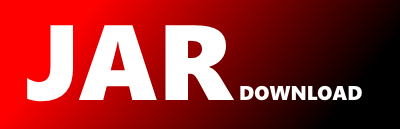
io.quarkus.redis.runtime.datasource.AbstractGraphCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import static io.smallrye.mutiny.helpers.ParameterValidation.nonNull;
import java.lang.reflect.Type;
import java.time.Duration;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Command;
import io.vertx.mutiny.redis.client.Response;
public class AbstractGraphCommands extends AbstractRedisCommands {
AbstractGraphCommands(RedisCommandExecutor redis, Type k) {
super(redis, new Marshaller(k));
}
Uni _graphDelete(K key) {
// Validation
nonNull(key, "key");
// Create command
RedisCommand cmd = RedisCommand.of(Command.GRAPH_DELETE)
.put(marshaller.encode(key));
return execute(cmd);
}
Uni _graphExplain(K key, String query) {
// Validation
nonNull(key, "key");
nonNull(query, "query");
// Create command
RedisCommand cmd = RedisCommand.of(Command.GRAPH_EXPLAIN)
.put(marshaller.encode(key))
.put(query);
return execute(cmd);
}
Uni _graphList() {
RedisCommand cmd = RedisCommand.of(Command.GRAPH_LIST);
return execute(cmd);
}
Uni _graphQuery(K key, String query) {
// Validation
nonNull(key, "key");
nonNull(query, "query");
// Create command
RedisCommand cmd = RedisCommand.of(Command.GRAPH_QUERY)
.put(marshaller.encode(key))
.put(query);
return execute(cmd);
}
Uni _graphQuery(K key, String query, Duration timeout) {
// Validation
nonNull(key, "key");
nonNull(query, "query");
nonNull(timeout, "timeout");
// Create command
RedisCommand cmd = RedisCommand.of(Command.GRAPH_QUERY)
.put(marshaller.encode(key))
.put(query)
.put("TIMEOUT").put(timeout.toMillis());
return execute(cmd);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy