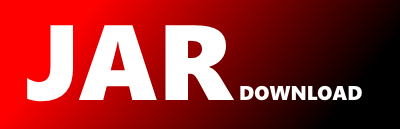
io.quarkus.redis.runtime.datasource.AbstractTopKCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import static io.smallrye.mutiny.helpers.ParameterValidation.doesNotContainNull;
import static io.smallrye.mutiny.helpers.ParameterValidation.isNotEmpty;
import static io.smallrye.mutiny.helpers.ParameterValidation.nonNull;
import static io.smallrye.mutiny.helpers.ParameterValidation.positive;
import java.lang.reflect.Type;
import java.util.Map;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Command;
import io.vertx.mutiny.redis.client.Response;
public class AbstractTopKCommands extends AbstractRedisCommands {
AbstractTopKCommands(RedisCommandExecutor redis, Type k, Type v) {
super(redis, new Marshaller(k, v));
}
Uni _topkAdd(K key, V item) {
// Validation
nonNull(key, "key");
nonNull(item, "item");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_ADD)
.put(marshaller.encode(key))
.put(marshaller.encode(item));
return execute(cmd);
}
Uni _topkAdd(K key, V... items) {
// Validation
nonNull(key, "key");
doesNotContainNull(items, "items");
if (items.length == 0) {
throw new IllegalArgumentException("`items` must not be empty");
}
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_ADD)
.put(marshaller.encode(key))
.putAll(marshaller.encode(items));
return execute(cmd);
}
Uni _topkIncrBy(K key, V item, int increment) {
// Validation
nonNull(key, "key");
nonNull(item, "item");
positive(increment, "increment");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_INCRBY)
.put(marshaller.encode(key))
.put(marshaller.encode(item))
.put(increment);
return execute(cmd);
}
Uni _topkIncrBy(K key, Map couples) {
// Validation
nonNull(key, "key");
nonNull(couples, "couples");
isNotEmpty(couples.keySet(), "couples");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_INCRBY)
.put(marshaller.encode(key));
for (Map.Entry entry : couples.entrySet()) {
cmd.put(marshaller.encode(entry.getKey()));
cmd.put(entry.getValue());
}
return execute(cmd);
}
Uni _topkList(K key) {
// Validation
nonNull(key, "key");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_LIST)
.put(marshaller.encode(key));
return execute(cmd);
}
Uni _topkListWithCount(K key) {
// Validation
nonNull(key, "key");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_LIST)
.put(marshaller.encode(key))
.put("WITHCOUNT");
return execute(cmd);
}
Uni _topkQuery(K key, V item) {
// Validation
nonNull(key, "key");
nonNull(item, "item");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_QUERY)
.put(marshaller.encode(key))
.put(marshaller.encode(item));
return execute(cmd);
}
Uni _topkQuery(K key, V... items) {
// Validation
nonNull(key, "key");
doesNotContainNull(items, "items");
if (items.length == 0) {
throw new IllegalArgumentException("`items` must not be empty");
}
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_QUERY)
.put(marshaller.encode(key))
.putAll(marshaller.encode(items));
return execute(cmd);
}
Uni _topkReserve(K key, int topk) {
// Validation
nonNull(key, "key");
positive(topk, "topk");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_RESERVE)
.put(marshaller.encode(key))
.put(topk);
return execute(cmd);
}
Uni _topkReserve(K key, int topk, int width, int depth, double decay) {
// Validation
nonNull(key, "key");
positive(topk, "topk");
// Create command
RedisCommand cmd = RedisCommand.of(Command.TOPK_RESERVE)
.put(marshaller.encode(key))
.put(topk)
.put(width)
.put(depth)
.put(decay);
return execute(cmd);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy