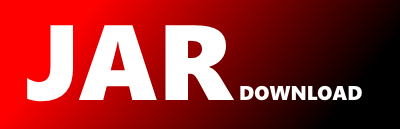
ratpack.guice.internal.InjectorBackedRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ratpack-guice Show documentation
Show all versions of ratpack-guice Show documentation
Integration with Google Guice for Ratpack applications - https://code.google.com/p/google-guice/
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ratpack.guice.internal;
import com.google.common.base.Function;
import com.google.common.base.Predicate;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Iterables;
import com.google.common.reflect.TypeToken;
import com.google.common.util.concurrent.UncheckedExecutionException;
import com.google.inject.Injector;
import com.google.inject.Provider;
import ratpack.api.Nullable;
import ratpack.func.Action;
import ratpack.registry.NotInRegistryException;
import ratpack.registry.PredicateCacheability;
import ratpack.registry.Registry;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.ExecutionException;
import static ratpack.util.ExceptionUtils.toException;
import static ratpack.util.ExceptionUtils.uncheck;
public class InjectorBackedRegistry implements Registry {
final Injector injector;
public InjectorBackedRegistry(Injector injector) {
this.injector = injector;
}
private final LoadingCache, List>> providerCache = CacheBuilder.newBuilder().build(new CacheLoader, List>>() {
@Override
public List> load(@SuppressWarnings("NullableProblems") TypeToken> key) throws Exception {
@SuppressWarnings({"unchecked", "RedundantCast"})
List> providers = (List>) GuiceUtil.allProvidersOfType(injector, key);
return providers;
}
});
private final LoadingCache, List>> predicateCache = CacheBuilder.newBuilder().build(new CacheLoader, List>>() {
@Override
public List> load(@SuppressWarnings("NullableProblems") PredicateCacheability.CacheKey> key) throws Exception {
return get(key);
}
private List> get(PredicateCacheability.CacheKey key) throws ExecutionException {
List> providers = getProviders(key.type);
if (providers.isEmpty()) {
return Collections.emptyList();
} else {
ImmutableList.Builder> builder = ImmutableList.builder();
Predicate super T> predicate = key.predicate;
for (Provider> provider : providers) {
@SuppressWarnings("unchecked") Provider castProvider = (Provider) provider;
if (predicate.apply(castProvider.get())) {
builder.add(castProvider);
}
}
return builder.build();
}
}
});
@Override
public O get(Class type) throws NotInRegistryException {
return get(TypeToken.of(type));
}
@Override
public O get(TypeToken type) throws NotInRegistryException {
O object = maybeGet(type);
if (object == null) {
throw new NotInRegistryException(type);
} else {
return object;
}
}
public T maybeGet(Class type) {
return maybeGet(TypeToken.of(type));
}
public T maybeGet(TypeToken type) {
List> providers = getProviders(type);
if (providers.isEmpty()) {
return null;
} else {
@SuppressWarnings("unchecked") T cast = (T) providers.get(0).get();
return cast;
}
}
private List> getProviders(TypeToken type) {
try {
return providerCache.get(type);
} catch (ExecutionException | UncheckedExecutionException e) {
throw uncheck(toException(e.getCause()));
}
}
@Override
public Iterable extends O> getAll(Class type) {
return getAll(TypeToken.of(type));
}
@Override
public Iterable extends O> getAll(TypeToken type) {
final List> providers = getProviders(type);
if (providers.isEmpty()) {
return Collections.emptyList();
} else {
return Iterables.transform(providers, new Function, O>() {
@Override
public O apply(Provider> input) {
@SuppressWarnings("unchecked") O cast = (O) input.get();
return cast;
}
});
}
}
private List> getAll(TypeToken type, Predicate super T> predicate) {
try {
return predicateCache.get(new PredicateCacheability.CacheKey<>(type, predicate));
} catch (ExecutionException | UncheckedExecutionException e) {
throw uncheck(toException(e.getCause()));
}
}
@Nullable
@Override
public T first(TypeToken type, Predicate super T> predicate) {
if (PredicateCacheability.isCacheable(predicate)) {
List> all = getAll(type, predicate);
if (all.isEmpty()) {
return null;
} else {
@SuppressWarnings("unchecked") T cast = (T) all.get(0).get();
return cast;
}
} else {
T object = maybeGet(type);
if (object != null && predicate.apply(object)) {
return object;
} else {
return null;
}
}
}
@Override
public Iterable extends T> all(TypeToken type, Predicate super T> predicate) {
if (PredicateCacheability.isCacheable(predicate)) {
@SuppressWarnings("unchecked") List extends T> cast = (List extends T>) getAll(type, predicate);
return cast;
} else {
return Iterables.filter(getAll(type), predicate);
}
}
@Override
public boolean each(TypeToken type, Predicate super T> predicate, Action super T> action) throws Exception {
if (PredicateCacheability.isCacheable(predicate)) {
Iterable extends T> all = all(type, predicate);
boolean any = false;
for (T t : all) {
any = true;
action.execute(t);
}
return any;
} else {
boolean foundMatch = false;
List> providers = getProviders(type);
for (Provider> provider : providers) {
@SuppressWarnings("unchecked") T cast = (T) provider.get();
if (predicate.apply(cast)) {
action.execute(cast);
foundMatch = true;
}
}
return foundMatch;
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InjectorBackedRegistry that = (InjectorBackedRegistry) o;
return injector.equals(that.injector);
}
@Override
public int hashCode() {
return injector.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy