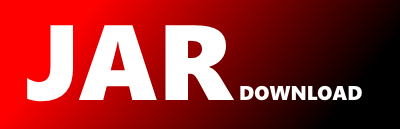
io.quarkus.smallrye.graphql.runtime.SmallRyeGraphQLRecorder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-smallrye-graphql Show documentation
Show all versions of quarkus-smallrye-graphql Show documentation
Create GraphQL Endpoints using the code-first approach from MicroProfile GraphQL
package io.quarkus.smallrye.graphql.runtime;
import java.util.List;
import java.util.function.Consumer;
import graphql.schema.GraphQLSchema;
import io.quarkus.arc.Arc;
import io.quarkus.arc.InstanceHandle;
import io.quarkus.arc.runtime.BeanContainer;
import io.quarkus.runtime.RuntimeValue;
import io.quarkus.runtime.ShutdownContext;
import io.quarkus.runtime.annotations.Recorder;
import io.quarkus.security.identity.CurrentIdentityAssociation;
import io.quarkus.smallrye.graphql.runtime.spi.QuarkusClassloadingService;
import io.quarkus.vertx.http.runtime.CurrentVertxRequest;
import io.quarkus.vertx.http.runtime.devmode.FileSystemStaticHandler;
import io.quarkus.vertx.http.runtime.webjar.WebJarNotFoundHandler;
import io.quarkus.vertx.http.runtime.webjar.WebJarStaticHandler;
import io.smallrye.graphql.cdi.producer.GraphQLProducer;
import io.smallrye.graphql.schema.model.Schema;
import io.vertx.core.Handler;
import io.vertx.ext.web.Route;
import io.vertx.ext.web.RoutingContext;
@Recorder
public class SmallRyeGraphQLRecorder {
public RuntimeValue createExecutionService(BeanContainer beanContainer, Schema schema) {
GraphQLProducer graphQLProducer = beanContainer.beanInstance(GraphQLProducer.class);
GraphQLSchema graphQLSchema = graphQLProducer.initialize(schema);
return new RuntimeValue<>(graphQLSchema != null);
}
public Handler executionHandler(RuntimeValue initialized, boolean allowGet,
boolean allowPostWithQueryParameters, boolean runBlocking) {
if (initialized.getValue()) {
return new SmallRyeGraphQLExecutionHandler(allowGet, allowPostWithQueryParameters, runBlocking,
getCurrentIdentityAssociation(),
Arc.container().instance(CurrentVertxRequest.class).get());
} else {
return new SmallRyeGraphQLNoEndpointHandler();
}
}
public Handler graphqlOverWebsocketHandler(BeanContainer beanContainer, RuntimeValue initialized,
boolean runBlocking) {
return new SmallRyeGraphQLOverWebSocketHandler(getCurrentIdentityAssociation(),
Arc.container().instance(CurrentVertxRequest.class).get(), runBlocking);
}
public Handler schemaHandler(RuntimeValue initialized, boolean schemaAvailable) {
if (initialized.getValue() && schemaAvailable) {
return new SmallRyeGraphQLSchemaHandler();
} else {
return new SmallRyeGraphQLNoEndpointHandler();
}
}
public Handler uiHandler(String graphqlUiFinalDestination,
String graphqlUiPath, List webRootConfigurations,
SmallRyeGraphQLRuntimeConfig runtimeConfig, ShutdownContext shutdownContext) {
if (runtimeConfig.enable) {
WebJarStaticHandler handler = new WebJarStaticHandler(graphqlUiFinalDestination, graphqlUiPath,
webRootConfigurations);
shutdownContext.addShutdownTask(new ShutdownContext.CloseRunnable(handler));
return handler;
} else {
return new WebJarNotFoundHandler();
}
}
public void setupClDevMode(ShutdownContext shutdownContext) {
QuarkusClassloadingService.setClassLoader(Thread.currentThread().getContextClassLoader());
shutdownContext.addShutdownTask(new Runnable() {
@Override
public void run() {
QuarkusClassloadingService.setClassLoader(null);
}
});
}
public Consumer routeFunction(Handler bodyHandler) {
return new Consumer() {
@Override
public void accept(Route route) {
route.handler(bodyHandler);
}
};
}
private CurrentIdentityAssociation getCurrentIdentityAssociation() {
InstanceHandle identityAssociations = Arc.container()
.instance(CurrentIdentityAssociation.class);
if (identityAssociations.isAvailable()) {
return identityAssociations.get();
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy