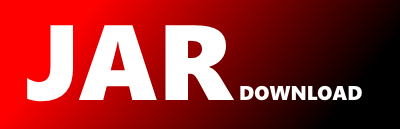
io.quarkus.redis.runtime.datasource.AbstractAutoSuggestCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import static io.quarkus.redis.runtime.datasource.Validation.notNullOrBlank;
import static io.smallrye.mutiny.helpers.ParameterValidation.nonNull;
import java.lang.reflect.Type;
import io.quarkus.redis.datasource.autosuggest.GetArgs;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Command;
import io.vertx.mutiny.redis.client.Response;
public class AbstractAutoSuggestCommands extends AbstractRedisCommands {
AbstractAutoSuggestCommands(RedisCommandExecutor redis, Type k) {
super(redis, new Marshaller(k));
}
Uni _ftSugAdd(K key, String string, double score, boolean increment) {
nonNull(key, "key");
notNullOrBlank(string, "string");
RedisCommand cmd = RedisCommand.of(Command.FT_SUGADD)
.put(marshaller.encode(key))
.put(string)
.put(score);
if (increment) {
cmd.put("INCR");
}
return execute(cmd);
}
Uni _ftSugDel(K key, String string) {
nonNull(key, "key");
notNullOrBlank(string, "string");
RedisCommand cmd = RedisCommand.of(Command.FT_SUGDEL)
.put(marshaller.encode(key))
.put(string);
return execute(cmd);
}
Uni _ftSugget(K key, String prefix) {
nonNull(key, "key");
notNullOrBlank(prefix, "prefix");
RedisCommand cmd = RedisCommand.of(Command.FT_SUGGET)
.put(marshaller.encode(key))
.put(prefix);
return execute(cmd);
}
Uni _ftSugget(K key, String prefix, GetArgs args) {
nonNull(key, "key");
notNullOrBlank(prefix, "prefix");
nonNull(args, "args");
RedisCommand cmd = RedisCommand.of(Command.FT_SUGGET)
.put(marshaller.encode(key))
.put(prefix)
.putArgs(args);
return execute(cmd);
}
Uni _ftSugLen(K key) {
nonNull(key, "key");
RedisCommand cmd = RedisCommand.of(Command.FT_SUGLEN)
.put(marshaller.encode(key));
return execute(cmd);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy