(key, clazz, null, -1).map(l -> l.get(0));
}
/**
* Execute the command JSON.ARRTRIM.
* Summary: Trims an array so that it contains only the specified inclusive range of elements.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, must not be {@code null}
* @param start the start index
* @param stop the stop index
* @return a uni emitting a list of integer containing, for each path, the array's new size, or {@code null} if
* the matching JSON value is not an array.
**/
Uni> jsonArrTrim(K key, String path, int start, int stop);
/**
* Execute the command JSON.CLEAR.
* Summary: Clears container values (Arrays/Objects), and sets numeric values to 0.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided. Non-existing paths are ignored.
* @return a uni emitting the number of value cleared
**/
Uni jsonClear(K key, String path);
/**
* Execute the command JSON.CLEAR.
* Summary: Clears container values (Arrays/Objects), and sets numeric values to 0.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @return a uni emitting the number of value cleared
**/
default Uni jsonClear(K key) {
return jsonClear(key, null);
}
/**
* Execute the command JSON.DEL.
* Summary: Deletes a value.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided. Non-existing paths are ignored.
* @return a uni emitting the number of path deleted
**/
Uni jsonDel(K key, String path);
/**
* Execute the command JSON.DEL.
* Summary: Deletes a value.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @return a uni emitting the number of path deleted (0 or more)
**/
default Uni jsonDel(K key) {
return jsonDel(key, null);
}
/**
* Execute the command JSON.MGET.
* Summary: Returns the values at path from multiple key arguments. Returns {@code null} for nonexistent keys
* and nonexistent paths.
* Group: json
*
*
* @param path path the path
* @param keys the keys, must not be {@code null}, must not contain {@code null}
* @return a uni emitting a list of JsonArray containing each retrieved value
**/
Uni> jsonMget(String path, K... keys);
/**
* Execute the command JSON.NUMINCRBY.
* Summary: Increments the number value stored at path by number.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided. Non-existing paths are ignored.
* @param value the value to add
* @return a uni emitting {@code null} when the operation completes
**/
Uni jsonNumincrby(K key, String path, double value);
/**
* Execute the command JSON.OBJKEYS.
* Summary: Returns the keys in the object that's referenced by path.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided.
* @return a uni emitting a list containing, for each matching path, the list of keys, or {@code null} if the
* matching JSON value is not an object.
**/
Uni>> jsonObjKeys(K key, String path);
/**
* Execute the command JSON.OBJKEYS.
* Summary: Returns the keys in the object that's referenced by path.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @return a uni emitting a list containing the list of keys, or {@code null} if the stored value is not a JSON object.
**/
default Uni> jsonObjKeys(K key) {
return jsonObjKeys(key, null).map(l -> {
if (l != null && !l.isEmpty()) {
return l.get(0);
}
return Collections.emptyList();
});
}
/**
* Execute the command JSON.OBJLEN.
* Summary: Reports the number of keys in the JSON Object at path in key.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided.
* @return a uni emitting a list containing, for each path, the length of the object, {@code null} if the matching
* JSON value is not an object
**/
Uni> jsonObjLen(K key, String path);
/**
* Execute the command JSON.OBJLEN.
* Summary: Reports the number of keys in the JSON Object at path in key.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @return a uni emitting the length of the JSON object, {@code null} if the matching JSON value is not an object
**/
default Uni jsonObjLen(K key) {
return jsonObjLen(key, null)
.map(l -> l.get(0));
}
/**
* Execute the command JSON.STRAPPEND.
* Summary: Appends the json-string values to the string at path.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided.
* @param value the string to append, must not be {@code null}
* @return a uni emitting a list containing, for each path, the new string length, {@code null} if the matching JSON
* value is not a string.
**/
Uni> jsonStrAppend(K key, String path, String value);
/**
* Execute the command JSON.STRLEN.
* Summary: Reports the length of the JSON String at path in key.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided.
* @return a uni emitting a list containing, for each path, the length of the string, {@code null} if the matching JSON
* value is not a string. Returns {@code null} if the key or path do not exist.
**/
Uni> jsonStrLen(K key, String path);
/**
* Execute the command JSON.TOGGLE.
* Summary: Toggle a boolean value stored at path.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, must not be {@code null}
* @return a uni emitting a list containing, for each path, the new boolean value, {@code null} if the matching JSON
* value is not a boolean.
**/
Uni> jsonToggle(K key, String path);
/**
* Execute the command JSON.TYPE.
* Summary: Reports the type of JSON value at path.
* Group: json
*
*
* @param key the key, must not be {@code null}
* @param path path the path, path defaults to {@code $} if not provided.
* @return a uni emitting a list containing, for each path, the json type as String (string, integer, number, boolean,
* object, array), empty if no match.
**/
Uni> jsonType(K key, String path);
}