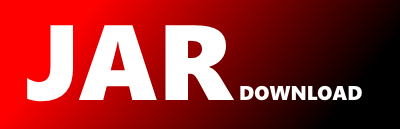
io.quarkus.redis.datasource.transactions.ReactiveTransactionalRedisDataSource Maven / Gradle / Ivy
Show all versions of quarkus-redis-client Show documentation
package io.quarkus.redis.datasource.transactions;
import io.quarkus.redis.datasource.autosuggest.ReactiveTransactionalAutoSuggestCommands;
import io.quarkus.redis.datasource.bitmap.ReactiveTransactionalBitMapCommands;
import io.quarkus.redis.datasource.bloom.ReactiveTransactionalBloomCommands;
import io.quarkus.redis.datasource.countmin.ReactiveTransactionalCountMinCommands;
import io.quarkus.redis.datasource.cuckoo.ReactiveTransactionalCuckooCommands;
import io.quarkus.redis.datasource.geo.ReactiveTransactionalGeoCommands;
import io.quarkus.redis.datasource.graph.ReactiveTransactionalGraphCommands;
import io.quarkus.redis.datasource.hash.ReactiveTransactionalHashCommands;
import io.quarkus.redis.datasource.hyperloglog.ReactiveTransactionalHyperLogLogCommands;
import io.quarkus.redis.datasource.json.ReactiveTransactionalJsonCommands;
import io.quarkus.redis.datasource.keys.ReactiveTransactionalKeyCommands;
import io.quarkus.redis.datasource.list.ReactiveTransactionalListCommands;
import io.quarkus.redis.datasource.search.ReactiveTransactionalSearchCommands;
import io.quarkus.redis.datasource.set.ReactiveTransactionalSetCommands;
import io.quarkus.redis.datasource.sortedset.ReactiveTransactionalSortedSetCommands;
import io.quarkus.redis.datasource.stream.ReactiveTransactionalStreamCommands;
import io.quarkus.redis.datasource.string.ReactiveTransactionalStringCommands;
import io.quarkus.redis.datasource.timeseries.ReactiveTransactionalTimeSeriesCommands;
import io.quarkus.redis.datasource.topk.ReactiveTransactionalTopKCommands;
import io.quarkus.redis.datasource.value.ReactiveTransactionalValueCommands;
import io.smallrye.common.annotation.Experimental;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Command;
/**
* Redis Data Source object used to execute commands in a Redis transaction ({@code MULTI}).
* Note that the results of the enqueued commands are not available until the completion of the transaction.
*/
public interface ReactiveTransactionalRedisDataSource {
/**
* Discard the current transaction.
*/
Uni discard();
/**
* Checks if the current transaction has been discarded by the user
*
* @return if the current transaction has been discarded by the user
*/
boolean discarded();
/**
* Gets the object to execute commands manipulating hashes (a.k.a. {@code Map<F, V>}).
*
* If you want to use a hash of {@code <String -> Person>} stored using String identifier, you would use:
* {@code hash(String.class, String.class, Person.class)}.
* If you want to use a hash of {@code <String -> Person>} stored using UUID identifier, you would use:
* {@code hash(UUID.class, String.class, Person.class)}.
*
* @param redisKeyType the class of the keys
* @param typeOfField the class of the fields
* @param typeOfValue the class of the values
* @param the type of the redis key
* @param the type of the fields (map's keys)
* @param the type of the value
* @return the object to execute commands manipulating hashes (a.k.a. {@code Map<K, V>}).
*/
ReactiveTransactionalHashCommands hash(Class redisKeyType, Class typeOfField,
Class typeOfValue);
/**
* Gets the object to execute commands manipulating hashes (a.k.a. {@code Map<String, V>}).
*
* This is a shortcut on {@code hash(String.class, String.class, V)}
*
* @param typeOfValue the class of the values
* @param the type of the value
* @return the object to execute commands manipulating hashes (a.k.a. {@code Map<String, V>}).
*/
default ReactiveTransactionalHashCommands hash(Class typeOfValue) {
return hash(String.class, String.class, typeOfValue);
}
/**
* Gets the object to execute commands manipulating geo items (a.k.a. {@code {longitude, latitude, member}}).
*
* {@code V} represents the type of the member, i.e. the localized thing.
*
* @param redisKeyType the class of the keys
* @param memberType the class of the members
* @param the type of the redis key
* @param the type of the member
* @return the object to execute geo commands.
*/
ReactiveTransactionalGeoCommands geo(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating geo items (a.k.a. {@code {longitude, latitude, member}}).
*
* {@code V} represents the type of the member, i.e. the localized thing.
*
* @param memberType the class of the members
* @param the type of the member
* @return the object to execute geo commands.
*/
default ReactiveTransactionalGeoCommands geo(Class memberType) {
return geo(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating keys and expiration times.
*
* @param redisKeyType the type of the keys
* @param the type of the key
* @return the object to execute commands manipulating keys.
*/
ReactiveTransactionalKeyCommands key(Class redisKeyType);
/**
* Gets the object to execute commands manipulating keys and expiration times.
*
* @return the object to execute commands manipulating keys.
*/
default ReactiveTransactionalKeyCommands key() {
return key(String.class);
}
/**
* Gets the object to execute commands manipulating sorted sets.
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value sorted in the sorted sets
* @param the type of the key
* @param the type of the value
* @return the object to manipulate sorted sets.
*/
ReactiveTransactionalSortedSetCommands sortedSet(Class redisKeyType, Class valueType);
/**
* Gets the object to execute commands manipulating sorted sets.
*
* @param valueType the type of the value sorted in the sorted sets
* @param the type of the value
* @return the object to manipulate sorted sets.
*/
default ReactiveTransactionalSortedSetCommands sortedSet(Class valueType) {
return sortedSet(String.class, valueType);
}
/**
* Gets the object to execute commands manipulating stored strings.
*
*
* NOTE: Instead of {@code string}, this group is named {@code value} to avoid the confusion with the
* Java String type. Indeed, Redis strings can be strings, numbers, byte arrays...
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the key
* @param the type of the value
* @return the object to manipulate stored strings.
*/
ReactiveTransactionalValueCommands value(Class redisKeyType, Class valueType);
/**
* Gets the object to execute commands manipulating stored strings.
*
*
* NOTE: Instead of {@code string}, this group is named {@code value} to avoid the confusion with the
* Java String type. Indeed, Redis strings can be strings, numbers, byte arrays...
*
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the value
* @return the object to manipulate stored strings.
*/
default ReactiveTransactionalValueCommands value(Class valueType) {
return value(String.class, valueType);
}
/**
* Gets the object to execute commands manipulating stored strings.
*
* @param redisKeyType the type of the keys
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the key
* @param the type of the value
* @return the object to manipulate stored strings.
* @deprecated Use {@link #value(Class, Class)} instead
*/
@Deprecated
ReactiveTransactionalStringCommands string(Class redisKeyType, Class valueType);
/**
* Gets the object to execute commands manipulating stored strings.
*
* @param valueType the type of the value, often String, or the value are encoded/decoded using codecs.
* @param the type of the value
* @return the object to manipulate stored strings.
* @deprecated Use {@link #value(Class)} instead
*/
@Deprecated
default ReactiveTransactionalStringCommands string(Class valueType) {
return string(String.class, valueType);
}
/**
* Gets the object to execute commands manipulating sets.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in each set
* @param the type of the key
* @param the type of the member
* @return the object to manipulate sets.
*/
ReactiveTransactionalSetCommands set(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating sets.
*
* @param memberType the type of the member stored in each set
* @param the type of the member
* @return the object to manipulate sets.
*/
default ReactiveTransactionalSetCommands set(Class memberType) {
return set(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating lists.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in each list
* @param the type of the key
* @param the type of the member
* @return the object to manipulate sets.
*/
ReactiveTransactionalListCommands list(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating lists.
*
* @param memberType the type of the member stored in each list
* @param the type of the member
* @return the object to manipulate sets.
*/
default ReactiveTransactionalListCommands list(Class memberType) {
return list(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating hyperloglog data structures.
*
* @param redisKeyType the type of the keys
* @param memberType the type of the member stored in the data structure
* @param the type of the key
* @param the type of the member
* @return the object to manipulate hyper log log data structures.
*/
ReactiveTransactionalHyperLogLogCommands hyperloglog(Class redisKeyType, Class memberType);
/**
* Gets the object to execute commands manipulating hyperloglog data structures.
*
* @param memberType the type of the member stored in the data structure
* @param the type of the member
* @return the object to manipulate hyper log log data structures.
*/
default ReactiveTransactionalHyperLogLogCommands hyperloglog(Class memberType) {
return hyperloglog(String.class, memberType);
}
/**
* Gets the object to execute commands manipulating bitmap data structures.
*
* @param redisKeyType the type of the keys
* @param the type of the key
* @return the object to manipulate bitmap data structures.
*/
ReactiveTransactionalBitMapCommands bitmap(Class redisKeyType);
/**
* Gets the object to execute commands manipulating bitmap data structures.
*
* @return the object to manipulate bitmap data structures.
*/
default ReactiveTransactionalBitMapCommands bitmap() {
return bitmap(String.class);
}
/**
* Gets the object to execute commands manipulating streams.
*
*
* @param redisKeyType the class of the keys
* @param typeOfField the class of the fields
* @param typeOfValue the class of the values
* @param the type of the redis key
* @param the type of the fields (map's keys)
* @param the type of the value
* @return the object to execute commands manipulating streams.
*/
ReactiveTransactionalStreamCommands stream(Class redisKeyType, Class typeOfField,
Class typeOfValue);
/**
* Gets the object to execute commands manipulating stream..
*
* This is a shortcut on {@code stream(String.class, String.class, V)}
*
* @param typeOfValue the class of the values
* @param the type of the value
* @return the object to execute commands manipulating streams.
*/
default ReactiveTransactionalStreamCommands stream(Class typeOfValue) {
return stream(String.class, String.class, typeOfValue);
}
/**
* Gets the object to manipulate JSON values.
* This group requires the RedisJSON module.
*
* @return the object to manipulate JSON values.
*/
default ReactiveTransactionalJsonCommands json() {
return json(String.class);
}
/**
* Gets the object to manipulate JSON values.
* This group requires the RedisJSON module.
*
* @param the type of keys
* @return the object to manipulate JSON values.
*/
ReactiveTransactionalJsonCommands json(Class redisKeyType);
/**
* Gets the object to manipulate Bloom filters.
* This group requires the RedisBloom module.
*
* @param valueType the type of value to store in the filters
* @param the type of value
* @return the object to manipulate Bloom filters
*/
default ReactiveTransactionalBloomCommands bloom(Class valueType) {
return bloom(String.class, valueType);
}
/**
* Gets the object to manipulate Bloom filters.
* This group requires the RedisBloom module.
*
* @param redisKeyType the type of the key
* @param valueType the type of value to store in the filters
* @param the type of key
* @param the type of value
* @return the object to manipulate Bloom filters
*/
ReactiveTransactionalBloomCommands bloom(Class redisKeyType, Class valueType);
/**
* Gets the object to manipulate Cuckoo filters.
* This group requires the RedisBloom module (including the Cuckoo
* filter support).
*
* @param the type of the values added into the Cuckoo filter
* @return the object to manipulate Cuckoo values.
*/
default ReactiveTransactionalCuckooCommands cuckoo(Class valueType) {
return cuckoo(String.class, valueType);
}
/**
* Gets the object to manipulate Cuckoo filters.
* This group requires the RedisBloom module (including the Cuckoo
* filter support).
*
* @param the type of keys
* @param the type of the values added into the Cuckoo filter
* @return the object to manipulate Cuckoo values.
*/
ReactiveTransactionalCuckooCommands cuckoo(Class redisKeyType, Class valueType);
/**
* Gets the object to manipulate Count-Min sketches.
* This group requires the RedisBloom module (including the count-min
* sketches support).
*
* @param the type of the values added into the count-min sketches
* @return the object to manipulate count-min sketches.
*/
default ReactiveTransactionalCountMinCommands countmin(Class valueType) {
return countmin(String.class, valueType);
}
/**
* Gets the object to manipulate Count-Min sketches.
* This group requires the RedisBloom module (including the count-min
* sketches support).
*
* @param the type of keys
* @param the type of the values added into the count-min sketches
* @return the object to manipulate count-min sketches.
*/
ReactiveTransactionalCountMinCommands countmin(Class redisKeyType, Class valueType);
/**
* Gets the object to manipulate Top-K list.
* This group requires the RedisBloom module (including the top-k
* list support).
*
* @param the type of the values added into the top-k lists
* @return the object to manipulate top-k lists.
*/
default ReactiveTransactionalTopKCommands topk(Class valueType) {
return topk(String.class, valueType);
}
/**
* Gets the object to manipulate Top-K list.
* This group requires the RedisBloom module (including the top-k
* list support).
*
* @param the type of keys
* @param the type of the values added into the top-k lists
* @return the object to manipulate top-k lists.
*/
ReactiveTransactionalTopKCommands topk(Class redisKeyType, Class valueType);
/**
* Gets the object to manipulate graphs.
* This group requires the RedisGraph module.
*
* @return the object to manipulate graphs lists.
*/
@Experimental("The Redis graph support is experimental")
default ReactiveTransactionalGraphCommands graph() {
return graph(String.class);
}
/**
* Gets the object to manipulate graphs.
* This group requires the RedisGraph module.
*
* @param the type of keys
* @return the object to manipulate graphs lists.
*/
@Experimental("The Redis graph support is experimental")
ReactiveTransactionalGraphCommands graph(Class redisKeyType);
/**
* Gets the object to emit commands from the {@code search} group.
* This group requires the RedisSearch module.
*
* @param the type of keys
* @return the object to search documents
*/
@Experimental("The Redis search support is experimental")
ReactiveTransactionalSearchCommands search(Class redisKeyType);
/**
* Gets the object to emit commands from the {@code search} group.
* This group requires the RedisSearch module.
*
* @return the object to search documents
*/
@Experimental("The Redis Search support is experimental")
default ReactiveTransactionalSearchCommands search() {
return search(String.class);
}
/**
* Gets the object to emit commands from the {@code auto-suggest} group.
* This group requires the RedisSearch module.
*
* @param the type of keys
* @return the object to get suggestions
*/
@Experimental("The Redis auto-suggest support is experimental")
ReactiveTransactionalAutoSuggestCommands autosuggest(Class redisKeyType);
/**
* Gets the object to emit commands from the {@code auto-suggest} group.
* This group requires the RedisSearch module.
*
* @return the object to get suggestions
*/
@Experimental("The Redis auto-suggest support is experimental")
default ReactiveTransactionalAutoSuggestCommands autosuggest() {
return autosuggest(String.class);
}
/**
* Gets the object to emit commands from the {@code time series} group.
* This group requires the Redis Time Series module.
*
* @param the type of keys
* @return the object to manipulate time series
*/
@Experimental("The Redis time series support is experimental")
ReactiveTransactionalTimeSeriesCommands timeseries(Class redisKeyType);
/**
* Gets the object to emit commands from the {@code time series} group.
* This group requires the Redis Time Series module.
*
* @return the object to manipulate time series
*/
@Experimental("The Redis time series support is experimental")
default ReactiveTransactionalTimeSeriesCommands timeseries() {
return timeseries(String.class);
}
/**
* Executes a command.
* This method is used to execute commands not offered by the API.
*
* @param command the command name
* @param args the parameters, encoded as String.
* @return the response
*/
Uni execute(String command, String... args);
/**
* Executes a command.
* This method is used to execute commands not offered by the API.
*
* @param command the command
* @param args the parameters, encoded as String.
* @return the response
*/
Uni execute(Command command, String... args);
/**
* Executes a command.
* This method is used to execute commands not offered by the API.
*
* @param command the command
* @param args the parameters, encoded as String.
* @return the response
*/
Uni execute(io.vertx.redis.client.Command command, String... args);
}