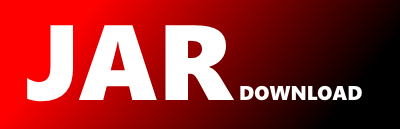
io.quarkus.websockets.next.Sender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-websockets-next Show documentation
Show all versions of quarkus-websockets-next Show documentation
Implementation of the WebSocket API with enhanced efficiency and usability
package io.quarkus.websockets.next;
import io.smallrye.common.annotation.CheckReturnValue;
import io.smallrye.common.annotation.Experimental;
import io.smallrye.mutiny.Uni;
import io.vertx.core.buffer.Buffer;
/**
* Sends messages to the connected WebSocket client.
*/
@Experimental("This API is experimental and may change in the future")
public interface Sender {
/**
* Send a text message.
*
* @param message
* @return a new {@link Uni} with a {@code null} item
*/
@CheckReturnValue
Uni sendText(String message);
/**
* Send a text message.
*
* @param
* @param message
* @return a new {@link Uni} with a {@code null} item
*/
@CheckReturnValue
Uni sendText(M message);
/**
* Send a binary message.
*
* @param message
* @return a new {@link Uni} with a {@code null} item
*/
@CheckReturnValue
Uni sendBinary(Buffer message);
/**
* Send a binary message.
*
* @param message
* @return a new {@link Uni} with a {@code null} item
*/
@CheckReturnValue
default Uni sendBinary(byte[] message) {
return sendBinary(Buffer.buffer(message));
}
/**
* Send a ping message.
*
* @param data May be at most 125 bytes
* @return a new {@link Uni} with a {@code null} item
*/
@CheckReturnValue
Uni sendPing(Buffer data);
/**
* Send an unsolicited pong message.
*
* Note that the server automatically responds to a ping message sent from the client. However, the RFC 6455
* section 5.5.3 states that unsolicited pong may serve as a
* unidirectional heartbeat.
*
* @param data May be at most 125 bytes
* @return a new {@link Uni} with a {@code null} item
*/
@CheckReturnValue
Uni sendPong(Buffer data);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy