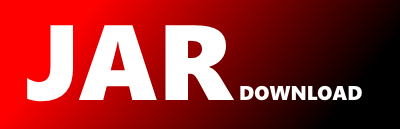
io.quarkus.smallrye.graphql.runtime.spi.datafetcher.QuarkusUniDataFetcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-smallrye-graphql Show documentation
Show all versions of quarkus-smallrye-graphql Show documentation
Create GraphQL Endpoints using the code-first approach from MicroProfile GraphQL
package io.quarkus.smallrye.graphql.runtime.spi.datafetcher;
import java.util.List;
import java.util.concurrent.Callable;
import graphql.schema.DataFetchingEnvironment;
import io.quarkus.arc.Arc;
import io.smallrye.context.SmallRyeThreadContext;
import io.smallrye.graphql.schema.model.Operation;
import io.smallrye.graphql.schema.model.Type;
import io.smallrye.mutiny.Uni;
import io.vertx.core.Context;
import io.vertx.core.Promise;
import io.vertx.core.Vertx;
public class QuarkusUniDataFetcher extends AbstractAsyncDataFetcher {
public QuarkusUniDataFetcher(Operation operation, Type type) {
super(operation, type);
}
@Override
protected Uni> handleUserMethodCall(DataFetchingEnvironment dfe, Object[] transformedArguments) throws Exception {
Context vc = Vertx.currentContext();
if (runBlocking(dfe) || !BlockingHelper.nonBlockingShouldExecuteBlocking(operation, vc)) {
return handleUserMethodCallNonBlocking(transformedArguments);
} else {
return handleUserMethodCallBlocking(transformedArguments, vc);
}
}
@Override
protected Uni> handleUserBatchLoad(DataFetchingEnvironment dfe, Object[] arguments) throws Exception {
Context vc = Vertx.currentContext();
if (runBlocking(dfe) || !BlockingHelper.nonBlockingShouldExecuteBlocking(operation, vc)) {
return handleUserBatchLoadNonBlocking(arguments);
} else {
return handleUserBatchLoadBlocking(arguments, vc);
}
}
private Uni> handleUserMethodCallNonBlocking(final Object[] transformedArguments)
throws Exception {
return (Uni>) operationInvoker.invoke(transformedArguments);
}
private Uni> handleUserMethodCallBlocking(Object[] transformedArguments, Context vc)
throws Exception {
SmallRyeThreadContext threadContext = Arc.container().select(SmallRyeThreadContext.class).get();
final Promise result = Promise.promise();
// We need some make sure that we call given the context
Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy