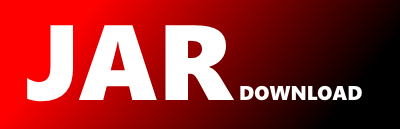
io.quarkus.redis.runtime.datasource.AbstractHashCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import static io.quarkus.redis.runtime.datasource.Validation.notNullOrEmpty;
import static io.quarkus.redis.runtime.datasource.Validation.positive;
import static io.smallrye.mutiny.helpers.ParameterValidation.doesNotContainNull;
import static io.smallrye.mutiny.helpers.ParameterValidation.nonNull;
import java.lang.reflect.Type;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Command;
import io.vertx.mutiny.redis.client.Response;
class AbstractHashCommands extends AbstractRedisCommands {
protected final Type typeOfValue;
protected final Type typeOfField;
AbstractHashCommands(RedisCommandExecutor redis, Type k, Type f, Type v) {
super(redis, new Marshaller(k, f, v));
this.typeOfField = f;
this.typeOfValue = v;
}
Uni _hdel(K key, F[] fields) {
nonNull(key, "key");
notNullOrEmpty(fields, "fields");
doesNotContainNull(fields, "fields");
RedisCommand cmd = RedisCommand.of(Command.HDEL)
.put(marshaller.encode(key));
for (F field : fields) {
cmd.put(marshaller.encode(field));
}
return execute(cmd);
}
Uni _hexists(K key, F field) {
nonNull(key, "key");
nonNull(field, "field");
return execute(RedisCommand.of(Command.HEXISTS).put(marshaller.encode(key)).put(marshaller.encode(field)));
}
Uni _hget(K key, F field) {
nonNull(key, "key");
nonNull(field, "field");
return execute(RedisCommand.of(Command.HGET).put(marshaller.encode(key)).put(marshaller.encode(field)));
}
Uni _hincrby(K key, F field, long amount) {
nonNull(key, "key");
nonNull(field, "field");
return execute(RedisCommand.of(Command.HINCRBY).put(marshaller.encode(key))
.put(marshaller.encode(field)).put(amount));
}
Uni _hincrbyfloat(K key, F field, double amount) {
nonNull(key, "key");
nonNull(field, "field");
return execute(RedisCommand.of(Command.HINCRBYFLOAT).put(marshaller.encode(key))
.put(marshaller.encode(field)).put(amount));
}
Uni _hgetall(K key) {
nonNull(key, "key");
return execute((RedisCommand.of(Command.HGETALL).put(marshaller.encode(key))));
}
Uni _hkeys(K key) {
nonNull(key, "key");
return execute((RedisCommand.of(Command.HKEYS).put(marshaller.encode(key))));
}
Uni _hlen(K key) {
nonNull(key, "key");
return execute((RedisCommand.of(Command.HLEN).put(marshaller.encode(key))));
}
@SafeVarargs
final Uni _hmget(K key, F... fields) {
RedisCommand cmd = RedisCommand.of(Command.HMGET);
cmd.put(marshaller.encode(key));
for (F field : fields) {
cmd.put(marshaller.encode(field));
}
return execute(cmd);
}
Uni _hmset(K key, Map map) {
nonNull(key, "key");
nonNull(map, "map");
if (map.isEmpty()) {
throw new IllegalArgumentException("`map` must not be empty");
}
RedisCommand cmd = RedisCommand.of(Command.HMSET);
cmd.put(marshaller.encode(key));
for (Map.Entry entry : map.entrySet()) {
cmd.put(marshaller.encode(entry.getKey()));
cmd.putNullable(marshaller.encode(entry.getValue()));
}
return execute(cmd);
}
Uni _hrandfield(K key) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.HRANDFIELD).put(marshaller.encode(key)));
}
Uni _hrandfield(K key, long count) {
nonNull(key, "key");
positive(count, "count");
return execute(RedisCommand.of(Command.HRANDFIELD).put(marshaller.encode(key)).put(count));
}
Uni _hrandfieldWithValues(K key, long count) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.HRANDFIELD).put(marshaller.encode(key)).put(count).put("WITHVALUES"));
}
Uni _hset(K key, F field, V value) {
nonNull(key, "key");
nonNull(field, "field");
nonNull(value, "value");
return execute(RedisCommand.of(Command.HSET)
.put(marshaller.encode(key))
.put(marshaller.encode(field))
.put(marshaller.encode(value)));
}
Uni _hset(K key, Map map) {
nonNull(key, "key");
nonNull(map, "map");
if (map.isEmpty()) {
throw new IllegalArgumentException("`map` must not be empty");
}
RedisCommand cmd = RedisCommand.of(Command.HSET);
cmd.put(marshaller.encode(key));
for (Map.Entry entry : map.entrySet()) {
cmd
.put(marshaller.encode(entry.getKey()))
.put(marshaller.encode(entry.getValue()));
}
return execute(cmd);
}
Uni _hsetnx(K key, F field, V value) {
nonNull(key, "key");
nonNull(field, "field");
return execute(RedisCommand.of(Command.HSETNX)
.put(marshaller.encode(key))
.put(marshaller.encode(field))
.put(marshaller.encode(value)));
}
Uni _hstrlen(K key, F field) {
nonNull(key, "key");
nonNull(field, "field");
return execute(RedisCommand.of(Command.HSTRLEN).put(marshaller.encode(key))
.put(marshaller.encode(field)));
}
Uni _hvals(K key) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.HVALS).put(marshaller.encode(key)));
}
V decodeV(Response resp) {
return marshaller.decode(typeOfValue, resp);
}
Map decodeMap(Response r) {
return marshaller.decodeAsMap(r, typeOfField, typeOfValue);
}
List decodeListOfField(Response r) {
return marshaller.decodeAsList(r, typeOfField);
}
List decodeListOfValue(Response r) {
return marshaller.decodeAsList(r, typeOfValue);
}
F decodeF(Response resp) {
return marshaller.decode(typeOfField, resp);
}
Map decodeOrderedMap(Response r, F[] fields) {
return marshaller.decodeAsOrderedMap(r, typeOfValue, fields);
}
Map decodeFieldWithValueMap(Response r) {
if (r == null) {
return Collections.emptyMap();
}
Map map = new HashMap<>();
for (Response nested : r) {
map.put(marshaller.decode(typeOfField, nested.get(0)),
marshaller.decode(typeOfValue, nested.get(1)));
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy