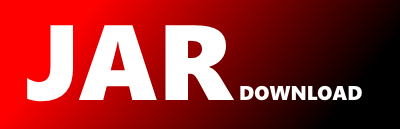
io.quarkus.redis.runtime.datasource.AbstractListCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import static io.quarkus.redis.runtime.datasource.Validation.notNullOrEmpty;
import static io.smallrye.mutiny.helpers.ParameterValidation.doesNotContainNull;
import static io.smallrye.mutiny.helpers.ParameterValidation.nonNull;
import static io.smallrye.mutiny.helpers.ParameterValidation.positive;
import static io.smallrye.mutiny.helpers.ParameterValidation.validate;
import java.lang.reflect.Type;
import java.time.Duration;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import io.quarkus.redis.datasource.list.KeyValue;
import io.quarkus.redis.datasource.list.LPosArgs;
import io.quarkus.redis.datasource.list.Position;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Command;
import io.vertx.mutiny.redis.client.Response;
class AbstractListCommands extends ReactiveSortable {
protected final Type typeOfValue;
protected final Type typeOfKey;
protected static final LPosArgs DEFAULT_INSTANCE = new LPosArgs();
AbstractListCommands(RedisCommandExecutor redis, Type k, Type v) {
super(redis, new Marshaller(k, v), v);
this.typeOfKey = k;
this.typeOfValue = v;
}
Uni _blmove(K source, K destination, Position positionInSource, Position positionInDest, Duration timeout) {
nonNull(source, "source");
nonNull(destination, "destination");
nonNull(positionInSource, "positionInSource");
nonNull(positionInDest, "positionInDest");
validate(timeout, "timeout");
return execute(RedisCommand.of(Command.BLMOVE).put(marshaller.encode(source))
.put(marshaller.encode(destination))
.put(positionInSource.name())
.put(positionInDest.name())
.put(timeout.toSeconds()));
}
V decodeV(Response r) {
return marshaller.decode(typeOfValue, r);
}
Uni _blmpop(Duration timeout, Position position, K... keys) {
nonNull(position, "position");
notNullOrEmpty(keys, "keys");
doesNotContainNull(keys, "keys");
validate(timeout, "timeout");
RedisCommand cmd = RedisCommand.of(Command.BLMPOP);
cmd.put(timeout.toSeconds());
cmd.put(keys.length);
cmd.putAll(marshaller.encode(keys));
cmd.put(position.name());
return execute(cmd);
}
KeyValue decodeKeyValue(Response r) {
if (r != null && r.getDelegate() != null) {
Response r1 = r.get(0);
Response r2 = r.get(1);
K key = marshaller.decode(typeOfKey, r1);
V val = marshaller.decode(typeOfValue, r2);
return new KeyValue<>(key, val);
}
return null;
}
KeyValue decodeKeyValueWithList(Response r) {
if (r != null && r.getDelegate() != null) {
Response r1 = r.get(0);
Response r2 = r.get(1).get(0);
K key = marshaller.decode(typeOfKey, r1);
V val = marshaller.decode(typeOfValue, r2);
return new KeyValue<>(key, val);
}
return null;
}
Uni _blmpop(Duration timeout, Position position, int count, K... keys) {
nonNull(position, "position");
notNullOrEmpty(keys, "keys");
doesNotContainNull(keys, "keys");
validate(timeout, "timeout");
positive(count, "count");
RedisCommand cmd = RedisCommand.of(Command.BLMPOP);
cmd.put(timeout.toSeconds());
cmd.put(keys.length);
cmd.putAll(marshaller.encode(keys));
cmd.put(position.name());
cmd.put("COUNT").put(count);
return execute(cmd);
}
List> decodeListOfKeyValue(Response r) {
if (r == null || r.getDelegate() == null) {
return Collections.emptyList();
}
List> res = new ArrayList<>();
K key = marshaller.decode(typeOfKey, r.get(0).toBytes());
