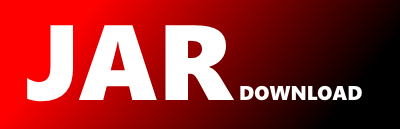
io.quarkus.redis.datasource.pubsub.PubSubCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.pubsub;
import java.util.List;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import io.quarkus.redis.datasource.RedisCommands;
/**
* Allows executing Pub/Sub commands.
* See the pub/sub documentation.
*
* @param the class of the exchanged messages.
*/
public interface PubSubCommands extends RedisCommands {
/**
* Publishes a message to a given channel
*
* @param channel the channel
* @param message the message
*/
void publish(String channel, V message);
/**
* Subscribes to a given channel.
*
* @param channel the channel
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the given
* channel, and is invoked on the I/O thread. So, you must not block. Offload to
* a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribe(String channel, Consumer onMessage);
/**
* Subscribes to a given pattern like {@code chan*l}.
*
* @param pattern the pattern
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPattern(String pattern, Consumer onMessage);
/**
* Same as {@link #subscribeToPattern(String, Consumer)}, but instead of receiving only the message payload, it
* also receives the name of the channel.
*
* @param pattern the pattern
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed. The first parameter is the name of the
* channel. The second parameter is the payload.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPattern(String pattern, BiConsumer onMessage);
/**
* Subscribes to the given patterns like {@code chan*l}.
*
* @param patterns the patterns
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPatterns(List patterns, Consumer onMessage);
/**
* Same as {@link #subscribeToPatterns(List, Consumer)}, but instead of only receiving the payload, it also receives
* the channel name.
*
* @param patterns the patterns
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed. The first parameter is the channel name.
* The second one if the payload.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPatterns(List patterns, BiConsumer onMessage);
/**
* Subscribes to the given channels.
*
* @param channels the channels
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the given
* channels, and is invoked on the I/O thread. So, you must not block. Offload to
* a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribe(List channels, Consumer onMessage);
/**
* Subscribes to a given channel.
*
* @param channel the channel
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the given
* channel, and is invoked on the I/O thread. So, you must not block. Offload to
* a separate thread if needed.
* @param onEnd the end handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @param onException the exception handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribe(String channel, Consumer onMessage, Runnable onEnd,
Consumer onException);
/**
* Subscribes to a given pattern like {@code chan*l}.
*
* @param pattern the pattern
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed.
* @param onEnd the end handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @param onException the exception handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPattern(String pattern, Consumer onMessage, Runnable onEnd,
Consumer onException);
/**
* Same as {@link #subscribeToPatterns(List, Consumer, Runnable, Consumer)}, but also receives the channel name.
*
* @param pattern the pattern
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed. The first parameter is the name of the
* channel. The second parameter is the payload.
* @param onEnd the end handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @param onException the exception handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPattern(String pattern, BiConsumer onMessage, Runnable onEnd,
Consumer onException);
/**
* Subscribes to the given patterns like {@code chan*l}.
*
* @param patterns the patterns
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed.
* @param onEnd the end handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @param onException the exception handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPatterns(List patterns, Consumer onMessage, Runnable onEnd,
Consumer onException);
/**
* Same as {@link #subscribeToPatterns(List, Consumer, Runnable, Consumer)}, but also receive the channel name.
*
* @param patterns the patterns
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the
* channels matching the pattern, and is invoked on the I/O thread. So, you must
* not block. Offload to a separate thread if needed. The first parameter is the name of the
* channel. The second parameter is the payload.
* @param onEnd the end handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @param onException the exception handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribeToPatterns(List patterns, BiConsumer onMessage, Runnable onEnd,
Consumer onException);
/**
* Subscribes to the given channels.
*
* @param channels the channels
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the given
* channels, and is invoked on the I/O thread. So, you must not block. Offload to
* a separate thread if needed.
* @param onEnd the end handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @param onException the exception handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribe(List channels, Consumer onMessage, Runnable onEnd,
Consumer onException);
/**
* Same as {@link #subscribe(List, Consumer, Runnable, Consumer)} but also receives the channel name.
*
* @param channels the channels
* @param onMessage the message consumer. Be aware that this callback is invoked for each message sent to the given
* channels, and is invoked on the I/O thread. So, you must not block. Offload to
* a separate thread if needed. The first parameter is the name of the channel. The second
* parameter is the payload.
* @param onEnd the end handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @param onException the exception handler. Be aware that this callback is invoked on the I/O thread.
* So, you must not block. Offload to a separate thread if needed.
* @return the subscriber object that lets you unsubscribe
*/
RedisSubscriber subscribe(List channels, BiConsumer onMessage, Runnable onEnd,
Consumer onException);
/**
* A redis subscriber
*/
interface RedisSubscriber {
/**
* Unsubscribes from the given channels/patterns
*
* @param channels the channels or patterns
*/
void unsubscribe(String... channels);
/**
* Unsubscribes from all the subscribed channels/patterns
*/
void unsubscribe();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy