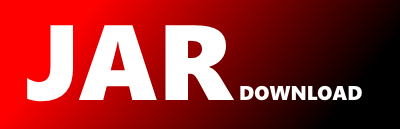
ratpack.test.handling.RequestFixture Maven / Gradle / Ivy
Show all versions of ratpack-test Show documentation
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ratpack.test.handling;
import com.google.common.net.HostAndPort;
import ratpack.func.Action;
import ratpack.handling.Chain;
import ratpack.handling.Handler;
import ratpack.launch.LaunchConfigBuilder;
import ratpack.registry.RegistrySpec;
import java.nio.file.Path;
import java.util.Map;
/**
* A contrived request environment, suitable for unit testing {@link Handler} implementations.
*
* A request fixture emulates a request, and the effective state of the request handling in the handler pipeline.
*
* A request fixture can be obtained by the {@link ratpack.test.UnitTest#requestFixture()} method.
* However it is often more convenient to use the alternative {@link ratpack.test.UnitTest#handle(ratpack.handling.Handler, ratpack.func.Action)} method.
*
* See {@link ratpack.test.UnitTest} for usage examples.
*
* @see ratpack.test.UnitTest
* @see #handle(ratpack.handling.Handler)
*/
public interface RequestFixture {
/**
* Sets the request body to be the given bytes, and adds a {@code Content-Type} request header of the given value.
*
* By default the body is empty.
*
* @param bytes the request body in bytes
* @param contentType the content type of the request body
* @return this
*/
RequestFixture body(byte[] bytes, String contentType);
/**
* Sets the request body to be the given string in utf8 bytes, and adds a {@code Content-Type} request header of the given value.
*
* By default the body is empty.
*
* @param text the request body as a string
* @param contentType the content type of the request body
* @return this
*/
RequestFixture body(String text, String contentType);
/**
* A specification of the context registry.
*
* Can be used to make objects (e.g. support services) available via context registry lookup.
*
* By default, only a {@link ratpack.error.ServerErrorHandler} and {@link ratpack.error.ClientErrorHandler} are in the context registry.
*
* @return a specification of the context registry
*/
RegistrySpec getRegistry();
/**
* Invokes the given handler with a newly created {@link ratpack.handling.Context} based on the state of this fixture.
*
* The return value can be used to examine the effective result of the handler.
*
* A result may be one of the following:
*
* - The sending of a response via one of the {@link ratpack.http.Response#send} methods
* - Rendering to the response via the {@link ratpack.handling.Context#render(Object)}
* - Raising of a client error via {@link ratpack.handling.Context#clientError(int)}
* - Raising of a server error via {@link ratpack.handling.Context#error(Throwable)}
* - Raising of a server error by the throwing of an exception
* - Delegating to the next handler by invoking one of the {@link ratpack.handling.Context#next} methods
*
* Note that any handlers {@link ratpack.handling.Context#insert inserted} by the handler under test will be invoked.
* If the last inserted handler delegates to the next handler, the handling will terminate with a result indicating that the effective result was delegating to the next handler.
*
* This method blocks until a result is achieved, even if the handler performs an asynchronous operation (such as performing {@link ratpack.handling.Context#blocking(java.util.concurrent.Callable) blocking IO}).
* As such, a time limit on the execution is imposed which by default is 5 seconds.
* The time limit can be changed via the {@link #timeout(int)} method.
* If the time limit is reached, a {@link HandlerTimeoutException} is thrown.
*
* @param handler the handler to test
* @return the effective result of the handling
* @throws HandlerTimeoutException if the handler does not produce a result in the time limit defined by this fixture
*/
HandlingResult handle(Handler handler) throws HandlerTimeoutException;
/**
* Similar to {@link #handle(ratpack.handling.Handler)}, but for testing a handler chain.
*
* @param chainAction the handler chain to test
* @return the effective result of the handling
* @throws HandlerTimeoutException if the handler does not produce a result in the time limit defined by this fixture
* @throws Exception any thrown by {@code chainAction}
*/
HandlingResult handleChain(Action super Chain> chainAction) throws Exception;
/**
* Set a request header value.
*
* By default there are no request headers.
*
* @param name the header name
* @param value the header value
* @return this
*/
RequestFixture header(String name, String value);
/**
* Configures the launch config to have the given base dir and given configuration.
*
* By default the launch config is equivalent to {@link ratpack.launch.LaunchConfigBuilder#noBaseDir() LaunchConfigBuilder.noBaseDir()}.{@link ratpack.launch.LaunchConfigBuilder#build() build()}.
*
* @param baseDir the launch config base dir
* @param action configuration of the launch config
* @return this
* @throws Exception any thrown by {@code action}
*/
RequestFixture launchConfig(Path baseDir, Action super LaunchConfigBuilder> action) throws Exception;
/**
* Configures the launch config to have no base dir and given configuration.
*
* By default the launch config is equivalent to {@link ratpack.launch.LaunchConfigBuilder#noBaseDir() LaunchConfigBuilder.noBaseDir()}.{@link ratpack.launch.LaunchConfigBuilder#build() build()}.
*
* @param action configuration of the launch config
* @return this
* @throws Exception any thrown by {@code action}
*/
RequestFixture launchConfig(Action super LaunchConfigBuilder> action) throws Exception;
/**
* Set the request method (case insensitive).
*
* The default method is {@code "GET"}.
*
* @param method the request method
* @return this
*/
RequestFixture method(String method);
/**
* Adds a path binding, with the given path tokens.
*
* By default, there are no path tokens and no path binding.
*
* @param pathTokens the path tokens to make available to the handler(s) under test
* @return this
*/
RequestFixture pathBinding(Map pathTokens);
/**
* Adds a path binding, with the given path tokens and parts.
*
* By default, there are no path tokens and no path binding.
*
* @param boundTo the part of the request path that the binding bound to
* @param pastBinding the part of the request path past {@code boundTo}
* @param pathTokens the path tokens and binding to make available to the handler(s) under test
* @return this
*/
RequestFixture pathBinding(String boundTo, String pastBinding, Map pathTokens);
/**
* Configures the context registry.
*
* @param action a registry specification action
* @return this
* @throws Exception any thrown by {@code action}
*/
RequestFixture registry(Action super RegistrySpec> action) throws Exception;
/**
* Set a response header value.
*
* Can be used to simulate the setting of a response header by an upstream handler.
*
* By default there are no request headers.
*
* @param name the header name
* @param value the header value
* @return this
*/
RequestFixture responseHeader(String name, String value);
/**
* Sets the maximum time to allow the handler under test to produce a result.
*
* As handlers may execute asynchronously, a maximum time limit must be used to guard against never ending handlers.
*
* @param timeoutSeconds the maximum number of seconds to allow the handler(s) under test to produce a result
* @return this
*/
RequestFixture timeout(int timeoutSeconds);
/**
* The URI of the request.
*
* No encoding is performed on the given value.
* It is expected to be a well formed URI path string (potentially including query and fragment strings)
*
* @param uri the URI of the request
* @return this
*/
RequestFixture uri(String uri);
/**
* Set the remote address from which the request is made.
*
* Effectively the return value of {@link ratpack.http.Request#getRemoteAddress()}.
* @param remote the remote host and port address
* @return this
*/
RequestFixture remoteAddress(HostAndPort remote);
/**
* Set the local address to which this request is made.
*
* Effectively the return value of {@link ratpack.http.Request#getLocalAddress()}.
*
* @param local the local host and port address
* @return this
*/
RequestFixture localAddress(HostAndPort local);
}