for (Response item : r.get(1)) {
if (item == null) {
res.add(KeyValue.of(key, null));
} else {
res.add(KeyValue.of(key, marshaller.decode(typeOfValue, item)));
}
}
return res;
}
Uni _blpop(Duration timeout, K... keys) {
notNullOrEmpty(keys, "keys");
doesNotContainNull(keys, "keys");
validate(timeout, "timeout");
RedisCommand cmd = RedisCommand.of(Command.BLPOP);
cmd.put(timeout.toSeconds());
cmd.putAll(marshaller.encode(keys));
cmd.put(timeout.toSeconds());
return execute(cmd);
}
Uni _brpop(Duration timeout, K... keys) {
notNullOrEmpty(keys, "keys");
doesNotContainNull(keys, "keys");
validate(timeout, "timeout");
RedisCommand cmd = RedisCommand.of(Command.BRPOP);
cmd.put(timeout.toSeconds());
cmd.putAll(marshaller.encode(keys));
cmd.put(timeout.toSeconds());
return execute(cmd);
}
Uni _brpoplpush(Duration timeout, K source, K destination) {
validate(timeout, "timeout");
nonNull(source, "source");
nonNull(destination, "destination");
return execute(RedisCommand.of(Command.BRPOPLPUSH).put(marshaller.encode(source))
.put(marshaller.encode(destination)).put(timeout.toSeconds()));
}
Uni _lindex(K key, long index) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.LINDEX).put(marshaller.encode(key)).put(index));
}
Uni _linsertBeforePivot(K key, V pivot, V element) {
nonNull(key, "key");
nonNull(pivot, "pivot");
nonNull(element, "element");
return execute(RedisCommand.of(Command.LINSERT).put(marshaller.encode(key)).put("BEFORE")
.put(marshaller.encode(pivot)).put(marshaller.encode(element)));
}
Uni _linsertAfterPivot(K key, V pivot, V element) {
nonNull(key, "key");
nonNull(pivot, "pivot");
nonNull(element, "element");
return execute(RedisCommand.of(Command.LINSERT).put(marshaller.encode(key)).put("AFTER")
.put(marshaller.encode(pivot)).put(marshaller.encode(element)));
}
Uni _llen(K key) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.LLEN).put(marshaller.encode(key)));
}
Uni _lmove(K source, K destination, Position positionInSource, Position positionInDest) {
nonNull(source, "source");
nonNull(destination, "destination");
nonNull(positionInSource, "positionInSource");
nonNull(positionInDest, "positionInDest");
return execute(RedisCommand.of(Command.LMOVE)
.put(marshaller.encode(source))
.put(marshaller.encode(destination))
.put(marshaller.encode(positionInSource.name()))
.put(marshaller.encode(positionInDest.name())));
}
Uni _lmpop(Position position, K... keys) {
nonNull(position, "position");
notNullOrEmpty(keys, "keys");
doesNotContainNull(keys, "keys");
RedisCommand cmd = RedisCommand.of(Command.LMPOP);
cmd.put(keys.length);
cmd.putAll(marshaller.encode(keys));
cmd.put(position.name());
return execute(cmd);
}
Uni _lmpop(Position position, int count, K... keys) {
nonNull(position, "position");
notNullOrEmpty(keys, "keys");
doesNotContainNull(keys, "keys");
positive(count, "count");
RedisCommand cmd = RedisCommand.of(Command.LMPOP);
cmd.put(keys.length);
cmd.putAll(marshaller.encode(keys));
cmd.put(position.name());
cmd.put("COUNT").put(count);
return execute(cmd);
}
Uni _lpop(K key) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.LPOP).put(marshaller.encode(key)));
}
Uni _lpop(K key, int count) {
nonNull(key, "key");
positive(count, "count");
return execute(RedisCommand.of(Command.LPOP).put(marshaller.encode(key)).put(count));
}
List decodeListV(Response r) {
return marshaller.decodeAsList(r, typeOfValue);
}
Uni _lpos(K key, V element) {
return _lpos(key, element, DEFAULT_INSTANCE);
}
Uni _lpos(K key, V element, LPosArgs args) {
nonNull(key, "key");
nonNull(element, "element");
RedisCommand cmd = RedisCommand.of(Command.LPOS);
cmd.put(marshaller.encode(key));
cmd.put(marshaller.encode(element));
cmd.putArgs(args);
return execute(cmd);
}
Long decodeLongOrNull(Response r) {
if (r == null) {
return null;
}
return r.toLong();
}
Uni _lpos(K key, V element, int count) {
return _lpos(key, element, count, DEFAULT_INSTANCE);
}
Uni _lpos(K key, V element, int count, LPosArgs args) {
nonNull(key, "key");
nonNull(element, "element");
Validation.positiveOrZero(count, "count"); // 0 -> All matches
RedisCommand cmd = RedisCommand.of(Command.LPOS);
cmd.put(marshaller.encode(key));
cmd.put(marshaller.encode(element));
cmd.put("COUNT").put(count);
cmd.putArgs(args);
return execute(cmd);
}
List decodeListOfLongs(Response r) {
return marshaller.decodeAsList(r, Response::toLong);
}
Uni _lpush(K key, V... elements) {
nonNull(key, "key");
notNullOrEmpty(elements, "elements");
doesNotContainNull(elements, "elements");
RedisCommand cmd = RedisCommand.of(Command.LPUSH);
cmd.put(marshaller.encode(key)).putAll(marshaller.encode(elements));
return execute(cmd);
}
Uni _lpushx(K key, V... elements) {
nonNull(key, "key");
notNullOrEmpty(elements, "elements");
doesNotContainNull(elements, "elements");
RedisCommand cmd = RedisCommand.of(Command.LPUSHX);
cmd.put(marshaller.encode(key)).putAll(marshaller.encode(elements));
return execute(cmd);
}
Uni _lrange(K key, long start, long stop) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.LRANGE).put(marshaller.encode(key))
.put(start)
.put(stop));
}
Uni _lrem(K key, long count, V element) {
nonNull(key, "key");
nonNull(element, "element");
return execute(RedisCommand.of(Command.LREM).put(marshaller.encode(key))
.put(count).put(marshaller.encode(element)));
}
Uni _lset(K key, long index, V element) {
nonNull(key, "key");
nonNull(element, "element");
return execute(RedisCommand.of(Command.LSET).put(marshaller.encode(key))
.put(index).put(marshaller.encode(element)));
}
Uni _ltrim(K key, long start, long stop) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.LTRIM).put(marshaller.encode(key))
.put(start).put(stop));
}
Uni _rpop(K key) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.RPOP).put(marshaller.encode(key)));
}
Uni _rpop(K key, int count) {
nonNull(key, "key");
return execute(RedisCommand.of(Command.RPOP).put(marshaller.encode(key)).put(count));
}
Uni _rpoplpush(K source, K destination) {
nonNull(source, "source");
nonNull(destination, "destination");
return execute(RedisCommand.of(Command.RPOPLPUSH)
.put(marshaller.encode(source)).put(marshaller.encode(destination)));
}
Uni _rpush(K key, V... values) {
nonNull(key, "key");
notNullOrEmpty(values, "values");
doesNotContainNull(values, "values");
RedisCommand cmd = RedisCommand.of(Command.RPUSH);
cmd.put(marshaller.encode(key)).putAll(marshaller.encode(values));
return execute(cmd);
}
Uni _rpushx(K key, V... values) {
nonNull(key, "key");
notNullOrEmpty(values, "values");
doesNotContainNull(values, "values");
RedisCommand cmd = RedisCommand.of(Command.RPUSHX);
cmd.put(marshaller.encode(key)).putAll(marshaller.encode(values));
return execute(cmd);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy