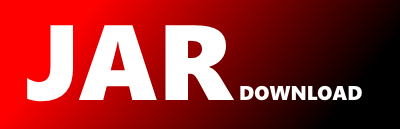
io.quarkus.redis.datasource.list.ReactiveTransactionalListCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.list;
import java.time.Duration;
import io.quarkus.redis.datasource.ReactiveTransactionalRedisCommands;
import io.smallrye.mutiny.Uni;
public interface ReactiveTransactionalListCommands extends ReactiveTransactionalRedisCommands {
/**
* Execute the command BLMOVE.
* Summary: Pop an element from a list, push it to another list and return it; or block until one is available
* Group: list
* Requires Redis 6.2.0
*
* @param source the key
* @param destination the key
* @param positionInSource the position of the element in the source, {@code LEFT} means the first element, {@code RIGHT}
* means the last element.
* @param positionInDest the position of the element in the destination, {@code LEFT} means the first element, {@code RIGHT}
* means the last element.
* @param timeout the operation timeout (in seconds)
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni blmove(K source, K destination, Position positionInSource, Position positionInDest, Duration timeout);
/**
* Execute the command BLMPOP.
* Summary: Pop elements from a list, or block until one is available
* Group: list
* Requires Redis 7.0.0
*
* @param timeout the operation timeout (in seconds)
* @param position whether if the element must be popped from the beginning of the list ({@code LEFT}) or from the end
* ({@code RIGHT})
* @param keys the keys from which the element must be popped
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni blmpop(Duration timeout, Position position, K... keys);
/**
* Execute the command BLMPOP.
* Summary: Pop elements from a list, or block until one is available
* Group: list
* Requires Redis 7.0.0
*
* @param timeout the operation timeout (in seconds)
* @param position whether if the element must be popped from the beginning of the list ({@code LEFT}) or from the end
* ({@code RIGHT})
* @param count the number of element to pop
* @param keys the keys from which the element must be popped
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni blmpop(Duration timeout, Position position, int count, K... keys);
/**
* Execute the command BLPOP.
* Summary: Remove and get the first element in a list, or block until one is available
* Group: list
* Requires Redis 2.0.0
*
* @param timeout the operation timeout (in seconds)
* @param keys the keys from which the element must be popped
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni blpop(Duration timeout, K... keys);
/**
* Execute the command BRPOP.
* Summary: Remove and get the last element in a list, or block until one is available
* Group: list
* Requires Redis 2.0.0
*
* @param timeout the operation timeout (in seconds)
* @param keys the keys from which the element must be popped
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni brpop(Duration timeout, K... keys);
/**
* Execute the command BRPOPLPUSH.
* Summary: Pop an element from a list, push it to another list and return it; or block until one is available
* Group: list
* Requires Redis 2.2.0
*
* @param timeout the timeout, in seconds
* @param source the source key
* @param destination the detination key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/brpoplpush
*/
@Deprecated
Uni brpoplpush(Duration timeout, K source, K destination);
/**
* Execute the command LINDEX.
* Summary: Get an element from a list by its index
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param index the index
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lindex(K key, long index);
/**
* Execute the command LINSERT.
* Summary: Insert an element before another element in a list
* Group: list
* Requires Redis 2.2.0
*
* @param key the key
* @param pivot the pivot, i.e. the position reference
* @param element the element to insert
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni linsertBeforePivot(K key, V pivot, V element);
/**
* Execute the command LINSERT.
* Summary: Insert an element after another element in a list
* Group: list
* Requires Redis 2.2.0
*
* @param key the key
* @param pivot the pivot, i.e. the position reference
* @param element the element to insert
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni linsertAfterPivot(K key, V pivot, V element);
/**
* Execute the command LLEN.
* Summary: Get the length of a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni llen(K key);
/**
* Execute the command LMOVE.
* Summary: Pop an element from a list, push it to another list and return it
* Group: list
* Requires Redis 6.2.0
*
* @param source the key
* @param destination the key
* @param positionInSource the position of the element to pop in the source (LEFT: first element, RIGHT: last element)
* @param positionInDestination the position of the element to insert in the destination (LEFT: first element, RIGHT: last
* element)
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lmove(K source, K destination, Position positionInSource, Position positionInDestination);
/**
* Execute the command LMPOP.
* Summary: Pop one element from the first non-empty list
* Group: list
* Requires Redis 7.0.0
*
* @param position the position of the item to pop (LEFT: beginning ot the list, RIGHT: end of the list)
* @param keys the keys from which the item will be popped, must not be empty
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lmpop(Position position, K... keys);
/**
* Execute the command LMPOP.
* Summary: Pop {@code count} elements from the first non-empty list
* Group: list
* Requires Redis 7.0.0
*
* @param position the position of the item to pop (LEFT: beginning ot the list, RIGHT: end of the list)
* @param count the number of items to pop
* @param keys the keys from which the item will be popped, must not be empty
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lmpop(Position position, int count, K... keys);
/**
* Execute the command LPOP.
* Summary: Remove and get the first elements in a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpop(K key);
/**
* Execute the command LPOP.
* Summary: Remove and get the first elements in a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param count the number of element to pop
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpop(K key, int count);
/**
* Execute the command LPOS.
* Summary: Return the index of matching elements on a list
* Group: list
* Requires Redis 6.0.6
*
* @param key the key
* @param element the element to find
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpos(K key, V element);
/**
* Execute the command LPOS.
* Summary: Return the index of matching elements on a list
* Group: list
* Requires Redis 6.0.6
*
* @param key the key
* @param element the element to find
* @param args the extra command parameter
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpos(K key, V element, LPosArgs args);
/**
* Execute the command LPOS.
* Summary: Return the index of matching elements on a list
* Group: list
* Requires Redis 6.0.6
*
* @param key the key
* @param element the element to find
* @param count the number of occurrence to find
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpos(K key, V element, int count);
/**
* Execute the command LPOS.
* Summary: Return the index of matching elements on a list
* Group: list
* Requires Redis 6.0.6
*
* @param key the key
* @param element the element to find
* @param count the number of occurrence to find
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpos(K key, V element, int count, LPosArgs args);
/**
* Execute the command LPUSH.
* Summary: Prepend one or multiple elements to a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param elements the elements to add
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpush(K key, V... elements);
/**
* Execute the command LPUSHX.
* Summary: Prepend an element to a list, only if the list exists
* Group: list
* Requires Redis 2.2.0
*
* @param key the key
* @param elements the elements to add
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lpushx(K key, V... elements);
/**
* Execute the command LRANGE.
* Summary: Get a range of elements from a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param start the starting position
* @param stop the last position
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lrange(K key, long start, long stop);
/**
* Execute the command LREM.
* Summary: Remove elements from a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param count the number of occurence to remove, following the given rules:
* if count > 0: Remove elements equal to element moving from head to tail.
* if count < 0: Remove elements equal to element moving from tail to head.
* if count = 0: Remove all elements equal to element.
* @param element the element to remove
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lrem(K key, long count, V element);
/**
* Execute the command LSET.
* Summary: Set the value of an element in a list by its index
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param index the index
* @param element the element to insert
*/
Uni lset(K key, long index, V element);
/**
* Execute the command LTRIM.
* Summary: Trim a list to the specified range
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param start the starting index
* @param stop the last index
*/
Uni ltrim(K key, long start, long stop);
/**
* Execute the command RPOP.
* Summary: Remove and get the last elements in a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni rpop(K key);
/**
* Execute the command RPOP.
* Summary: Remove and get the last elements in a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param count the number of element to pop
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni rpop(K key, int count);
/**
* Execute the command RPOPLPUSH.
* Summary: Remove the last element in a list, prepend it to another list and return it
* Group: list
* Requires Redis 1.2.0
*
* @param source the key
* @param destination the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/rpoplpush
*/
@Deprecated
Uni rpoplpush(K source, K destination);
/**
* Execute the command RPUSH.
* Summary: Append one or multiple elements to a list
* Group: list
* Requires Redis 1.0.0
*
* @param key the key
* @param values the values to add to the list
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni rpush(K key, V... values);
/**
* Execute the command RPUSHX.
* Summary: Append an element to a list, only if the list exists
* Group: list
* Requires Redis 2.2.0
*
* @param key the key
* @param values the values to add to the list
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni rpushx(K key, V... values);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy