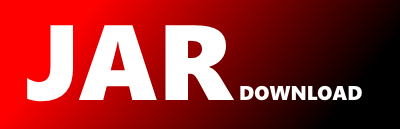
io.quarkus.redis.datasource.value.ReactiveTransactionalValueCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.datasource.value;
import java.util.Map;
import io.quarkus.redis.datasource.ReactiveTransactionalRedisCommands;
import io.smallrye.mutiny.Uni;
public interface ReactiveTransactionalValueCommands extends ReactiveTransactionalRedisCommands {
/**
* Execute the command APPEND.
* Summary: Append a value to a key
* Group: string
* Requires Redis 2.0.0
*
* @param key the key
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni append(K key, V value);
/**
* Execute the command DECR.
* Summary: Decrement the integer value of a key by one
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni decr(K key);
/**
* Execute the command DECRBY.
* Summary: Decrement the integer value of a key by the given number
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param amount the amount, can be negative
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni decrby(K key, long amount);
/**
* Execute the command GET.
* Summary: Get the value of a key
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni get(K key);
/**
* Execute the command GETDEL.
* Summary: Get the value of a key and delete the key
* Group: string
* Requires Redis 6.2.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni getdel(K key);
/**
* Execute the command GETEX.
* Summary: Get the value of a key and optionally set its expiration
* Group: string
* Requires Redis 6.2.0
*
* @param key the key
* @param args the getex command extra-arguments
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni getex(K key, GetExArgs args);
/**
* Execute the command GETRANGE.
* Summary: Get a substring of the string stored at a key
* Group: string
* Requires Redis 2.4.0
*
* @param key the key
* @param start the start offset
* @param end the end offset
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni getrange(K key, long start, long end);
/**
* Execute the command GETSET.
* Summary: Set the string value of a key and return its old value
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
* @deprecated See https://redis.io/commands/getset
*/
Uni getset(K key, V value);
/**
* Execute the command INCR.
* Summary: Increment the integer value of a key by one
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni incr(K key);
/**
* Execute the command INCRBY.
* Summary: Increment the integer value of a key by the given amount
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param amount the amount, can be negative
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni incrby(K key, long amount);
/**
* Execute the command INCRBYFLOAT.
* Summary: Increment the float value of a key by the given amount
* Group: string
* Requires Redis 2.6.0
*
* @param key the key
* @param amount the amount, can be negative
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni incrbyfloat(K key, double amount);
/**
* Execute the command LCS.
* Summary: Find longest common substring
* Group: string
* Requires Redis 7.0.0
*
* @param key1 the key
* @param key2 the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lcs(K key1, K key2);
/**
* Execute the command LCS.
* Summary: Find longest common substring and return the length (using {@code LEN})
* Group: string
* Requires Redis 7.0.0
*
* @param key1 the key
* @param key2 the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni lcsLength(K key1, K key2);
/**
* Execute the command MGET.
* Summary: Get the values of all the given keys
* Group: string
* Requires Redis 1.0.0
*
* @param keys the keys
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni mget(K... keys);
/**
* Execute the command MSET.
* Summary: Set multiple keys to multiple values
* Group: string
* Requires Redis 1.0.1
*
* @param map the key/value map containing the items to store
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni mset(Map map);
/**
* Execute the command MSETNX.
* Summary: Set multiple keys to multiple values, only if none of the keys exist
* Group: string
* Requires Redis 1.0.1
*
* @param map the key/value map containing the items to store
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni msetnx(Map map);
/**
* Execute the command PSETEX.
* Summary: Set the value and expiration in milliseconds of a key
* Group: string
* Requires Redis 2.6.0
*
* @param key the key
* @param milliseconds the duration in ms
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni psetex(K key, long milliseconds, V value);
/**
* Execute the command SET.
* Summary: Set the string value of a key
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni set(K key, V value);
/**
* Execute the command SET.
* Summary: Set the string value of a key
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @param setArgs the set command extra-arguments
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni set(K key, V value, SetArgs setArgs);
/**
* Execute the command SET.
* Summary: Set the string value of a key, and return the previous value
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni setGet(K key, V value);
/**
* Execute the command SET.
* Summary: Set the string value of a key, and return the previous value
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @param setArgs the set command extra-arguments
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni setGet(K key, V value, SetArgs setArgs);
/**
* Execute the command SETEX.
* Summary: Set the value and expiration of a key
* Group: string
* Requires Redis 2.0.0
*
* @param key the key
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
*/
Uni setex(K key, long seconds, V value);
/**
* Execute the command SETNX.
* Summary: Set the value of a key, only if the key does not exist
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni setnx(K key, V value);
/**
* Execute the command SETRANGE.
* Summary: Overwrite part of a string at key starting at the specified offset
* Group: string
* Requires Redis 2.2.0
*
* @param key the key
* @param value the value
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni setrange(K key, long offset, V value);
/**
* Execute the command STRLEN.
* Summary: Get the length of the value stored in a key
* Group: string
* Requires Redis 2.2.0
*
* @param key the key
* @return A {@code Uni} emitting {@code null} when the command has been enqueued successfully in the transaction, a failure
* otherwise. In the case of failure, the transaction is discarded.
*/
Uni strlen(K key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy