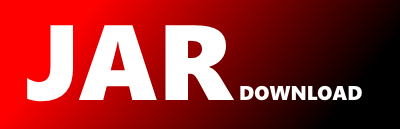
io.rapidpro.expressions.ExcellentVisitor Maven / Gradle / Ivy
// Generated from io/rapidpro/expressions/Excellent.g4 by ANTLR 4.5
package io.rapidpro.expressions;
import org.antlr.v4.runtime.misc.NotNull;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link ExcellentParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface ExcellentVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link ExcellentParser#parse}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParse(ExcellentParser.ParseContext ctx);
/**
* Visit a parse tree produced by the {@code decimalLiteral}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDecimalLiteral(ExcellentParser.DecimalLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code parentheses}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParentheses(ExcellentParser.ParenthesesContext ctx);
/**
* Visit a parse tree produced by the {@code negation}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNegation(ExcellentParser.NegationContext ctx);
/**
* Visit a parse tree produced by the {@code exponentExpression}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExponentExpression(ExcellentParser.ExponentExpressionContext ctx);
/**
* Visit a parse tree produced by the {@code additionOrSubtractionExpression}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAdditionOrSubtractionExpression(ExcellentParser.AdditionOrSubtractionExpressionContext ctx);
/**
* Visit a parse tree produced by the {@code false}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFalse(ExcellentParser.FalseContext ctx);
/**
* Visit a parse tree produced by the {@code contextReference}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitContextReference(ExcellentParser.ContextReferenceContext ctx);
/**
* Visit a parse tree produced by the {@code comparisonExpression}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComparisonExpression(ExcellentParser.ComparisonExpressionContext ctx);
/**
* Visit a parse tree produced by the {@code concatenation}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConcatenation(ExcellentParser.ConcatenationContext ctx);
/**
* Visit a parse tree produced by the {@code stringLiteral}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringLiteral(ExcellentParser.StringLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code functionCall}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionCall(ExcellentParser.FunctionCallContext ctx);
/**
* Visit a parse tree produced by the {@code true}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrue(ExcellentParser.TrueContext ctx);
/**
* Visit a parse tree produced by the {@code equalityExpression}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEqualityExpression(ExcellentParser.EqualityExpressionContext ctx);
/**
* Visit a parse tree produced by the {@code multiplicationOrDivisionExpression}
* labeled alternative in {@link ExcellentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultiplicationOrDivisionExpression(ExcellentParser.MultiplicationOrDivisionExpressionContext ctx);
/**
* Visit a parse tree produced by {@link ExcellentParser#fnname}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFnname(ExcellentParser.FnnameContext ctx);
/**
* Visit a parse tree produced by the {@code functionParameters}
* labeled alternative in {@link ExcellentParser#parameters}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionParameters(ExcellentParser.FunctionParametersContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy