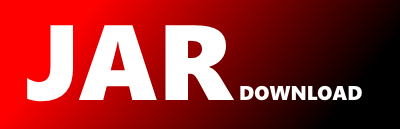
ratpack.registry.internal.CachingBackedRegistry Maven / Gradle / Ivy
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ratpack.registry.internal;
import com.google.common.base.Predicate;
import com.google.common.base.Supplier;
import com.google.common.collect.Iterables;
import com.google.common.reflect.TypeToken;
import ratpack.func.Action;
import ratpack.registry.PredicateCacheability;
import ratpack.registry.Registry;
import ratpack.registry.RegistryBacking;
import ratpack.util.Types;
import java.util.Iterator;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.function.Function;
public class CachingBackedRegistry implements Registry {
private final RegistryBacking registryBacking;
public CachingBackedRegistry(RegistryBacking registryBacking) {
this.registryBacking = registryBacking;
}
private final ConcurrentMap, Iterable extends Supplier>>> supplierCache = new ConcurrentHashMap<>();
private final ConcurrentMap, Iterable extends Supplier>>> predicateCache = new ConcurrentHashMap<>();
public Optional maybeGet(TypeToken type) {
Iterator extends Supplier> suppliers = getSuppliers(type).iterator();
if (!suppliers.hasNext()) {
return Optional.empty();
} else {
return Optional.of(suppliers.next().get());
}
}
private static V compute(Map map, K key, Function super K, ? extends V> supplier) {
V value = map.get(key);
if (value == null) {
value = supplier.apply(key);
map.put(key, value);
}
return value;
}
@Override
public Iterable extends O> getAll(TypeToken type) {
return transformToInstances(getSuppliers(type));
}
protected Iterable transformToInstances(Iterable extends Supplier> suppliers) {
//noinspection Convert2MethodRef
return Iterables.transform(suppliers, (s) -> s.get());
}
protected Iterable extends Supplier> getSuppliers(TypeToken type) {
return Types.cast(compute(supplierCache, type, t -> registryBacking.provide(type)));
}
protected Iterable extends Supplier> getSuppliers(TypeToken type, Predicate super T> predicate) {
return Types.cast(
compute(predicateCache, new PredicateCacheability.CacheKey<>(type, predicate), key ->
Iterables.filter(getSuppliers(type), new Predicate>() {
@Override
public boolean apply(Supplier input) {
return predicate.apply(input.get());
}
})
)
);
}
@Override
public Optional first(TypeToken type, Predicate super T> predicate) {
Iterator extends T> matching = all(type, predicate).iterator();
if (!matching.hasNext()) {
return Optional.empty();
} else {
return Optional.of(matching.next());
}
}
@Override
public Iterable extends T> all(TypeToken type, Predicate super T> predicate) {
if (PredicateCacheability.isCacheable(predicate)) {
return transformToInstances(getSuppliers(type, predicate));
} else {
return Iterables.filter(getAll(type), predicate);
}
}
@Override
public boolean each(TypeToken type, Predicate super T> predicate, Action super T> action) throws Exception {
if (PredicateCacheability.isCacheable(predicate)) {
Iterable extends T> all = all(type, predicate);
boolean any = false;
for (T t : all) {
any = true;
action.execute(t);
}
return any;
} else {
boolean foundMatch = false;
Iterable extends Supplier> suppliers = getSuppliers(type);
for (Supplier supplier : suppliers) {
T instance = supplier.get();
if (predicate.apply(instance)) {
action.execute(instance);
foundMatch = true;
}
}
return foundMatch;
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CachingBackedRegistry that = (CachingBackedRegistry) o;
return registryBacking.equals(that.registryBacking);
}
@Override
public int hashCode() {
return registryBacking.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy