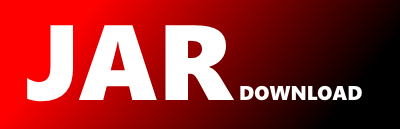
io.quarkus.redis.datasource.timeseries.CreateArgs Maven / Gradle / Ivy
Show all versions of quarkus-redis-client Show documentation
package io.quarkus.redis.datasource.timeseries;
import static io.smallrye.mutiny.helpers.ParameterValidation.nonNull;
import java.time.Duration;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import io.quarkus.redis.datasource.RedisCommandExtraArguments;
/**
* Represents the extra arguments of the {@code ts.create} command.
*/
public class CreateArgs implements RedisCommandExtraArguments {
private Duration retention;
private String enc;
private int chunkSize;
private DuplicatePolicy policy;
private final Map labels = new HashMap<>();
/**
* Set the maximum age for samples compared to the highest reported timestamp, in milliseconds.
* Samples are expired based solely on the difference between their timestamp and the timestamps passed to
* subsequent TS.ADD, TS.MADD, TS.INCRBY, and TS.DECRBY calls.
*
* When set to 0, samples never expire. When not specified, the option is set to the global RETENTION_POLICY
* configuration of the database, which by default is 0.
*
* @param retention the retention, must not be {@code null}
* @return the current {@code CreateArgs}
*/
public CreateArgs setRetention(Duration retention) {
this.retention = nonNull(retention, "retention");
return this;
}
/**
* Set the retention duration so the samples never expire.
*
* @return the current {@code CreateArgs}
* @see #setRetention(Duration)
*/
public CreateArgs forever() {
this.retention = Duration.ZERO;
return this;
}
/**
* Set the series samples encoding format to {@code COMPRESSED}, applies compression to the series samples.
* {@code COMPRESSED} is almost always the right choice. Compression not only saves memory but usually improves
* performance due to a lower number of memory accesses. It can result in about 90% memory reduction.
* The exception are highly irregular timestamps or values, which occur rarely.
*
* When not specified, the option is set to COMPRESSED.
*
* @return the current {@code CreateArgs}
*/
public CreateArgs compressed() {
this.enc = "COMPRESSED";
return this;
}
/**
* Set the series samples encoding format to {@code UNCOMPRESSED}, applies compression to the series samples.
*
* {@code COMPRESSED} is almost always the right choice. Compression not only saves memory but usually improves
* performance due to a lower number of memory accesses. It can result in about 90% memory reduction.
* The exception are highly irregular timestamps or values, which occur rarely.
*
* When not specified, the option is set to COMPRESSED.
*
* @return the current {@code CreateArgs}
*/
public CreateArgs uncompressed() {
this.enc = "UNCOMPRESSED";
return this;
}
/**
* Sets the initial allocation size, in bytes, for the data part of each new chunk.
* Actual chunks may consume more memory. Changing chunkSize (using TS.ALTER) does not affect existing chunks.
*
* Must be a multiple of 8 in the range [48 .. 1048576].
* When not specified, it is set to 4096 bytes (a single memory page).
*
* @param size the chunk size, between 48 and 1048576
* @return the current {@code CreateArgs}
*/
public CreateArgs chunkSize(int size) {
this.chunkSize = size;
return this;
}
/**
* Set the policy for handling insertion (TS.ADD and TS.MADD) of multiple samples with identical timestamps.
*
* When not specified: set to the global DUPLICATE_POLICY configuration of the database (which, by default, is BLOCK).
*
* @param policy the policy, must not be {@code null}
* @return the current {@code CreateArgs}
*/
public CreateArgs duplicatePolicy(DuplicatePolicy policy) {
this.policy = policy;
return this;
}
/**
* Set a label-value pairs that represent metadata labels of the key and serve as a secondary index.
*
* The TS.MGET, TS.MRANGE, and TS.MREVRANGE commands operate on multiple time series based on their labels.
* The TS.QUERYINDEX command returns all time series keys matching a given filter based on their labels.
*
* @param label the label, must not be {@code null}
* @param value the value, must not be {@code null}
* @return the current {@code CreateArgs}
*/
public CreateArgs label(String label, Object value) {
labels.put(label, value);
return this;
}
@Override
public List