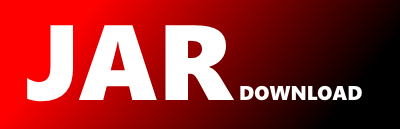
io.quarkus.websockets.next.WebSocketsServerRuntimeConfig Maven / Gradle / Ivy
Show all versions of quarkus-websockets-next Show documentation
package io.quarkus.websockets.next;
import java.time.Duration;
import java.util.List;
import java.util.Optional;
import java.util.OptionalInt;
import io.quarkus.runtime.annotations.ConfigPhase;
import io.quarkus.runtime.annotations.ConfigRoot;
import io.smallrye.config.ConfigMapping;
import io.smallrye.config.WithDefault;
import io.smallrye.config.WithParentName;
@ConfigMapping(prefix = "quarkus.websockets-next.server")
@ConfigRoot(phase = ConfigPhase.RUN_TIME)
public interface WebSocketsServerRuntimeConfig {
/**
* See The WebSocket Protocol
*/
Optional> supportedSubprotocols();
/**
* Compression Extensions for WebSocket are supported by default.
*
* See also RFC 7692
*/
@WithDefault("true")
boolean perMessageCompressionSupported();
/**
* The compression level must be a value between 0 and 9. The default value is
* {@value io.vertx.core.http.HttpServerOptions#DEFAULT_WEBSOCKET_COMPRESSION_LEVEL}.
*/
OptionalInt compressionLevel();
/**
* The maximum size of a message in bytes. The default values is
* {@value io.vertx.core.http.HttpServerOptions#DEFAULT_MAX_WEBSOCKET_MESSAGE_SIZE}.
*/
OptionalInt maxMessageSize();
/**
* The interval after which, when set, the server sends a ping message to a connected client automatically.
*
* Ping messages are not sent automatically by default.
*/
Optional autoPingInterval();
/**
* The strategy used when an error occurs but no error handler can handle the failure.
*
* By default, the error message is logged and the connection is closed when an unhandled failure occurs.
*/
@WithDefault("log-and-close")
UnhandledFailureStrategy unhandledFailureStrategy();
/**
* WebSockets-specific security configuration.
*/
Security security();
/**
* Dev mode configuration.
*/
DevMode devMode();
/**
* Traffic logging config.
*/
TrafficLoggingConfig trafficLogging();
/**
* Telemetry configuration.
*/
@WithParentName
TelemetryConfig telemetry();
interface Security {
/**
* Quarkus redirects HTTP handshake request to this URL if an HTTP upgrade is rejected due to the authorization
* failure. This configuration property takes effect when you secure endpoint with a standard security annotation.
* For example, the HTTP upgrade is secured if an endpoint class is annotated with the `@RolesAllowed` annotation.
*/
Optional authFailureRedirectUrl();
}
interface DevMode {
/**
* The limit of messages kept for a Dev UI connection. If less than zero then no messages are stored and sent to the Dev
* UI view.
*/
@WithDefault("1000")
long connectionMessagesLimit();
}
}