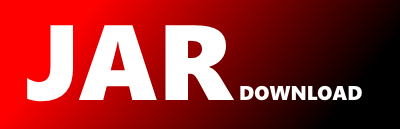
io.quarkus.redis.datasource.string.TransactionalStringCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.string;
import java.util.Map;
import io.quarkus.redis.datasource.TransactionalRedisCommands;
@Deprecated
public interface TransactionalStringCommands extends TransactionalRedisCommands {
/**
* Execute the command APPEND.
* Summary: Append a value to a key
* Group: string
* Requires Redis 2.0.0
*
* @param key the key
* @param value the value
*/
void append(K key, V value);
/**
* Execute the command DECR.
* Summary: Decrement the integer value of a key by one
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
*/
void decr(K key);
/**
* Execute the command DECRBY.
* Summary: Decrement the integer value of a key by the given number
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param amount the amount, can be negative
*/
void decrby(K key, long amount);
/**
* Execute the command GET.
* Summary: Get the value of a key
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
*/
void get(K key);
/**
* Execute the command GETDEL.
* Summary: Get the value of a key and delete the key
* Group: string
* Requires Redis 6.2.0
*
* @param key the key
*/
void getdel(K key);
/**
* Execute the command GETEX.
* Summary: Get the value of a key and optionally set its expiration
* Group: string
* Requires Redis 6.2.0
*
* @param key the key
* @param args the getex command extra-arguments
*/
void getex(K key, GetExArgs args);
/**
* Execute the command GETRANGE.
* Summary: Get a substring of the string stored at a key
* Group: string
* Requires Redis 2.4.0
*
* @param key the key
* @param start the start offset
* @param end the end offset
*/
void getrange(K key, long start, long end);
/**
* Execute the command GETSET.
* Summary: Set the string value of a key and return its old value
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @deprecated See https://redis.io/commands/getset
*/
void getset(K key, V value);
/**
* Execute the command INCR.
* Summary: Increment the integer value of a key by one
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
*/
void incr(K key);
/**
* Execute the command INCRBY.
* Summary: Increment the integer value of a key by the given amount
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param amount the amount, can be negative
*/
void incrby(K key, long amount);
/**
* Execute the command INCRBYFLOAT.
* Summary: Increment the float value of a key by the given amount
* Group: string
* Requires Redis 2.6.0
*
* @param key the key
* @param amount the amount, can be negative
*/
void incrbyfloat(K key, double amount);
/**
* Execute the command LCS.
* Summary: Find longest common substring
* Group: string
* Requires Redis 7.0.0
*
* @param key1 the key
* @param key2 the key
*/
void lcs(K key1, K key2);
/**
* Execute the command LCS.
* Summary: Find longest common substring and return the length (using {@code LEN})
* Group: string
* Requires Redis 7.0.0
*
* @param key1 the key
* @param key2 the key
*/
void lcsLength(K key1, K key2);
/**
* Execute the command MGET.
* Summary: Get the values of all the given keys
* Group: string
* Requires Redis 1.0.0
*
* @param keys the keys
*/
void mget(K... keys);
/**
* Execute the command MSET.
* Summary: Set multiple keys to multiple values
* Group: string
* Requires Redis 1.0.1
*
* @param map the key/value map containing the items to store
*/
void mset(Map map);
/**
* Execute the command MSETNX.
* Summary: Set multiple keys to multiple values, only if none of the keys exist
* Group: string
* Requires Redis 1.0.1
*
* @param map the key/value map containing the items to store
*/
void msetnx(Map map);
/**
* Execute the command PSETEX.
* Summary: Set the value and expiration in milliseconds of a key
* Group: string
* Requires Redis 2.6.0
*
* @param key the key
* @param milliseconds the duration in ms
* @param value the value
*/
void psetex(K key, long milliseconds, V value);
/**
* Execute the command SET.
* Summary: Set the string value of a key
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
*/
void set(K key, V value);
/**
* Execute the command SET.
* Summary: Set the string value of a key
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @param setArgs the set command extra-arguments
*/
void set(K key, V value, SetArgs setArgs);
/**
* Execute the command SET.
* Summary: Set the string value of a key, and return the previous value
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
*/
void setGet(K key, V value);
/**
* Execute the command SET.
* Summary: Set the string value of a key, and return the previous value
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @param setArgs the set command extra-arguments
*/
void setGet(K key, V value, SetArgs setArgs);
/**
* Execute the command SETEX.
* Summary: Set the value and expiration of a key
* Group: string
* Requires Redis 2.0.0
*
* @param key the key
* @param value the value
*/
void setex(K key, long seconds, V value);
/**
* Execute the command SETNX.
* Summary: Set the value of a key, only if the key does not exist
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
*/
void setnx(K key, V value);
/**
* Execute the command SETRANGE.
* Summary: Overwrite part of a string at key starting at the specified offset
* Group: string
* Requires Redis 2.2.0
*
* @param key the key
* @param value the value
*/
void setrange(K key, long offset, V value);
/**
* Execute the command STRLEN.
* Summary: Get the length of the value stored in a key
* Group: string
* Requires Redis 2.2.0
*
* @param key the key
*/
void strlen(K key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy