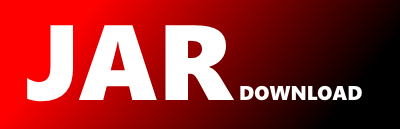
ratpack.server.internal.SmartHttpContentCompressor Maven / Gradle / Ivy
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ratpack.server.internal;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.http.HttpContentCompressor;
import io.netty.handler.codec.http.HttpHeaders;
import io.netty.handler.codec.http.HttpObject;
import io.netty.handler.codec.http.HttpResponse;
import java.util.List;
/**
* Adapted from https://github.com/scireum/sirius/blob/develop/web/src/sirius/web/http/SmartHttpContentCompressor.java
*
* Credit to Andreas Haufler ([email protected]).
*/
public class SmartHttpContentCompressor extends HttpContentCompressor {
private boolean passThrough;
@Override
protected void encode(ChannelHandlerContext ctx, HttpObject msg, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy