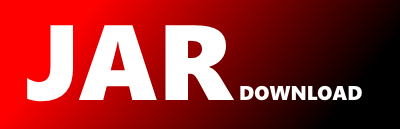
io.quarkus.redis.runtime.datasource.BlockingTopKCommandsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import java.time.Duration;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import io.quarkus.redis.datasource.RedisDataSource;
import io.quarkus.redis.datasource.topk.ReactiveTopKCommands;
import io.quarkus.redis.datasource.topk.TopKCommands;
public class BlockingTopKCommandsImpl extends AbstractRedisCommandGroup implements TopKCommands {
private final ReactiveTopKCommands reactive;
public BlockingTopKCommandsImpl(RedisDataSource ds, ReactiveTopKCommands reactive, Duration timeout) {
super(ds, timeout);
this.reactive = reactive;
}
@Override
public Optional topkAdd(K key, V item) {
return Optional.ofNullable(reactive.topkAdd(key, item).await().atMost(timeout));
}
@Override
public List topkAdd(K key, V... items) {
return reactive.topkAdd(key, items).await().atMost(timeout);
}
@Override
public Optional topkIncrBy(K key, V item, int increment) {
return Optional.ofNullable(reactive.topkIncrBy(key, item, increment).await().atMost(timeout));
}
@Override
public Map topkIncrBy(K key, Map couples) {
return reactive.topkIncrBy(key, couples).await().atMost(timeout);
}
@Override
public List topkList(K key) {
return reactive.topkList(key).await().atMost(timeout);
}
@Override
public Map topkListWithCount(K key) {
return reactive.topkListWithCount(key).await().atMost(timeout);
}
@Override
public boolean topkQuery(K key, V item) {
return reactive.topkQuery(key, item).await().atMost(timeout);
}
@Override
public List topkQuery(K key, V... items) {
return reactive.topkQuery(key, items).await().atMost(timeout);
}
@Override
public void topkReserve(K key, int topk) {
reactive.topkReserve(key, topk).await().atMost(timeout);
}
@Override
public void topkReserve(K key, int topk, int width, int depth, double decay) {
reactive.topkReserve(key, topk, width, depth, decay).await().atMost(timeout);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy