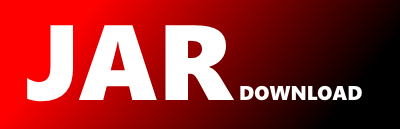
io.quarkus.redis.runtime.datasource.ReactiveBitMapCommandsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import java.lang.reflect.Type;
import java.util.List;
import io.quarkus.redis.datasource.ReactiveRedisCommands;
import io.quarkus.redis.datasource.ReactiveRedisDataSource;
import io.quarkus.redis.datasource.bitmap.BitFieldArgs;
import io.quarkus.redis.datasource.bitmap.ReactiveBitMapCommands;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Response;
public class ReactiveBitMapCommandsImpl extends AbstractBitMapCommands
implements ReactiveBitMapCommands, ReactiveRedisCommands {
private final ReactiveRedisDataSource reactive;
public ReactiveBitMapCommandsImpl(ReactiveRedisDataSourceImpl redis, Type k) {
super(redis, k);
this.reactive = redis;
}
@Override
public ReactiveRedisDataSource getDataSource() {
return reactive;
}
@Override
public Uni bitcount(K key) {
return super._bitcount(key)
.map(Response::toLong);
}
@Override
public Uni bitcount(K key, long start, long end) {
return super._bitcount(key, start, end)
.map(Response::toLong);
}
@Override
public Uni> bitfield(K key, BitFieldArgs bitFieldArgs) {
return super._bitfield(key, bitFieldArgs)
.map(this::decodeListOfLongs);
}
@Override
public Uni bitpos(K key, int bit) {
return super._bitpos(key, bit)
.map(Response::toLong);
}
@Override
public Uni bitpos(K key, int bit, long start) {
return super._bitpos(key, bit, start)
.map(Response::toLong);
}
@Override
public Uni bitpos(K key, int bit, long start, long end) {
return super._bitpos(key, bit, start, end)
.map(Response::toLong);
}
@SafeVarargs
@Override
public final Uni bitopAnd(K destination, K... keys) {
return super._bitopAnd(destination, keys)
.map(Response::toLong);
}
@Override
public Uni bitopNot(K destination, K source) {
return super._bitopNot(destination, source)
.map(Response::toLong);
}
@SafeVarargs
@Override
public final Uni bitopOr(K destination, K... keys) {
return super._bitopOr(destination, keys)
.map(Response::toLong);
}
@SafeVarargs
@Override
public final Uni bitopXor(K destination, K... keys) {
return super._bitopXor(destination, keys)
.map(Response::toLong);
}
@Override
public Uni setbit(K key, long offset, int value) {
return super._setbit(key, offset, value)
.map(Response::toInteger);
}
@Override
public Uni getbit(K key, long offset) {
return super._getbit(key, offset)
.map(Response::toInteger);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy