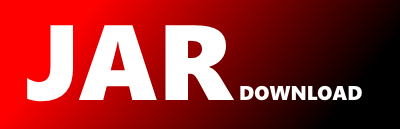
io.quarkus.redis.runtime.datasource.BlockingHashCommandsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import java.time.Duration;
import java.util.List;
import java.util.Map;
import io.quarkus.redis.datasource.RedisDataSource;
import io.quarkus.redis.datasource.ScanArgs;
import io.quarkus.redis.datasource.hash.HashCommands;
import io.quarkus.redis.datasource.hash.HashScanCursor;
import io.quarkus.redis.datasource.hash.ReactiveHashCommands;
public class BlockingHashCommandsImpl extends AbstractRedisCommandGroup implements HashCommands {
private final ReactiveHashCommands reactive;
public BlockingHashCommandsImpl(RedisDataSource ds, ReactiveHashCommands reactive, Duration timeout) {
super(ds, timeout);
this.reactive = reactive;
}
@Override
public int hdel(K key, F... fields) {
return reactive.hdel(key, fields)
.await().atMost(timeout);
}
@Override
public boolean hexists(K key, F field) {
return reactive.hexists(key, field)
.await().atMost(timeout);
}
@Override
public V hget(K key, F field) {
return reactive.hget(key, field)
.await().atMost(timeout);
}
@Override
public long hincrby(K key, F field, long amount) {
return reactive.hincrby(key, field, amount)
.await().atMost(timeout);
}
@Override
public double hincrbyfloat(K key, F field, double amount) {
return reactive.hincrbyfloat(key, field, amount)
.await().atMost(timeout);
}
@Override
public Map hgetall(K key) {
return reactive.hgetall(key)
.await().atMost(timeout);
}
@Override
public List hkeys(K key) {
return reactive.hkeys(key).await().atMost(timeout);
}
@Override
public long hlen(K key) {
return reactive.hlen(key)
.await().atMost(timeout);
}
@Override
public Map hmget(K key, F... fields) {
return reactive.hmget(key, fields)
.await().atMost(timeout);
}
@Override
public void hmset(K key, Map map) {
reactive.hmset(key, map)
.await().atMost(timeout);
}
@Override
public F hrandfield(K key) {
return reactive.hrandfield(key)
.await().atMost(timeout);
}
@Override
public List hrandfield(K key, long count) {
return reactive.hrandfield(key, count)
.await().atMost(timeout);
}
@Override
public Map hrandfieldWithValues(K key, long count) {
return reactive.hrandfieldWithValues(key, count)
.await().atMost(timeout);
}
@Override
public HashScanCursor hscan(K key) {
return new HashScanBlockingCursorImpl<>(reactive.hscan(key), timeout);
}
@Override
public HashScanCursor hscan(K key, ScanArgs scanArgs) {
return new HashScanBlockingCursorImpl<>(reactive.hscan(key, scanArgs), timeout);
}
@Override
public boolean hset(K key, F field, V value) {
return reactive.hset(key, field, value)
.await().atMost(timeout);
}
@Override
public long hset(K key, Map map) {
return reactive.hset(key, map)
.await().atMost(timeout);
}
@Override
public boolean hsetnx(K key, F field, V value) {
return reactive.hsetnx(key, field, value)
.await().atMost(timeout);
}
@Override
public long hstrlen(K key, F field) {
return reactive.hstrlen(key, field)
.await().atMost(timeout);
}
@Override
public List hvals(K key) {
return reactive.hvals(key)
.await().atMost(timeout);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy