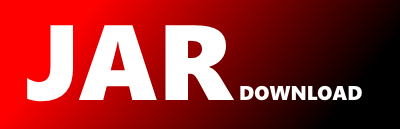
ratpack.server.internal.NettyHandlerAdapter Maven / Gradle / Ivy
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ratpack.server.internal;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.*;
import io.netty.handler.codec.http.*;
import io.netty.util.CharsetUtil;
import ratpack.error.ClientErrorHandler;
import ratpack.error.ServerErrorHandler;
import ratpack.error.internal.DefaultClientErrorHandler;
import ratpack.error.internal.DefaultServerErrorHandler;
import ratpack.error.internal.ErrorCatchingHandler;
import ratpack.event.internal.DefaultEventController;
import ratpack.file.FileRenderer;
import ratpack.file.FileSystemBinding;
import ratpack.file.MimeTypes;
import ratpack.file.internal.ActivationBackedMimeTypes;
import ratpack.file.internal.DefaultFileHttpTransmitter;
import ratpack.file.internal.DefaultFileRenderer;
import ratpack.file.internal.FileHttpTransmitter;
import ratpack.form.internal.FormParser;
import ratpack.func.Action;
import ratpack.handling.*;
import ratpack.handling.direct.DirectChannelAccess;
import ratpack.handling.direct.internal.DefaultDirectChannelAccess;
import ratpack.handling.internal.*;
import ratpack.http.*;
import ratpack.http.internal.*;
import ratpack.launch.LaunchConfig;
import ratpack.launch.internal.LaunchConfigInternal;
import ratpack.registry.Registries;
import ratpack.registry.Registry;
import ratpack.render.CharSequenceRenderer;
import ratpack.render.internal.DefaultCharSequenceRenderer;
import ratpack.render.internal.DefaultRenderController;
import ratpack.server.BindAddress;
import ratpack.server.PublicAddress;
import ratpack.server.Stopper;
import ratpack.util.internal.NumberUtil;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.util.concurrent.ConcurrentHashMap;
import static io.netty.handler.codec.http.HttpHeaders.isKeepAlive;
import static io.netty.handler.codec.http.HttpResponseStatus.NOT_FOUND;
@ChannelHandler.Sharable
public class NettyHandlerAdapter extends SimpleChannelInboundHandler {
private final Handler[] handlers;
private final Handler return404;
private final ConcurrentHashMap> channelSubscriptions = new ConcurrentHashMap<>(0);
private final DefaultContext.ApplicationConstants applicationConstants;
private final FinishedOnThreadCallbackManager finishedOnThreadCallbackManager = new FinishedOnThreadCallbackManager();
private Registry registry;
private final boolean addResponseTimeHeader;
private final boolean compressResponses;
public NettyHandlerAdapter(Stopper stopper, Handler handler, LaunchConfig launchConfig) {
this.handlers = new Handler[]{new ErrorCatchingHandler(handler)};
this.return404 = new ClientErrorForwardingHandler(NOT_FOUND.code());
this.registry = Registries.registry()
// If you update this list, update the class level javadoc on Context.
.add(Background.class, launchConfig.getBackground())
.add(finishedOnThreadCallbackManager)
.add(Stopper.class, stopper)
.add(FileSystemBinding.class, launchConfig.getBaseDir())
.add(MimeTypes.class, new ActivationBackedMimeTypes())
.add(PublicAddress.class, new DefaultPublicAddress(launchConfig.getPublicAddress(), launchConfig.getSSLContext() == null ? "http" : "https"))
.add(Redirector.class, new DefaultRedirector())
.add(ClientErrorHandler.class, new DefaultClientErrorHandler())
.add(ServerErrorHandler.class, new DefaultServerErrorHandler())
.add(LaunchConfig.class, launchConfig)
.add(FileRenderer.class, new DefaultFileRenderer())
.add(CharSequenceRenderer.class, new DefaultCharSequenceRenderer())
.add(FormParser.class, FormParser.multiPart())
.add(FormParser.class, FormParser.urlEncoded())
.build();
ContextStorage contextStorage;
if (launchConfig instanceof LaunchConfigInternal) {
contextStorage = ((LaunchConfigInternal) launchConfig).getContextStorage();
} else {
throw new IllegalArgumentException("launchConfig must implement internal protocol " + LaunchConfigInternal.class.getName());
}
this.addResponseTimeHeader = launchConfig.isTimeResponses();
this.compressResponses = launchConfig.isCompressResponses();
this.applicationConstants = new DefaultContext.ApplicationConstants(launchConfig.getForeground(), launchConfig.getBackground(), contextStorage, new DefaultRenderController());
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if (!(msg instanceof FullHttpRequest)) {
Action
© 2015 - 2025 Weber Informatics LLC | Privacy Policy