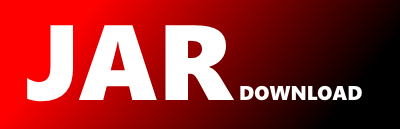
io.quarkus.websockets.next.runtime.WebSocketClientConnectionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quarkus-websockets-next Show documentation
Show all versions of quarkus-websockets-next Show documentation
Implementation of the WebSocket API with enhanced efficiency and usability
package io.quarkus.websockets.next.runtime;
import java.net.URI;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import io.quarkus.websockets.next.HandshakeRequest;
import io.quarkus.websockets.next.WebSocketClientConnection;
import io.vertx.core.http.WebSocket;
import io.vertx.core.http.WebSocketBase;
class WebSocketClientConnectionImpl extends WebSocketConnectionBase implements WebSocketClientConnection {
private final String clientId;
private final WebSocket webSocket;
WebSocketClientConnectionImpl(String clientId, WebSocket webSocket, Codecs codecs,
Map pathParams, URI serverEndpointUri, Map> headers,
TrafficLogger trafficLogger) {
super(Map.copyOf(pathParams), codecs, new ClientHandshakeRequestImpl(serverEndpointUri, headers), trafficLogger);
this.clientId = clientId;
this.webSocket = Objects.requireNonNull(webSocket);
}
@Override
WebSocketBase webSocket() {
return webSocket;
}
@Override
public String clientId() {
return clientId;
}
@Override
public String toString() {
return "WebSocket client connection [id=" + identifier + ", clientId=" + clientId + "]";
}
@Override
public int hashCode() {
return Objects.hash(identifier);
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
WebSocketClientConnectionImpl other = (WebSocketClientConnectionImpl) obj;
return Objects.equals(identifier, other.identifier);
}
private static class ClientHandshakeRequestImpl implements HandshakeRequest {
private final URI serverEndpointUrl;
private final Map> headers;
ClientHandshakeRequestImpl(URI serverEndpointUrl, Map> headers) {
this.serverEndpointUrl = serverEndpointUrl;
Map> copy = new HashMap<>();
for (Entry> e : headers.entrySet()) {
copy.put(e.getKey().toLowerCase(), List.copyOf(e.getValue()));
}
this.headers = copy;
}
@Override
public String header(String name) {
List values = headers(name);
return values.isEmpty() ? null : values.get(0);
}
@Override
public List headers(String name) {
return headers.getOrDefault(Objects.requireNonNull(name).toLowerCase(), List.of());
}
@Override
public Map> headers() {
return headers;
}
@Override
public String scheme() {
return serverEndpointUrl.getScheme();
}
@Override
public String host() {
return serverEndpointUrl.getHost();
}
@Override
public int port() {
return serverEndpointUrl.getPort();
}
@Override
public String path() {
return serverEndpointUrl.getPath();
}
@Override
public String query() {
return serverEndpointUrl.getQuery();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy