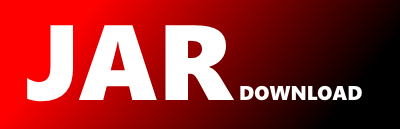
ratpack.exec.internal.DefaultPromise Maven / Gradle / Ivy
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ratpack.exec.internal;
import ratpack.exec.*;
import ratpack.func.Action;
import ratpack.func.Function;
import ratpack.func.NoArgAction;
import ratpack.func.Predicate;
import java.util.function.Consumer;
import java.util.function.Supplier;
public class DefaultPromise implements Promise {
private final Consumer super Fulfiller super T>> fulfillment;
private final Supplier executionProvider;
public DefaultPromise(Supplier executionProvider, Consumer super Fulfiller super T>> fulfillment) {
this.executionProvider = executionProvider;
this.fulfillment = fulfillment;
}
@Override
public SuccessPromise onError(final Action super Throwable> errorHandler) {
return new DefaultSuccessPromise<>(executionProvider, fulfillment, errorHandler);
}
@Override
public void then(Action super T> then) {
propagatingSuccessPromise().then(then);
}
private SuccessPromise propagatingSuccessPromise() {
return onError(Action.throwException());
}
@Override
public Promise map(Function super T, ? extends O> transformer) {
return propagatingSuccessPromise().map(transformer);
}
@Override
public Promise blockingMap(Function super T, ? extends O> transformer) {
return propagatingSuccessPromise().blockingMap(transformer);
}
@Override
public Promise flatMap(Function super T, ? extends Promise> transformer) {
return propagatingSuccessPromise().flatMap(transformer);
}
@Override
public Promise route(Predicate super T> predicate, Action super T> action) {
return propagatingSuccessPromise().route(predicate, action);
}
@Override
public Promise onNull(NoArgAction action) {
return propagatingSuccessPromise().onNull(action);
}
@Override
public Promise cache() {
return propagatingSuccessPromise().cache();
}
@Override
public Promise defer(Action super Runnable> releaser) {
return propagatingSuccessPromise().defer(releaser);
}
@Override
public Promise onYield(Runnable onYield) {
return propagatingSuccessPromise().onYield(onYield);
}
@Override
public Promise wiretap(Action super Result> listener) {
return propagatingSuccessPromise().wiretap(listener);
}
@Override
public Promise throttled(Throttle throttle) {
return propagatingSuccessPromise().throttled(throttle);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy