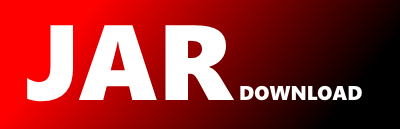
io.reacted.patterns.UnChecked Maven / Gradle / Ivy
/*
* Copyright (c) 2020 , [ [email protected] ]
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree.
*/
package io.reacted.patterns;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.BinaryOperator;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.function.UnaryOperator;
public final class UnChecked {
private UnChecked() { }
public static Supplier supplier(CheckedSupplier checkedSupplier) {
return unchecker(checkedSupplier);
}
@SuppressWarnings("ResultOfMethodCallIgnored")
public static Consumer consumer(CheckedConsumer checkedConsumer) {
return arg -> unchecker(() -> {
checkedConsumer.accept(arg);
return Try.VOID;
});
}
@SuppressWarnings("ReturnValueIgnored")
public static BiConsumer biConsumer(CheckedBiConsumer checkedBiConsumer) {
return (arg1, arg2) -> unchecker(() -> { checkedBiConsumer.accept(arg1, arg2); return Try.VOID; }).get();
}
public static Function function(CheckedFunction checkedFunction) {
return arg -> unchecker(() -> checkedFunction.apply(arg)).get();
}
public static BiFunction biFunction(CheckedBiFunction checkedBifunction) {
return (arg1, arg2) -> unchecker(() -> checkedBifunction.apply(arg1, arg2)).get();
}
public static UnaryOperator unaryOperator(CheckedUnaryOperator checkedUnaryOperator) {
return arg1 -> unchecker(() -> checkedUnaryOperator.apply(arg1)).get();
}
public static BinaryOperator binaryOperator(CheckedBinaryOperator checkedBinaryOperator) {
return (arg1, arg2) -> unchecker(() -> checkedBinaryOperator.apply(arg1, arg2)).get();
}
public static Predicate predicate(CheckedPredicate checkedPredicate) {
return arg -> unchecker(() -> checkedPredicate.test(arg)).get();
}
@SuppressWarnings("EmptyMethod")
@FunctionalInterface
public interface CheckedSupplier {
T get() throws Throwable;
}
@FunctionalInterface
public interface CheckedConsumer {
void accept(T arg1) throws Throwable;
}
@FunctionalInterface
public interface CheckedBiConsumer {
void accept(T arg1, U arg2) throws Throwable;
}
@FunctionalInterface
public interface CheckedFunction {
U apply(T arg1) throws Throwable;
}
@FunctionalInterface
public interface CheckedBiFunction {
R apply(T arg1, U arg2) throws Throwable;
}
@FunctionalInterface
public interface CheckedUnaryOperator extends CheckedFunction {
static CheckedUnaryOperator identity() {
return t -> t;
}
}
@FunctionalInterface
public interface CheckedBinaryOperator extends CheckedBiFunction { }
@FunctionalInterface
public interface CheckedPredicate {
boolean test(T arg) throws Throwable;
}
@FunctionalInterface
public interface CheckedRunnable {
void run() throws Throwable;
}
@FunctionalInterface
public interface CheckedCallable {
T call() throws Throwable;
}
private static Supplier unchecker(CheckedSupplier resultSupplier) {
return () -> Try.of(resultSupplier::get)
.orElseRecover(UnChecked::sneakyThrow);
}
@SuppressWarnings("unchecked")
public static U sneakyThrow(Throwable t) throws T {
throw (T) t;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy