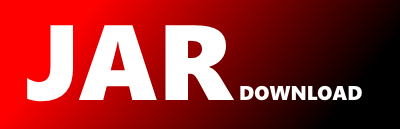
ratpack.func.BiAction Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ratpack.func;
import ratpack.util.Exceptions;
import java.util.function.BiConsumer;
/**
* A generic type for an object that does some work with 2 input things.
*
* This type serves the same purpose as the JDK's {@link java.util.function.BiConsumer}, but allows throwing checked exceptions.
* It contains methods for bridging to and from the JDK type.
*
* @param The type of the first thing.
* @param The type of the second thing.
*/
@FunctionalInterface
public interface BiAction {
/**
* Executes the action against the given thing.
*
* @param t the first thing to input to the action
* @param u the second thing to input to the action
* @throws Exception if anything goes wrong
*/
void execute(T t, U u) throws Exception;
/**
* Creates a JDK {@link java.util.function.BiConsumer} from this action.
*
* Any exceptions thrown by {@code this} action will be unchecked via {@link ratpack.util.Exceptions#uncheck(Throwable)} and rethrown.
*
* @return this function as a JDK style consumer.
*/
default BiConsumer toBiConsumer() {
return (t, u) -> {
try {
execute(t, u);
} catch (Exception e) {
throw Exceptions.uncheck(e);
}
};
}
/**
* Creates an bi-action from a JDK bi-consumer.
*
* @param consumer the JDK consumer
* @param the type of the first object this action accepts
* @param the type of the second object this action accepts
* @return the given consumer as an action
*/
static BiAction from(BiConsumer consumer) {
return consumer::accept;
}
}