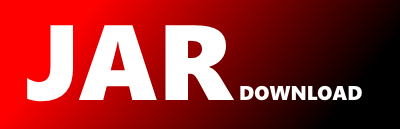
io.quarkus.redis.datasource.value.ReactiveValueCommands Maven / Gradle / Ivy
Show all versions of quarkus-redis-client Show documentation
package io.quarkus.redis.datasource.value;
import java.util.Map;
import io.quarkus.redis.datasource.ReactiveRedisCommands;
import io.smallrye.mutiny.Uni;
/**
* Allows executing commands from the {@code string} group.
* See the string command list for further information
* about these commands.
*
* This group can be used with value of type {@code String}, or a type which will be automatically
* serialized/deserialized with a codec.
*
* NOTE: Instead of {@code string}, this group is named {@code value} to avoid the confusion with the
* Java String type. Indeed, Redis strings can be strings, numbers, byte arrays...
*
* @param the type of the key
* @param the type of the value
*/
public interface ReactiveValueCommands extends ReactiveRedisCommands {
/**
* Execute the command APPEND.
* Summary: Append a value to a key
* Group: string
* Requires Redis 2.0.0
*
* @param key the key
* @param value the value
* @return the length of the string after the append operation.
**/
Uni append(K key, V value);
/**
* Execute the command DECR.
* Summary: Decrement the integer value of a key by one
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @return the value of key after the decrement
**/
Uni decr(K key);
/**
* Execute the command DECRBY.
* Summary: Decrement the integer value of a key by the given number
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param amount the amount, can be negative
* @return the value of key after the decrement
**/
Uni decrby(K key, long amount);
/**
* Execute the command GET.
* Summary: Get the value of a key
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @return the value of key, or {@code null} when key does not exist. If one of the passed key does not exist, the
* returned map contains a {@code null} value associated with the missing key.
**/
Uni get(K key);
/**
* Execute the command GETDEL.
* Summary: Get the value of a key and delete the key
* Group: string
* Requires Redis 6.2.0
*
* @param key the key
* @return the value of key, {@code null} when key does not exist, or an error if the key's value type isn't a string.
**/
Uni getdel(K key);
/**
* Execute the command GETEX.
* Summary: Get the value of a key and optionally set its expiration
* Group: string
* Requires Redis 6.2.0
*
* @param key the key
* @param args the getex command extra-arguments
* @return the value of key, or {@code null} when key does not exist.
**/
Uni getex(K key, GetExArgs args);
/**
* Execute the command GETRANGE.
* Summary: Get a substring of the string stored at a key
* Group: string
* Requires Redis 2.4.0
*
* @param key the key
* @param start the start offset
* @param end the end offset
* @return the sub-string
**/
Uni getrange(K key, long start, long end);
/**
* Execute the command GETSET.
* Summary: Set the string value of a key and return its old value
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param value the value
* @return the old value stored at key, or {@code null} when key did not exist.
* @deprecated See https://redis.io/commands/getset
**/
Uni getset(K key, V value);
/**
* Execute the command INCR.
* Summary: Increment the integer value of a key by one
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @return the value of key after the increment
**/
Uni incr(K key);
/**
* Execute the command INCRBY.
* Summary: Increment the integer value of a key by the given amount
* Group: string
* Requires Redis 1.0.0
*
* @param key the key
* @param amount the amount, can be negative
* @return the value of key after the increment
**/
Uni incrby(K key, long amount);
/**
* Execute the command INCRBYFLOAT.
* Summary: Increment the float value of a key by the given amount
* Group: string
* Requires Redis 2.6.0
*
* @param key the key
* @param amount the amount, can be negative
* @return the value of key after the increment.
**/
Uni incrbyfloat(K key, double amount);
/**
* Execute the command LCS.
* Summary: Find longest common substring
* Group: string
* Requires Redis 7.0.0
*
* @param key1 the key
* @param key2 the key
* @return the string representing the longest common substring is returned.
**/
Uni lcs(K key1, K key2);
/**
* Execute the command LCS.
* Summary: Find longest common substring and return the length (using {@code LEN})
* Group: string
* Requires Redis 7.0.0
*
* @param key1 the key
* @param key2 the key
* @return the length of the longest common substring.
**/
Uni lcsLength(K key1, K key2);
// TODO Add LCS with IDX support
/**
* Execute the command MGET.
* Summary: Get the values of all the given keys
* Group: string
* Requires Redis 1.0.0
*
* @param keys the keys
* @return list of values at the specified keys.
**/
Uni