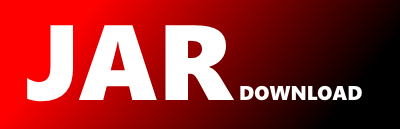
io.quarkus.smallrye.graphql.runtime.SmallRyeGraphQLAbstractHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-smallrye-graphql Show documentation
Show all versions of quarkus-smallrye-graphql Show documentation
Create GraphQL Endpoints using the code-first approach from MicroProfile GraphQL
package io.quarkus.smallrye.graphql.runtime;
import java.io.StringReader;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonReader;
import javax.json.JsonReaderFactory;
import io.quarkus.arc.Arc;
import io.quarkus.arc.InjectableContext;
import io.quarkus.arc.ManagedContext;
import io.quarkus.security.identity.CurrentIdentityAssociation;
import io.quarkus.security.identity.SecurityIdentity;
import io.quarkus.vertx.http.runtime.CurrentVertxRequest;
import io.quarkus.vertx.http.runtime.security.QuarkusHttpUser;
import io.smallrye.graphql.execution.ExecutionService;
import io.smallrye.graphql.execution.context.SmallRyeContextManager;
import io.vertx.core.Handler;
import io.vertx.core.MultiMap;
import io.vertx.ext.web.RoutingContext;
/**
* Handler that does the execution of GraphQL Requests
*/
public abstract class SmallRyeGraphQLAbstractHandler implements Handler {
private final CurrentIdentityAssociation currentIdentityAssociation;
private final CurrentVertxRequest currentVertxRequest;
private final ManagedContext currentManagedContext;
private final Handler currentManagedContextTerminationHandler;
private final Handler currentManagedContextExceptionHandler;
private final boolean runBlocking;
private volatile ExecutionService executionService;
protected static final JsonReaderFactory jsonReaderFactory = Json.createReaderFactory(null);
public SmallRyeGraphQLAbstractHandler(
CurrentIdentityAssociation currentIdentityAssociation,
CurrentVertxRequest currentVertxRequest,
boolean runBlocking) {
this.currentIdentityAssociation = currentIdentityAssociation;
this.currentVertxRequest = currentVertxRequest;
this.currentManagedContext = Arc.container().requestContext();
this.runBlocking = runBlocking;
this.currentManagedContextTerminationHandler = createCurrentManagedContextTerminationHandler();
this.currentManagedContextExceptionHandler = createCurrentManagedContextTerminationHandler();
}
private Handler createCurrentManagedContextTerminationHandler() {
return new Handler<>() {
@Override
public void handle(Object e) {
terminate();
}
};
}
@Override
public void handle(final RoutingContext ctx) {
ctx.response()
.endHandler(currentManagedContextTerminationHandler)
.exceptionHandler(currentManagedContextExceptionHandler)
.closeHandler(currentManagedContextTerminationHandler);
if (!currentManagedContext.isActive()) {
currentManagedContext.activate();
}
try {
handleWithIdentity(ctx);
deactivate();
} catch (Throwable t) {
terminate();
throw t;
}
}
private void handleWithIdentity(final RoutingContext ctx) {
if (currentIdentityAssociation != null) {
QuarkusHttpUser existing = (QuarkusHttpUser) ctx.user();
if (existing != null) {
SecurityIdentity identity = existing.getSecurityIdentity();
currentIdentityAssociation.setIdentity(identity);
} else {
currentIdentityAssociation.setIdentity(QuarkusHttpUser.getSecurityIdentity(ctx, null));
}
}
currentVertxRequest.setCurrent(ctx);
doHandle(ctx);
}
protected abstract void doHandle(final RoutingContext ctx);
protected JsonObject inputToJsonObject(String input) {
try (JsonReader jsonReader = jsonReaderFactory.createReader(new StringReader(input))) {
return jsonReader.readObject();
}
}
protected ExecutionService getExecutionService() {
if (this.executionService == null) {
this.executionService = Arc.container().instance(ExecutionService.class).get();
}
return this.executionService;
}
protected Map getMetaData(RoutingContext ctx) {
// Add some context to dfe
Map metaData = new ConcurrentHashMap<>();
metaData.put("runBlocking", runBlocking);
metaData.put("httpHeaders", getHeaders(ctx));
InjectableContext.ContextState state = currentManagedContext.getState();
metaData.put("state", state);
return metaData;
}
private Map> getHeaders(RoutingContext ctx) {
Map> h = new HashMap<>();
MultiMap headers = ctx.request().headers();
for (String header : headers.names()) {
h.put(header, headers.getAll(header));
}
return h;
}
private void deactivate() {
SmallRyeContextManager.clearCurrentSmallRyeContext();
currentManagedContext.deactivate();
}
private void terminate() {
SmallRyeContextManager.clearCurrentSmallRyeContext();
currentManagedContext.terminate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy