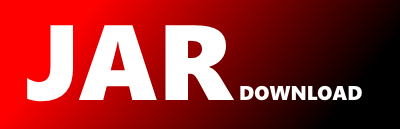
io.quarkus.websockets.next.OpenConnections Maven / Gradle / Ivy
Show all versions of quarkus-websockets-next Show documentation
package io.quarkus.websockets.next;
import java.util.Collection;
import java.util.Optional;
import java.util.stream.Stream;
import jakarta.enterprise.inject.Default;
import io.smallrye.common.annotation.Experimental;
/**
* Provides convenient access to all open connections.
*
* Quarkus provides a CDI bean with bean type {@link OpenConnections} and qualifier {@link Default}.
*/
@Experimental("This API is experimental and may change in the future")
public interface OpenConnections extends Iterable {
/**
* Returns an immutable snapshot of all open connections at the given time.
*
* @return an immutable collection of all open connections
*/
default Collection listAll() {
return stream().toList();
}
/**
* Returns an immutable snapshot of all open connections for the given endpoint id.
*
* @param endpointId
* @return an immutable collection of all open connections for the given endpoint id
* @see WebSocket#endpointId()
*/
default Collection findByEndpointId(String endpointId) {
return stream().filter(c -> c.endpointId().equals(endpointId)).toList();
}
/**
* Returns the open connection with the given id.
*
* @param connectionId
* @return the open connection or empty {@link Optional} if no open connection with the given id exists
* @see WebSocketConnection#id()
*/
default Optional findByConnectionId(String connectionId) {
return stream().filter(c -> c.id().equals(connectionId)).findFirst();
}
/**
* Returns the stream of all open connections at the given time.
*
* @return the stream of open connections
*/
Stream stream();
}