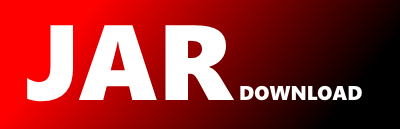
io.quarkus.redis.datasource.keys.CopyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.keys;
import java.util.ArrayList;
import java.util.List;
import io.quarkus.redis.datasource.RedisCommandExtraArguments;
/**
* Arguments for the Redis COPY command.
*/
public class CopyArgs implements RedisCommandExtraArguments {
private long destinationDb = -1;
private boolean replace;
/**
* Specify an alternative logical database index for the destination key.
*
* @param destinationDb logical database index to apply for {@literal DB}.
* @return the current {@code CopyArgs}.
*/
public CopyArgs destinationDb(long destinationDb) {
this.destinationDb = destinationDb;
return this;
}
/**
* Hint redis to remove the destination key before copying the value to it.
*
* @param replace remove destination key before copying the value {@literal REPLACE}.
* @return {@code this}.
*/
public CopyArgs replace(boolean replace) {
this.replace = replace;
return this;
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy