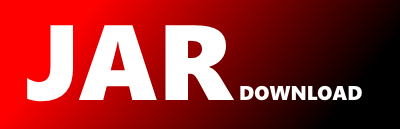
io.quarkus.websockets.next.WebSocketConnector Maven / Gradle / Ivy
Show all versions of quarkus-websockets-next Show documentation
package io.quarkus.websockets.next;
import java.net.URI;
import java.net.URLEncoder;
import jakarta.enterprise.inject.Default;
import jakarta.enterprise.inject.Instance;
import io.smallrye.common.annotation.CheckReturnValue;
import io.smallrye.common.annotation.Experimental;
import io.smallrye.mutiny.Uni;
/**
* A connector can be used to configure and open a new client connection backed by a client endpoint that is used to
* consume and send messages.
*
* Quarkus provides a CDI bean with bean type {@code WebSocketConnector} and qualifier {@link Default}. The actual type
* argument of an injection point is used to determine the client endpoint. The type is validated during build
* and if it does not represent a client endpoint then the build fails.
*
* This construct is not thread-safe and should not be used concurrently.
*
* Connectors should not be reused. If you need to create multiple connections in a row you'll need to obtain a new connetor
* instance programmatically using {@link Instance#get()}:
*
* import jakarta.enterprise.inject.Instance;
*
* @Inject
* Instance<WebSocketConnector<MyEndpoint>> connector;
*
* void connect() {
* var connection1 = connector.get().baseUri(uri)
* .addHeader("Foo", "alpha")
* .connectAndAwait();
* var connection2 = connector.get().baseUri(uri)
* .addHeader("Foo", "bravo")
* .connectAndAwait();
* }
*
*
* @param The client endpoint class
* @see WebSocketClient
* @see WebSocketClientConnection
*/
@Experimental("This API is experimental and may change in the future")
public interface WebSocketConnector {
/**
* Set the base URI.
*
* @param baseUri
* @return self
*/
WebSocketConnector baseUri(URI baseUri);
/**
* Set the base URI.
*
* @param baseUri
* @return self
*/
default WebSocketConnector baseUri(String baseUri) {
return baseUri(URI.create(baseUri));
}
/**
* Set the path param.
*
* The value is encoded using {@link URLEncoder#encode(String, java.nio.charset.Charset)} before it's used to build the
* target URI.
*
* @param name
* @param value
* @return self
* @throws IllegalArgumentException If the client endpoint path does not contain a parameter with the given name
* @see WebSocketClient#path()
*/
WebSocketConnector pathParam(String name, String value);
/**
* Add a header used during the initial handshake request.
*
* @param name
* @param value
* @return self
* @see HandshakeRequest
*/
WebSocketConnector addHeader(String name, String value);
/**
* Add the subprotocol.
*
* @param name
* @param value
* @return self
*/
WebSocketConnector addSubprotocol(String value);
/**
*
* @return a new {@link Uni} with a {@link WebSocketClientConnection} item
*/
@CheckReturnValue
Uni connect();
/**
*
* @return the client connection
*/
default WebSocketClientConnection connectAndAwait() {
return connect().await().indefinitely();
}
}