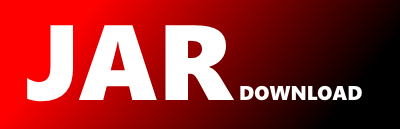
io.quarkus.redis.runtime.datasource.ScanReactiveCursorImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-redis-client Show documentation
Show all versions of quarkus-redis-client Show documentation
Connect to Redis in either imperative or reactive style
package io.quarkus.redis.runtime.datasource;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import io.quarkus.redis.datasource.ReactiveCursor;
import io.quarkus.redis.datasource.keys.ReactiveKeyScanCursor;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.vertx.mutiny.redis.client.Command;
public class ScanReactiveCursorImpl extends AbstractRedisCommands implements ReactiveKeyScanCursor {
private final Type typeOfKey;
private long cursor;
private final List extra = new ArrayList<>();
public ScanReactiveCursorImpl(RedisCommandExecutor redis, Marshaller marshaller, Type typeOfKey, List extra) {
super(redis, marshaller);
this.cursor = ReactiveCursor.INITIAL_CURSOR_ID;
this.typeOfKey = typeOfKey;
this.extra.addAll(extra);
}
@Override
public long cursorId() {
return cursor;
}
@Override
public boolean hasNext() {
return cursor != 0;
}
@Override
public Uni> next() {
long pos = cursor == INITIAL_CURSOR_ID ? 0 : cursor;
return execute(RedisCommand.of(Command.SCAN).put(pos).putAll(extra))
.invoke(response -> cursor = response.get(0).toLong())
.map(response -> marshaller.decodeAsSet(response.get(1), typeOfKey));
}
@Override
public Multi toMulti() {
return Multi.createBy().repeating()
.uni(this::next)
.whilst(m -> !m.isEmpty() && hasNext())
.onItem().transformToMultiAndConcatenate(set -> Multi.createFrom().items(set.stream()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy