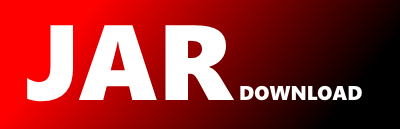
io.quarkus.smallrye.graphql.client.runtime.GraphQLClientConfig Maven / Gradle / Ivy
The newest version!
package io.quarkus.smallrye.graphql.client.runtime;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.OptionalInt;
import io.quarkus.runtime.annotations.ConfigDocMapKey;
import io.quarkus.runtime.annotations.ConfigGroup;
import io.quarkus.runtime.annotations.ConfigItem;
@ConfigGroup
public class GraphQLClientConfig {
/**
* The URL location of the target GraphQL service.
*/
@ConfigItem
public Optional url;
/**
* HTTP headers to add when communicating with the target GraphQL service.
*/
@ConfigItem(name = "header")
@ConfigDocMapKey("header-name")
public Map headers;
/**
* WebSocket subprotocols that should be supported by this client for running GraphQL operations over websockets.
* Allowed values are:
* - `graphql-ws` for the deprecated Apollo protocol
* - `graphql-transport-ws` for the newer GraphQL over WebSocket protocol (default value)
* If multiple protocols are provided, the actual protocol to be used will be subject to negotiation with
* the server.
*/
@ConfigItem(defaultValue = "graphql-transport-ws")
public Optional> subprotocols;
/**
* If true, then queries and mutations will run over the websocket transport rather than pure HTTP.
* Off by default, because it has higher overhead.
*/
@ConfigItem
public Optional executeSingleResultOperationsOverWebsocket;
/**
* Maximum time in milliseconds that will be allowed to wait for the server to acknowledge a websocket connection
* (send a subprotocol-specific ACK message).
*/
@ConfigItem
public OptionalInt websocketInitializationTimeout;
/**
* The trust store location. Can point to either a classpath resource or a file.
*
* @deprecated This configuration property is deprecated. Consider using the Quarkus TLS registry.
* Set the desired TLS bucket name using the following configuration property:
* {@code quarkus.smallrye-graphql-client."client-name".tls-bucket-name}.
*/
@ConfigItem
@Deprecated(forRemoval = true, since = "3.16.0")
public Optional trustStore;
/**
* The trust store password.
*
* @deprecated This configuration property is deprecated. Consider using the Quarkus TLS registry.
* Set the desired TLS bucket name using the following configuration property:
* {@code quarkus.smallrye-graphql-client."client-name".tls-bucket-name}.
*/
@ConfigItem
@Deprecated(forRemoval = true, since = "3.16.0")
public Optional trustStorePassword;
/**
* The type of the trust store. Defaults to "JKS".
*
* @deprecated This configuration property is deprecated. Consider using the Quarkus TLS registry.
* Set the desired TLS bucket name using the following configuration property:
* {@code quarkus.smallrye-graphql-client."client-name".tls-bucket-name}.
*/
@ConfigItem
@Deprecated(forRemoval = true, since = "3.16.0")
public Optional trustStoreType;
/**
* The key store location. Can point to either a classpath resource or a file.
*
* @deprecated This configuration property is deprecated. Consider using the Quarkus TLS registry.
* Set the desired TLS bucket name using the following configuration property:
* {@code quarkus.smallrye-graphql-client."client-name".tls-bucket-name}.
*/
@ConfigItem
@Deprecated(forRemoval = true, since = "3.16.0")
public Optional keyStore;
/**
* The key store password.
*
* @deprecated This configuration property is deprecated. Consider using the Quarkus TLS registry.
* Set the desired TLS bucket name using the following configuration property:
* {@code quarkus.smallrye-graphql-client."client-name".tls-bucket-name}.
*/
@ConfigItem
@Deprecated(forRemoval = true, since = "3.16.0")
public Optional keyStorePassword;
/**
* The type of the key store. Defaults to "JKS".
*
* @deprecated This configuration property is deprecated. Consider using the Quarkus TLS registry.
* Set the desired TLS bucket name using the following configuration property:
* {@code quarkus.smallrye-graphql-client."client-name".tls-bucket-name}.
*
*/
@ConfigItem
@Deprecated(forRemoval = true, since = "3.16.0")
public Optional keyStoreType;
/**
* Hostname of the proxy to use.
*/
@ConfigItem
public Optional proxyHost;
/**
* Port number of the proxy to use.
*/
@ConfigItem
public OptionalInt proxyPort;
/**
* Username for the proxy to use.
*/
@ConfigItem
public Optional proxyUsername;
/**
* Password for the proxy to use.
*/
@ConfigItem
public Optional proxyPassword;
/**
* Maximum number of redirects to follow.
*/
@ConfigItem
public OptionalInt maxRedirects;
/**
* Additional payload sent on websocket initialization.
*/
@ConfigItem(name = "init-payload")
@ConfigDocMapKey("property-name")
public Map initPayload;
/**
* Allowing unexpected fields in response.
* If true, there will be warning log of an unexpected field.
* Else it throws an error.
*/
@ConfigItem
public Optional allowUnexpectedResponseFields;
/**
* The name of the TLS configuration (bucket) used for client authentication in the TLS registry.
*/
@ConfigItem
public Optional tlsConfigurationName;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy