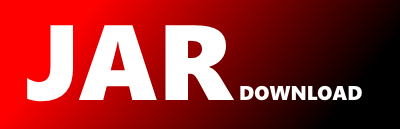
io.reactivex.rxjava3.core.Observable Maven / Gradle / Ivy
Show all versions of rxjava Show documentation
/*
* Copyright (c) 2016-present, RxJava Contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package io.reactivex.rxjava3.core;
import java.util.*;
import java.util.concurrent.*;
import java.util.stream.*;
import org.reactivestreams.Publisher;
import io.reactivex.rxjava3.annotations.*;
import io.reactivex.rxjava3.disposables.*;
import io.reactivex.rxjava3.exceptions.*;
import io.reactivex.rxjava3.functions.*;
import io.reactivex.rxjava3.internal.functions.*;
import io.reactivex.rxjava3.internal.jdk8.*;
import io.reactivex.rxjava3.internal.observers.*;
import io.reactivex.rxjava3.internal.operators.flowable.*;
import io.reactivex.rxjava3.internal.operators.maybe.MaybeToObservable;
import io.reactivex.rxjava3.internal.operators.mixed.*;
import io.reactivex.rxjava3.internal.operators.observable.*;
import io.reactivex.rxjava3.internal.operators.single.SingleToObservable;
import io.reactivex.rxjava3.internal.util.*;
import io.reactivex.rxjava3.observables.*;
import io.reactivex.rxjava3.observers.*;
import io.reactivex.rxjava3.operators.ScalarSupplier;
import io.reactivex.rxjava3.plugins.RxJavaPlugins;
import io.reactivex.rxjava3.schedulers.*;
/**
* The {@code Observable} class is the non-backpressured, optionally multi-valued base reactive class that
* offers factory methods, intermediate operators and the ability to consume synchronous
* and/or asynchronous reactive dataflows.
*
* Many operators in the class accept {@link ObservableSource}(s), the base reactive interface
* for such non-backpressured flows, which {@code Observable} itself implements as well.
*
* The {@code Observable}'s operators, by default, run with a buffer size of 128 elements (see {@link Flowable#bufferSize()}),
* that can be overridden globally via the system parameter {@code rx3.buffer-size}. Most operators, however, have
* overloads that allow setting their internal buffer size explicitly.
*
* The documentation for this class makes use of marble diagrams. The following legend explains these diagrams:
*
*
*
* The design of this class was derived from the
* Reactive-Streams design and specification
* by removing any backpressure-related infrastructure and implementation detail, replacing the
* {@code org.reactivestreams.Subscription} with {@link Disposable} as the primary means to dispose of
* a flow.
*
* The {@code Observable} follows the protocol
*
* onSubscribe onNext* (onError | onComplete)?
*
* where
* the stream can be disposed through the {@code Disposable} instance provided to consumers through
* {@code Observer.onSubscribe}.
*
* Unlike the {@code Observable} of version 1.x, {@link #subscribe(Observer)} does not allow external disposal
* of a subscription and the {@link Observer} instance is expected to expose such capability.
*
Example:
*
* Disposable d = Observable.just("Hello world!")
* .delay(1, TimeUnit.SECONDS)
* .subscribeWith(new DisposableObserver<String>() {
* @Override public void onStart() {
* System.out.println("Start!");
* }
* @Override public void onNext(String t) {
* System.out.println(t);
* }
* @Override public void onError(Throwable t) {
* t.printStackTrace();
* }
* @Override public void onComplete() {
* System.out.println("Done!");
* }
* });
*
* Thread.sleep(500);
* // the sequence can now be disposed via dispose()
* d.dispose();
*
*
* @param
* the type of the items emitted by the {@code Observable}
* @see Flowable
* @see io.reactivex.rxjava3.observers.DisposableObserver
*/
public abstract class Observable<@NonNull T> implements ObservableSource {
/**
* Mirrors the one {@link ObservableSource} in an {@link Iterable} of several {@code ObservableSource}s that first either emits an item or sends
* a termination notification.
*
*
*
* When one of the {@code ObservableSource}s signal an item or terminates first, all subscriptions to the other
* {@code ObservableSource}s are disposed.
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* -
* If any of the losing {@code ObservableSource}s signals an error, the error is routed to the global
* error handler via {@link RxJavaPlugins#onError(Throwable)}.
*
*
*
* @param the common element type
* @param sources
* an {@code Iterable} of {@code ObservableSource} sources competing to react first. A subscription to each source will
* occur in the same order as in the {@code Iterable}.
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Amb
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable amb(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources) {
Objects.requireNonNull(sources, "sources is null");
return RxJavaPlugins.onAssembly(new ObservableAmb<>(null, sources));
}
/**
* Mirrors the one {@link ObservableSource} in an array of several {@code ObservableSource}s that first either emits an item or sends
* a termination notification.
*
*
*
* When one of the {@code ObservableSource}s signal an item or terminates first, all subscriptions to the other
* {@code ObservableSource}s are disposed.
*
* - Scheduler:
* - {@code ambArray} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* -
* If any of the losing {@code ObservableSource}s signals an error, the error is routed to the global
* error handler via {@link RxJavaPlugins#onError(Throwable)}.
*
*
*
* @param the common element type
* @param sources
* an array of {@code ObservableSource} sources competing to react first. A subscription to each source will
* occur in the same order as in the array.
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Amb
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
public static <@NonNull T> Observable ambArray(@NonNull ObservableSource extends T>... sources) {
Objects.requireNonNull(sources, "sources is null");
int len = sources.length;
if (len == 0) {
return empty();
}
if (len == 1) {
return (Observable)wrap(sources[0]);
}
return RxJavaPlugins.onAssembly(new ObservableAmb<>(sources, null));
}
/**
* Returns the default 'island' size or capacity-increment hint for unbounded buffers.
* Delegates to {@link Flowable#bufferSize} but is public for convenience.
*
The value can be overridden via system parameter {@code rx3.buffer-size}
* before the {@link Flowable} class is loaded.
* @return the default 'island' size or capacity-increment hint
*/
@CheckReturnValue
public static int bufferSize() {
return Flowable.bufferSize();
}
/**
* Combines a collection of source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the returned {@code ObservableSource}s each time an item is received from any of the returned {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided iterable of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the returned {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T, @NonNull R> Observable combineLatest(
@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner) {
return combineLatest(sources, combiner, bufferSize());
}
/**
* Combines an {@link Iterable} of source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the returned {@code ObservableSource}s each time an item is received from any of the returned {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided {@code Iterable} of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the returned {@code ObservableSource}s
* @param bufferSize
* the expected number of row combination items to be buffered internally
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull R> Observable combineLatest(
@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
// the queue holds a pair of values so we need to double the capacity
int s = bufferSize << 1;
return RxJavaPlugins.onAssembly(new ObservableCombineLatest<>(null, sources, combiner, s, false));
}
/**
* Combines an array of source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the {@code ObservableSource}s each time an item is received from any of the returned {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
*
*
* - Scheduler:
* - {@code combineLatestArray} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T, @NonNull R> Observable combineLatestArray(
@NonNull ObservableSource extends T>[] sources,
@NonNull Function super Object[], ? extends R> combiner) {
return combineLatestArray(sources, combiner, bufferSize());
}
/**
* Combines an array of source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
*
*
* - Scheduler:
* - {@code combineLatestArray} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @param bufferSize
* the expected number of row combination items to be buffered internally
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull R> Observable combineLatestArray(
@NonNull ObservableSource extends T>[] sources,
@NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
if (sources.length == 0) {
return empty();
}
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
// the queue holds a pair of values so we need to double the capacity
int s = bufferSize << 1;
return RxJavaPlugins.onAssembly(new ObservableCombineLatest<>(sources, null, combiner, s, false));
}
/**
* Combines two source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from either of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull BiFunction super T1, ? super T2, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines three source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param source3
* the third source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull ObservableSource extends T3> source3,
@NonNull Function3 super T1, ? super T2, ? super T3, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2, source3 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines four source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param source3
* the third source {@code ObservableSource}
* @param source4
* the fourth source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull ObservableSource extends T3> source3, @NonNull ObservableSource extends T4> source4,
@NonNull Function4 super T1, ? super T2, ? super T3, ? super T4, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2, source3, source4 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines five source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param source3
* the third source {@code ObservableSource}
* @param source4
* the fourth source {@code ObservableSource}
* @param source5
* the fifth source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull ObservableSource extends T3> source3, @NonNull ObservableSource extends T4> source4,
@NonNull ObservableSource extends T5> source5,
@NonNull Function5 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2, source3, source4, source5 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines six source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param source3
* the third source {@code ObservableSource}
* @param source4
* the fourth source {@code ObservableSource}
* @param source5
* the fifth source {@code ObservableSource}
* @param source6
* the sixth source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull ObservableSource extends T3> source3, @NonNull ObservableSource extends T4> source4,
@NonNull ObservableSource extends T5> source5, @NonNull ObservableSource extends T6> source6,
@NonNull Function6 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2, source3, source4, source5, source6 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines seven source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the element type of the seventh source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param source3
* the third source {@code ObservableSource}
* @param source4
* the fourth source {@code ObservableSource}
* @param source5
* the fifth source {@code ObservableSource}
* @param source6
* the sixth source {@code ObservableSource}
* @param source7
* the seventh source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6},
* {@code source7} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull T7, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull ObservableSource extends T3> source3, @NonNull ObservableSource extends T4> source4,
@NonNull ObservableSource extends T5> source5, @NonNull ObservableSource extends T6> source6,
@NonNull ObservableSource extends T7> source7,
@NonNull Function7 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(source7, "source7 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2, source3, source4, source5, source6, source7 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines eight source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the element type of the seventh source
* @param the element type of the eighth source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param source3
* the third source {@code ObservableSource}
* @param source4
* the fourth source {@code ObservableSource}
* @param source5
* the fifth source {@code ObservableSource}
* @param source6
* the sixth source {@code ObservableSource}
* @param source7
* the seventh source {@code ObservableSource}
* @param source8
* the eighth source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6},
* {@code source7}, {@code source8} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull T7, @NonNull T8, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull ObservableSource extends T3> source3, @NonNull ObservableSource extends T4> source4,
@NonNull ObservableSource extends T5> source5, @NonNull ObservableSource extends T6> source6,
@NonNull ObservableSource extends T7> source7, @NonNull ObservableSource extends T8> source8,
@NonNull Function8 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(source7, "source7 is null");
Objects.requireNonNull(source8, "source8 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2, source3, source4, source5, source6, source7, source8 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines nine source {@link ObservableSource}s by emitting an item that aggregates the latest values of each of the
* {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the element type of the seventh source
* @param the element type of the eighth source
* @param the element type of the ninth source
* @param the combined output type
* @param source1
* the first source {@code ObservableSource}
* @param source2
* the second source {@code ObservableSource}
* @param source3
* the third source {@code ObservableSource}
* @param source4
* the fourth source {@code ObservableSource}
* @param source5
* the fifth source {@code ObservableSource}
* @param source6
* the sixth source {@code ObservableSource}
* @param source7
* the seventh source {@code ObservableSource}
* @param source8
* the eighth source {@code ObservableSource}
* @param source9
* the ninth source {@code ObservableSource}
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6},
* {@code source7}, {@code source8}, {@code source9} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull T7, @NonNull T8, @NonNull T9, @NonNull R> Observable combineLatest(
@NonNull ObservableSource extends T1> source1, @NonNull ObservableSource extends T2> source2,
@NonNull ObservableSource extends T3> source3, @NonNull ObservableSource extends T4> source4,
@NonNull ObservableSource extends T5> source5, @NonNull ObservableSource extends T6> source6,
@NonNull ObservableSource extends T7> source7, @NonNull ObservableSource extends T8> source8,
@NonNull ObservableSource extends T9> source9,
@NonNull Function9 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(source7, "source7 is null");
Objects.requireNonNull(source8, "source8 is null");
Objects.requireNonNull(source9, "source9 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new ObservableSource[] { source1, source2, source3, source4, source5, source6, source7, source8, source9 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines an array of {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function.
*
*
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Scheduler:
* - {@code combineLatestArrayDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T, @NonNull R> Observable combineLatestArrayDelayError(
@NonNull ObservableSource extends T>[] sources,
@NonNull Function super Object[], ? extends R> combiner) {
return combineLatestArrayDelayError(sources, combiner, bufferSize());
}
/**
* Combines an array of {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function and delays any error from the sources until
* all source {@code ObservableSource}s terminate.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
*
*
* - Scheduler:
* - {@code combineLatestArrayDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @param bufferSize
* the expected number of row combination items to be buffered internally
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull R> Observable combineLatestArrayDelayError(@NonNull ObservableSource extends T>[] sources,
@NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
if (sources.length == 0) {
return empty();
}
// the queue holds a pair of values so we need to double the capacity
int s = bufferSize << 1;
return RxJavaPlugins.onAssembly(new ObservableCombineLatest<>(sources, null, combiner, s, true));
}
/**
* Combines an {@link Iterable} of {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function and delays any error from the sources until
* all source {@code ObservableSource}s terminate.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided iterable of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
*
*
* - Scheduler:
* - {@code combineLatestDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the {@code Iterable} of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T, @NonNull R> Observable combineLatestDelayError(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner) {
return combineLatestDelayError(sources, combiner, bufferSize());
}
/**
* Combines an {@link Iterable} of {@link ObservableSource}s by emitting an item that aggregates the latest values of each of
* the {@code ObservableSource}s each time an item is received from any of the {@code ObservableSource}s, where this
* aggregation is defined by a specified function and delays any error from the sources until
* all source {@code ObservableSource}s terminate.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated till that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided iterable of {@code ObservableSource}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
*
*
* - Scheduler:
* - {@code combineLatestDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code ObservableSource}s
* @param combiner
* the aggregation function used to combine the items emitted by the {@code ObservableSource}s
* @param bufferSize
* the expected number of row combination items to be buffered internally
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull R> Observable combineLatestDelayError(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
// the queue holds a pair of values so we need to double the capacity
int s = bufferSize << 1;
return RxJavaPlugins.onAssembly(new ObservableCombineLatest<>(null, sources, combiner, s, true));
}
/**
* Concatenates elements of each {@link ObservableSource} provided via an {@link Iterable} sequence into a single sequence
* of elements without interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
* @param the common value type of the sources
* @param sources the {@code Iterable} sequence of {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable concat(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources) {
Objects.requireNonNull(sources, "sources is null");
return fromIterable(sources).concatMapDelayError((Function)Functions.identity(), false, bufferSize());
}
/**
* Returns an {@code Observable} that emits the items emitted by each of the {@link ObservableSource}s emitted by the
* {@code ObservableSource}, one after the other, without interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources
* an {@code ObservableSource} that emits {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concat(@NonNull ObservableSource extends ObservableSource extends T>> sources) {
return concat(sources, bufferSize());
}
/**
* Returns an {@code Observable} that emits the items emitted by each of the {@link ObservableSource}s emitted by the outer
* {@code ObservableSource}, one after the other, without interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources
* an {@code ObservableSource} that emits {@code ObservableSource}s
* @param bufferSize
* the number of inner {@code ObservableSource}s expected to be buffered.
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: Concat
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable concat(@NonNull ObservableSource extends ObservableSource extends T>> sources, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
return RxJavaPlugins.onAssembly(new ObservableConcatMap(sources, Functions.identity(), bufferSize, ErrorMode.IMMEDIATE));
}
/**
* Returns an {@code Observable} that emits the items emitted by two {@link ObservableSource}s, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param source1
* an {@code ObservableSource} to be concatenated
* @param source2
* an {@code ObservableSource} to be concatenated
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1} or {@code source2} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable concat(@NonNull ObservableSource extends T> source1, ObservableSource extends T> source2) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
return concatArray(source1, source2);
}
/**
* Returns an {@code Observable} that emits the items emitted by three {@link ObservableSource}s, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param source1
* an {@code ObservableSource} to be concatenated
* @param source2
* an {@code ObservableSource} to be concatenated
* @param source3
* an {@code ObservableSource} to be concatenated
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2} or {@code source3} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable concat(
@NonNull ObservableSource extends T> source1, @NonNull ObservableSource extends T> source2,
@NonNull ObservableSource extends T> source3) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
return concatArray(source1, source2, source3);
}
/**
* Returns an {@code Observable} that emits the items emitted by four {@link ObservableSource}s, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param source1
* an {@code ObservableSource} to be concatenated
* @param source2
* an {@code ObservableSource} to be concatenated
* @param source3
* an {@code ObservableSource} to be concatenated
* @param source4
* an {@code ObservableSource} to be concatenated
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3} or {@code source4} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable concat(
@NonNull ObservableSource extends T> source1, @NonNull ObservableSource extends T> source2,
@NonNull ObservableSource extends T> source3, @NonNull ObservableSource extends T> source4) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
return concatArray(source1, source2, source3, source4);
}
/**
* Concatenates a variable number of {@link ObservableSource} sources.
*
* Note: named this way because of overload conflict with {@code concat(ObservableSource)}
*
*
*
* - Scheduler:
* - {@code concatArray} does not operate by default on a particular {@link Scheduler}.
*
* @param sources the array of sources
* @param the common base value type
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
@SafeVarargs
public static <@NonNull T> Observable concatArray(@NonNull ObservableSource extends T>... sources) {
Objects.requireNonNull(sources, "sources is null");
if (sources.length == 0) {
return empty();
}
if (sources.length == 1) {
return wrap((ObservableSource)sources[0]);
}
return RxJavaPlugins.onAssembly(new ObservableConcatMap(fromArray(sources), Functions.identity(), bufferSize(), ErrorMode.BOUNDARY));
}
/**
* Concatenates a variable number of {@link ObservableSource} sources and delays errors from any of them
* till all terminate.
*
*
*
* - Scheduler:
* - {@code concatArrayDelayError} does not operate by default on a particular {@link Scheduler}.
*
* @param sources the array of sources
* @param the common base value type
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
@SafeVarargs
public static <@NonNull T> Observable concatArrayDelayError(@NonNull ObservableSource extends T>... sources) {
Objects.requireNonNull(sources, "sources is null");
if (sources.length == 0) {
return empty();
}
if (sources.length == 1) {
@SuppressWarnings("unchecked")
Observable source = (Observable)wrap(sources[0]);
return source;
}
return concatDelayError(fromArray(sources));
}
/**
* Concatenates an array of {@link ObservableSource}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s and then drains them
* in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code ObservableSource}s that need to be eagerly concatenated
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.0
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
@NonNull
public static <@NonNull T> Observable concatArrayEager(@NonNull ObservableSource extends T>... sources) {
return concatArrayEager(bufferSize(), bufferSize(), sources);
}
/**
* Concatenates an array of {@link ObservableSource}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s and then drains them
* in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code ObservableSource}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrent subscriptions at a time, {@link Integer#MAX_VALUE}
* is interpreted as indication to subscribe to all sources at once
* @param bufferSize the number of elements expected from each {@code ObservableSource} to be buffered
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @since 2.0
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
@SafeVarargs
public static <@NonNull T> Observable concatArrayEager(int maxConcurrency, int bufferSize, @NonNull ObservableSource extends T>... sources) {
return fromArray(sources).concatMapEagerDelayError((Function)Functions.identity(), false, maxConcurrency, bufferSize);
}
/**
* Concatenates an array of {@link ObservableSource}s eagerly into a single stream of values
* and delaying any errors until all sources terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s
* and then drains them in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code ObservableSource}s that need to be eagerly concatenated
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.2.1 - experimental
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
@NonNull
public static <@NonNull T> Observable concatArrayEagerDelayError(@NonNull ObservableSource extends T>... sources) {
return concatArrayEagerDelayError(bufferSize(), bufferSize(), sources);
}
/**
* Concatenates an array of {@link ObservableSource}s eagerly into a single stream of values
* and delaying any errors until all sources terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s
* and then drains them in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code ObservableSource}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrent subscriptions at a time, {@link Integer#MAX_VALUE}
* is interpreted as indication to subscribe to all sources at once
* @param bufferSize the number of elements expected from each {@code ObservableSource} to be buffered
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @since 2.2.1 - experimental
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
@SafeVarargs
public static <@NonNull T> Observable concatArrayEagerDelayError(int maxConcurrency, int bufferSize, @NonNull ObservableSource extends T>... sources) {
return fromArray(sources).concatMapEagerDelayError((Function)Functions.identity(), true, maxConcurrency, bufferSize);
}
/**
* Concatenates the {@link Iterable} sequence of {@link ObservableSource}s into a single {@code Observable} sequence
* by subscribing to each {@code ObservableSource}, one after the other, one at a time and delays any errors till
* the all inner {@code ObservableSource}s terminate.
*
*
*
* - Scheduler:
* - {@code concatDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources the {@code Iterable} sequence of {@code ObservableSource}s
* @return the new {@code Observable} with the concatenating behavior
* @throws NullPointerException if {@code sources} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable concatDelayError(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources) {
Objects.requireNonNull(sources, "sources is null");
return concatDelayError(fromIterable(sources));
}
/**
* Concatenates the {@link ObservableSource} sequence of {@code ObservableSource}s into a single {@code Observable} sequence
* by subscribing to each inner {@code ObservableSource}, one after the other, one at a time and delays any errors till the
* all inner and the outer {@code ObservableSource}s terminate.
*
*
*
* - Scheduler:
* - {@code concatDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources the {@code ObservableSource} sequence of {@code ObservableSource}s
* @return the new {@code Observable} with the concatenating behavior
* @throws NullPointerException if {@code sources} is {@code null}
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatDelayError(@NonNull ObservableSource extends ObservableSource extends T>> sources) {
return concatDelayError(sources, bufferSize(), true);
}
/**
* Concatenates the {@link ObservableSource} sequence of {@code ObservableSource}s into a single sequence by subscribing to each inner {@code ObservableSource},
* one after the other, one at a time and delays any errors till the all inner and the outer {@code ObservableSource}s terminate.
*
*
*
* - Scheduler:
* - {@code concatDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources the {@code ObservableSource} sequence of {@code ObservableSource}s
* @param bufferSize the number of inner {@code ObservableSource}s expected to be buffered
* @param tillTheEnd if {@code true}, exceptions from the outer and all inner {@code ObservableSource}s are delayed to the end
* if {@code false}, exception from the outer {@code ObservableSource} is delayed till the active {@code ObservableSource} terminates
* @return the new {@code Observable} with the concatenating behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable concatDelayError(@NonNull ObservableSource extends ObservableSource extends T>> sources, int bufferSize, boolean tillTheEnd) {
Objects.requireNonNull(sources, "sources is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize is null");
return RxJavaPlugins.onAssembly(new ObservableConcatMap(sources, Functions.identity(), bufferSize, tillTheEnd ? ErrorMode.END : ErrorMode.BOUNDARY));
}
/**
* Concatenates a sequence of {@link ObservableSource}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s and then drains them
* in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.0
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEager(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources) {
return concatEager(sources, bufferSize(), bufferSize());
}
/**
* Concatenates a sequence of {@link ObservableSource}s eagerly into a single stream of values and
* runs a limited number of inner sequences at once.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s and then drains them
* in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code ObservableSource}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code ObservableSource}s can be active at the same time
* @param bufferSize the number of elements expected from each inner {@code ObservableSource} to be buffered
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @since 2.0
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEager(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources, int maxConcurrency, int bufferSize) {
return fromIterable(sources).concatMapEagerDelayError((Function)Functions.identity(), false, maxConcurrency, bufferSize);
}
/**
* Concatenates an {@link ObservableSource} sequence of {@code ObservableSource}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code ObservableSource}s as they are observed. The operator buffers the values emitted by these
* {@code ObservableSource}s and then drains them in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.0
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEager(@NonNull ObservableSource extends ObservableSource extends T>> sources) {
return concatEager(sources, bufferSize(), bufferSize());
}
/**
* Concatenates an {@link ObservableSource} sequence of {@code ObservableSource}s eagerly into a single stream of values
* and runs a limited number of inner sequences at once.
*
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code ObservableSource}s as they are observed. The operator buffers the values emitted by these
* {@code ObservableSource}s and then drains them in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code ObservableSource}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code ObservableSource}s can be active at the same time
* @param bufferSize the number of inner {@code ObservableSource} expected to be buffered
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @since 2.0
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEager(@NonNull ObservableSource extends ObservableSource extends T>> sources, int maxConcurrency, int bufferSize) {
return wrap(sources).concatMapEager((Function)Functions.identity(), maxConcurrency, bufferSize);
}
/**
* Concatenates a sequence of {@link ObservableSource}s eagerly into a single stream of values,
* delaying errors until all the inner sequences terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s and then drains them
* in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEagerDelayError(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources) {
return concatEagerDelayError(sources, bufferSize(), bufferSize());
}
/**
* Concatenates a sequence of {@link ObservableSource}s eagerly into a single stream of values,
* delaying errors until all the inner sequences terminate and runs a limited number of inner
* sequences at once.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* {@code ObservableSource}s. The operator buffers the values emitted by these {@code ObservableSource}s and then drains them
* in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code ObservableSource}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code ObservableSource}s can be active at the same time
* @param bufferSize the number of elements expected from each inner {@code ObservableSource} to be buffered
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @since 3.0.0
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEagerDelayError(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources, int maxConcurrency, int bufferSize) {
return fromIterable(sources).concatMapEagerDelayError((Function)Functions.identity(), true, maxConcurrency, bufferSize);
}
/**
* Concatenates an {@link ObservableSource} sequence of {@code ObservableSource}s eagerly into a single stream of values,
* delaying errors until all the inner and the outer sequence terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code ObservableSource}s as they are observed. The operator buffers the values emitted by these
* {@code ObservableSource}s and then drains them in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEagerDelayError(@NonNull ObservableSource extends ObservableSource extends T>> sources) {
return concatEagerDelayError(sources, bufferSize(), bufferSize());
}
/**
* Concatenates an {@link ObservableSource} sequence of {@code ObservableSource}s eagerly into a single stream of values,
* delaying errors until all the inner and the outer sequence terminate and runs a limited number of inner sequences at once.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code ObservableSource}s as they are observed. The operator buffers the values emitted by these
* {@code ObservableSource}s and then drains them in order, each one after the previous one completes.
*
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code ObservableSource}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code ObservableSource}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code ObservableSource}s can be active at the same time
* @param bufferSize the number of inner {@code ObservableSource} expected to be buffered
* @return the new {@code Observable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @since 3.0.0
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable concatEagerDelayError(@NonNull ObservableSource extends ObservableSource extends T>> sources, int maxConcurrency, int bufferSize) {
return wrap(sources).concatMapEagerDelayError((Function)Functions.identity(), true, maxConcurrency, bufferSize);
}
/**
* Provides an API (via a cold {@code Observable}) that bridges the reactive world with the callback-style world.
*
* Example:
*
* Observable.<Event>create(emitter -> {
* Callback listener = new Callback() {
* @Override
* public void onEvent(Event e) {
* emitter.onNext(e);
* if (e.isLast()) {
* emitter.onComplete();
* }
* }
*
* @Override
* public void onFailure(Exception e) {
* emitter.onError(e);
* }
* };
*
* AutoCloseable c = api.someMethod(listener);
*
* emitter.setCancellable(c::close);
*
* });
*
*
* Whenever an {@link Observer} subscribes to the returned {@code Observable}, the provided
* {@link ObservableOnSubscribe} callback is invoked with a fresh instance of an {@link ObservableEmitter}
* that will interact only with that specific {@code Observer}. If this {@code Observer}
* disposes the flow (making {@link ObservableEmitter#isDisposed} return {@code true}),
* other observers subscribed to the same returned {@code Observable} are not affected.
*
*
*
* You should call the {@code ObservableEmitter}'s {@code onNext}, {@code onError} and {@code onComplete} methods in a serialized fashion. The
* rest of its methods are thread-safe.
*
* - Scheduler:
* - {@code create} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type
* @param source the emitter that is called when an {@code Observer} subscribes to the returned {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source} is {@code null}
* @see ObservableOnSubscribe
* @see ObservableEmitter
* @see Cancellable
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable create(@NonNull ObservableOnSubscribe source) {
Objects.requireNonNull(source, "source is null");
return RxJavaPlugins.onAssembly(new ObservableCreate<>(source));
}
/**
* Returns an {@code Observable} that calls an {@link ObservableSource} factory to create an {@code ObservableSource} for each new {@link Observer}
* that subscribes. That is, for each subscriber, the actual {@code ObservableSource} that subscriber observes is
* determined by the factory function.
*
*
*
* The {@code defer} operator allows you to defer or delay emitting items from an {@code ObservableSource} until such time as an
* {@code Observer} subscribes to the {@code ObservableSource}. This allows an {@code Observer} to easily obtain updates or a
* refreshed version of the sequence.
*
* - Scheduler:
* - {@code defer} does not operate by default on a particular {@link Scheduler}.
*
*
* @param supplier
* the {@code ObservableSource} factory function to invoke for each {@code Observer} that subscribes to the
* resulting {@code Observable}
* @param
* the type of the items emitted by the {@code ObservableSource}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code supplier} is {@code null}
* @see ReactiveX operators documentation: Defer
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable defer(@NonNull Supplier extends ObservableSource extends T>> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return RxJavaPlugins.onAssembly(new ObservableDefer<>(supplier));
}
/**
* Returns an {@code Observable} that emits no items to the {@link Observer} and immediately invokes its
* {@link Observer#onComplete onComplete} method.
*
*
*
* - Scheduler:
* - {@code empty} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the type of the items (ostensibly) emitted by the {@code Observable}
* @return the shared {@code Observable} instance
* @see ReactiveX operators documentation: Empty
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings("unchecked")
@NonNull
public static <@NonNull T> Observable empty() {
return RxJavaPlugins.onAssembly((Observable) ObservableEmpty.INSTANCE);
}
/**
* Returns an {@code Observable} that invokes an {@link Observer}'s {@link Observer#onError onError} method when the
* {@code Observer} subscribes to it.
*
*
*
* - Scheduler:
* - {@code error} does not operate by default on a particular {@link Scheduler}.
*
*
* @param supplier
* a {@link Supplier} factory to return a {@link Throwable} for each individual {@code Observer}
* @param
* the type of the items (ostensibly) emitted by the {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code supplier} is {@code null}
* @see ReactiveX operators documentation: Throw
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable error(@NonNull Supplier extends Throwable> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return RxJavaPlugins.onAssembly(new ObservableError<>(supplier));
}
/**
* Returns an {@code Observable} that invokes an {@link Observer}'s {@link Observer#onError onError} method when the
* {@code Observer} subscribes to it.
*
*
*
* - Scheduler:
* - {@code error} does not operate by default on a particular {@link Scheduler}.
*
*
* @param throwable
* the particular {@link Throwable} to pass to {@link Observer#onError onError}
* @param
* the type of the items (ostensibly) emitted by the {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code throwable} is {@code null}
* @see ReactiveX operators documentation: Throw
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable error(@NonNull Throwable throwable) {
Objects.requireNonNull(throwable, "throwable is null");
return error(Functions.justSupplier(throwable));
}
/**
* Returns an {@code Observable} instance that runs the given {@link Action} for each {@link Observer} and
* emits either its exception or simply completes.
*
*
*
* - Scheduler:
* - {@code fromAction} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@code Action} throws an exception, the respective {@link Throwable} is
* delivered to the downstream via {@link Observer#onError(Throwable)},
* except when the downstream has canceled the resulting {@code Observable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link io.reactivex.rxjava3.exceptions.UndeliverableException UndeliverableException}.
*
*
* @param the target type
* @param action the {@code Action} to run for each {@code Observer}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code action} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromAction(@NonNull Action action) {
Objects.requireNonNull(action, "action is null");
return RxJavaPlugins.onAssembly(new ObservableFromAction<>(action));
}
/**
* Converts an array into an {@link ObservableSource} that emits the items in the array.
*
*
*
* - Scheduler:
* - {@code fromArray} does not operate by default on a particular {@link Scheduler}.
*
*
* @param items
* the array of elements
* @param
* the type of items in the array and the type of items to be emitted by the resulting {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code items} is {@code null}
* @see ReactiveX operators documentation: From
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
@SafeVarargs
public static <@NonNull T> Observable fromArray(@NonNull T... items) {
Objects.requireNonNull(items, "items is null");
if (items.length == 0) {
return empty();
}
if (items.length == 1) {
return just(items[0]);
}
return RxJavaPlugins.onAssembly(new ObservableFromArray<>(items));
}
/**
* Returns an {@code Observable} that, when an observer subscribes to it, invokes a function you specify and then
* emits the value returned from that function.
*
*
*
* This allows you to defer the execution of the function you specify until an observer subscribes to the
* {@code Observable}. That is to say, it makes the function "lazy."
*
* - Scheduler:
* - {@code fromCallable} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@link Callable} throws an exception, the respective {@link Throwable} is
* delivered to the downstream via {@link Observer#onError(Throwable)},
* except when the downstream has disposed the current {@code Observable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link UndeliverableException}.
*
*
* @param callable
* a function, the execution of which should be deferred; {@code fromCallable} will invoke this
* function only when an observer subscribes to the {@code Observable} that {@code fromCallable} returns
* @param
* the type of the item returned by the {@code Callable} and emitted by the {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code callable} is {@code null}
* @see #defer(Supplier)
* @see #fromSupplier(Supplier)
* @since 2.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromCallable(@NonNull Callable extends T> callable) {
Objects.requireNonNull(callable, "callable is null");
return RxJavaPlugins.onAssembly(new ObservableFromCallable<>(callable));
}
/**
* Wraps a {@link CompletableSource} into an {@code Observable}.
*
*
*
* - Scheduler:
* - {@code fromCompletable} does not operate by default on a particular {@link Scheduler}.
*
* @param the target type
* @param completableSource the {@code CompletableSource} to convert from
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code completableSource} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromCompletable(@NonNull CompletableSource completableSource) {
Objects.requireNonNull(completableSource, "completableSource is null");
return RxJavaPlugins.onAssembly(new ObservableFromCompletable<>(completableSource));
}
/**
* Converts a {@link Future} into an {@code Observable}.
*
*
*
* The operator calls {@link Future#get()}, which is a blocking method, on the subscription thread.
* It is recommended applying {@link #subscribeOn(Scheduler)} to move this blocking wait to a
* background thread, and if the {@link Scheduler} supports it, interrupt the wait when the flow
* is disposed.
*
* Unlike 1.x, disposing the {@code Observable} won't cancel the future. If necessary, one can use composition to achieve the
* cancellation effect: {@code futureObservableSource.doOnDispose(() -> future.cancel(true));}.
*
* Also note that this operator will consume a {@link CompletionStage}-based {@code Future} subclass (such as
* {@link CompletableFuture}) in a blocking manner as well. Use the {@link #fromCompletionStage(CompletionStage)}
* operator to convert and consume such sources in a non-blocking fashion instead.
*
* - Scheduler:
* - {@code fromFuture} does not operate by default on a particular {@code Scheduler}.
*
*
* @param future
* the source {@code Future}
* @param
* the type of object that the {@code Future} returns, and also the type of item to be emitted by
* the resulting {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code future} is {@code null}
* @see ReactiveX operators documentation: From
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromFuture(@NonNull Future extends T> future) {
Objects.requireNonNull(future, "future is null");
return RxJavaPlugins.onAssembly(new ObservableFromFuture<>(future, 0L, null));
}
/**
* Converts a {@link Future} into an {@code Observable}, with a timeout on the {@code Future}.
*
*
*
* The operator calls {@link Future#get(long, TimeUnit)}, which is a blocking method, on the subscription thread.
* It is recommended applying {@link #subscribeOn(Scheduler)} to move this blocking wait to a
* background thread, and if the {@link Scheduler} supports it, interrupt the wait when the flow
* is disposed.
*
* Unlike 1.x, disposing the {@code Observable} won't cancel the future. If necessary, one can use composition to achieve the
* cancellation effect: {@code futureObservableSource.doOnDispose(() -> future.cancel(true));}.
*
* Also note that this operator will consume a {@link CompletionStage}-based {@code Future} subclass (such as
* {@link CompletableFuture}) in a blocking manner as well. Use the {@link #fromCompletionStage(CompletionStage)}
* operator to convert and consume such sources in a non-blocking fashion instead.
*
* - Scheduler:
* - {@code fromFuture} does not operate by default on a particular {@code Scheduler}.
*
*
* @param future
* the source {@code Future}
* @param timeout
* the maximum time to wait before calling {@code get}
* @param unit
* the {@link TimeUnit} of the {@code timeout} argument
* @param
* the type of object that the {@code Future} returns, and also the type of item to be emitted by
* the resulting {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code future} or {@code unit} is {@code null}
* @see ReactiveX operators documentation: From
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromFuture(@NonNull Future extends T> future, long timeout, @NonNull TimeUnit unit) {
Objects.requireNonNull(future, "future is null");
Objects.requireNonNull(unit, "unit is null");
return RxJavaPlugins.onAssembly(new ObservableFromFuture<>(future, timeout, unit));
}
/**
* Converts an {@link Iterable} sequence into an {@code Observable} that emits the items in the sequence.
*
*
*
* - Scheduler:
* - {@code fromIterable} does not operate by default on a particular {@link Scheduler}.
*
*
* @param source
* the source {@code Iterable} sequence
* @param
* the type of items in the {@code Iterable} sequence and the type of items to be emitted by the
* resulting {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source} is {@code null}
* @see ReactiveX operators documentation: From
* @see #fromStream(Stream)
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromIterable(@NonNull Iterable extends T> source) {
Objects.requireNonNull(source, "source is null");
return RxJavaPlugins.onAssembly(new ObservableFromIterable<>(source));
}
/**
* Returns an {@code Observable} instance that when subscribed to, subscribes to the {@link MaybeSource} instance and
* emits {@code onSuccess} as a single item or forwards any {@code onComplete} or
* {@code onError} signal.
*
*
*
* - Scheduler:
* - {@code fromMaybe} does not operate by default on a particular {@link Scheduler}.
*
* @param the value type of the {@code MaybeSource} element
* @param maybe the {@code MaybeSource} instance to subscribe to, not {@code null}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code maybe} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromMaybe(@NonNull MaybeSource maybe) {
Objects.requireNonNull(maybe, "maybe is null");
return RxJavaPlugins.onAssembly(new MaybeToObservable<>(maybe));
}
/**
* Converts an arbitrary Reactive Streams {@link Publisher} into an {@code Observable}.
*
*
*
* The {@code Publisher} must follow the
* Reactive-Streams specification.
* Violating the specification may result in undefined behavior.
*
* If possible, use {@link #create(ObservableOnSubscribe)} to create a
* source-like {@code Observable} instead.
*
* Note that even though {@code Publisher} appears to be a functional interface, it
* is not recommended to implement it through a lambda as the specification requires
* state management that is not achievable with a stateless lambda.
*
* - Backpressure:
* - The source {@code publisher} is consumed in an unbounded fashion without applying any
* backpressure to it.
* - Scheduler:
* - {@code fromPublisher} does not operate by default on a particular {@link Scheduler}.
*
* @param the value type of the flow
* @param publisher the {@code Publisher} to convert
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code publisher} is {@code null}
* @see #create(ObservableOnSubscribe)
*/
@BackpressureSupport(BackpressureKind.UNBOUNDED_IN)
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromPublisher(@NonNull Publisher extends T> publisher) {
Objects.requireNonNull(publisher, "publisher is null");
return RxJavaPlugins.onAssembly(new ObservableFromPublisher<>(publisher));
}
/**
* Returns an {@code Observable} instance that runs the given {@link Runnable} for each {@link Observer} and
* emits either its unchecked exception or simply completes.
*
*
*
* If the code to be wrapped needs to throw a checked or more broader {@link Throwable} exception, that
* exception has to be converted to an unchecked exception by the wrapped code itself. Alternatively,
* use the {@link #fromAction(Action)} method which allows the wrapped code to throw any {@code Throwable}
* exception and will signal it to observers as-is.
*
* - Scheduler:
* - {@code fromRunnable} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@code Runnable} throws an exception, the respective {@code Throwable} is
* delivered to the downstream via {@link Observer#onError(Throwable)},
* except when the downstream has canceled the resulting {@code Observable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link io.reactivex.rxjava3.exceptions.UndeliverableException UndeliverableException}.
*
*
* @param the target type
* @param run the {@code Runnable} to run for each {@code Observer}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code run} is {@code null}
* @since 3.0.0
* @see #fromAction(Action)
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromRunnable(@NonNull Runnable run) {
Objects.requireNonNull(run, "run is null");
return RxJavaPlugins.onAssembly(new ObservableFromRunnable<>(run));
}
/**
* Returns an {@code Observable} instance that when subscribed to, subscribes to the {@link SingleSource} instance and
* emits {@code onSuccess} as a single item or forwards the {@code onError} signal.
*
*
*
* - Scheduler:
* - {@code fromSingle} does not operate by default on a particular {@link Scheduler}.
*
* @param the value type of the {@code SingleSource} element
* @param source the {@code SingleSource} instance to subscribe to, not {@code null}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code source} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromSingle(@NonNull SingleSource source) {
Objects.requireNonNull(source, "source is null");
return RxJavaPlugins.onAssembly(new SingleToObservable<>(source));
}
/**
* Returns an {@code Observable} that, when an observer subscribes to it, invokes a supplier function you specify and then
* emits the value returned from that function.
*
*
*
* This allows you to defer the execution of the function you specify until an observer subscribes to the
* {@code Observable}. That is to say, it makes the function "lazy."
*
* - Scheduler:
* - {@code fromSupplier} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@link Supplier} throws an exception, the respective {@link Throwable} is
* delivered to the downstream via {@link Observer#onError(Throwable)},
* except when the downstream has disposed the current {@code Observable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link UndeliverableException}.
*
*
* @param supplier
* a function, the execution of which should be deferred; {@code fromSupplier} will invoke this
* function only when an observer subscribes to the {@code Observable} that {@code fromSupplier} returns
* @param
* the type of the item emitted by the {@code Observable}
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code supplier} is {@code null}
* @see #defer(Supplier)
* @see #fromCallable(Callable)
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable fromSupplier(@NonNull Supplier extends T> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return RxJavaPlugins.onAssembly(new ObservableFromSupplier<>(supplier));
}
/**
* Returns a cold, synchronous and stateless generator of values.
*
*
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the generated value type
* @param generator the {@link Consumer} called in a loop after a downstream {@link Observer} has
* subscribed. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code generator} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable generate(@NonNull Consumer> generator) {
Objects.requireNonNull(generator, "generator is null");
return generate(Functions.nullSupplier(),
ObservableInternalHelper.simpleGenerator(generator), Functions.emptyConsumer());
}
/**
* Returns a cold, synchronous and stateful generator of values.
*
*
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Observer} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Observer}
* @param generator the {@link BiConsumer} called in a loop after a downstream {@code Observer} has
* subscribed. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code initialState} or {@code generator} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull S> Observable generate(@NonNull Supplier initialState, @NonNull BiConsumer> generator) {
Objects.requireNonNull(generator, "generator is null");
return generate(initialState, ObservableInternalHelper.simpleBiGenerator(generator), Functions.emptyConsumer());
}
/**
* Returns a cold, synchronous and stateful generator of values.
*
*
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Observer} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Observer}
* @param generator the {@link BiConsumer} called in a loop after a downstream {@code Observer} has
* subscribed. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @param disposeState the {@link Consumer} that is called with the current state when the generator
* terminates the sequence or it gets disposed
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code initialState}, {@code generator} or {@code disposeState} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull S> Observable generate(
@NonNull Supplier initialState,
@NonNull BiConsumer> generator,
@NonNull Consumer super S> disposeState) {
Objects.requireNonNull(generator, "generator is null");
return generate(initialState, ObservableInternalHelper.simpleBiGenerator(generator), disposeState);
}
/**
* Returns a cold, synchronous and stateful generator of values.
*
*
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Observer} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Observer}
* @param generator the {@link BiConsumer} called in a loop after a downstream {@code Observer} has
* subscribed. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event and should return a (new) state for
* the next invocation. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code initialState} or {@code generator} is {@code null}
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T, @NonNull S> Observable generate(@NonNull Supplier initialState, @NonNull BiFunction, S> generator) {
return generate(initialState, generator, Functions.emptyConsumer());
}
/**
* Returns a cold, synchronous and stateful generator of values.
*
*
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Observer} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Observer}
* @param generator the {@link BiConsumer} called in a loop after a downstream {@code Observer} has
* subscribed. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event and should return a (new) state for
* the next invocation. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @param disposeState the {@link Consumer} that is called with the current state when the generator
* terminates the sequence or it gets disposed
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code initialState}, {@code generator} or {@code disposeState} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull S> Observable generate(@NonNull Supplier initialState, @NonNull BiFunction, S> generator,
@NonNull Consumer super S> disposeState) {
Objects.requireNonNull(initialState, "initialState is null");
Objects.requireNonNull(generator, "generator is null");
Objects.requireNonNull(disposeState, "disposeState is null");
return RxJavaPlugins.onAssembly(new ObservableGenerate<>(initialState, generator, disposeState));
}
/**
* Returns an {@code Observable} that emits a {@code 0L} after the {@code initialDelay} and ever increasing numbers
* after each {@code period} of time thereafter.
*
*
*
* - Scheduler:
* - {@code interval} operates by default on the {@code computation} {@link Scheduler}.
*
*
* @param initialDelay
* the initial delay time to wait before emitting the first value of 0L
* @param period
* the period of time between emissions of the subsequent numbers
* @param unit
* the time unit for both {@code initialDelay} and {@code period}
* @return the new {@code Observable} instance
* @see ReactiveX operators documentation: Interval
* @throws NullPointerException if {@code unit} is {@code null}
* @since 1.0.12
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.COMPUTATION)
@NonNull
public static Observable interval(long initialDelay, long period, @NonNull TimeUnit unit) {
return interval(initialDelay, period, unit, Schedulers.computation());
}
/**
* Returns an {@code Observable} that emits a {@code 0L} after the {@code initialDelay} and ever increasing numbers
* after each {@code period} of time thereafter, on a specified {@link Scheduler}.
*
*
*
* - Scheduler:
* - You specify which {@code Scheduler} this operator will use.
*
*
* @param initialDelay
* the initial delay time to wait before emitting the first value of 0L
* @param period
* the period of time between emissions of the subsequent numbers
* @param unit
* the time unit for both {@code initialDelay} and {@code period}
* @param scheduler
* the {@code Scheduler} on which the waiting happens and items are emitted
* @return the new {@code Observable} instance
* @see ReactiveX operators documentation: Interval
* @since 1.0.12
* @throws NullPointerException if {@code unit} or {@code scheduler} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.CUSTOM)
public static Observable interval(long initialDelay, long period, @NonNull TimeUnit unit, @NonNull Scheduler scheduler) {
Objects.requireNonNull(unit, "unit is null");
Objects.requireNonNull(scheduler, "scheduler is null");
return RxJavaPlugins.onAssembly(new ObservableInterval(Math.max(0L, initialDelay), Math.max(0L, period), unit, scheduler));
}
/**
* Returns an {@code Observable} that emits a sequential number every specified interval of time.
*
*
*
* - Scheduler:
* - {@code interval} operates by default on the {@code computation} {@link Scheduler}.
*
*
* @param period
* the period size in time units (see below)
* @param unit
* time units to use for the interval size
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code unit} is {@code null}
* @see ReactiveX operators documentation: Interval
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.COMPUTATION)
@NonNull
public static Observable interval(long period, @NonNull TimeUnit unit) {
return interval(period, period, unit, Schedulers.computation());
}
/**
* Returns an {@code Observable} that emits a sequential number every specified interval of time, on a
* specified {@link Scheduler}.
*
*
*
* - Scheduler:
* - You specify which {@code Scheduler} this operator will use.
*
*
* @param period
* the period size in time units (see below)
* @param unit
* time units to use for the interval size
* @param scheduler
* the {@code Scheduler} to use for scheduling the items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code unit} or {@code scheduler} is {@code null}
* @see ReactiveX operators documentation: Interval
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.CUSTOM)
@NonNull
public static Observable interval(long period, @NonNull TimeUnit unit, @NonNull Scheduler scheduler) {
return interval(period, period, unit, scheduler);
}
/**
* Signals a range of long values, the first after some initial delay and the rest periodically after.
*
* The sequence completes immediately after the last value (start + count - 1) has been reached.
*
*
*
* - Scheduler:
* - {@code intervalRange} by default operates on the {@link Schedulers#computation() computation} {@link Scheduler}.
*
* @param start that start value of the range
* @param count the number of values to emit in total, if zero, the operator emits an {@code onComplete} after the initial delay.
* @param initialDelay the initial delay before signaling the first value (the start)
* @param period the period between subsequent values
* @param unit the unit of measure of the {@code initialDelay} and {@code period} amounts
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code unit} is {@code null}
* @throws IllegalArgumentException
* if {@code count} is negative, or if {@code start} + {@code count} − 1 exceeds
* {@link Long#MAX_VALUE}
* @see #range(int, int)
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.COMPUTATION)
public static Observable intervalRange(long start, long count, long initialDelay, long period, @NonNull TimeUnit unit) {
return intervalRange(start, count, initialDelay, period, unit, Schedulers.computation());
}
/**
* Signals a range of long values, the first after some initial delay and the rest periodically after.
*
* The sequence completes immediately after the last value (start + count - 1) has been reached.
*
*
*
* - Scheduler:
* - you provide the {@link Scheduler}.
*
* @param start that start value of the range
* @param count the number of values to emit in total, if zero, the operator emits an {@code onComplete} after the initial delay.
* @param initialDelay the initial delay before signaling the first value (the start)
* @param period the period between subsequent values
* @param unit the unit of measure of the {@code initialDelay} and {@code period} amounts
* @param scheduler the target scheduler where the values and terminal signals will be emitted
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code unit} or {@code scheduler} is {@code null}
* @throws IllegalArgumentException
* if {@code count} is negative, or if {@code start} + {@code count} − 1 exceeds
* {@link Long#MAX_VALUE}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.CUSTOM)
public static Observable intervalRange(long start, long count, long initialDelay, long period, @NonNull TimeUnit unit, @NonNull Scheduler scheduler) {
if (count < 0) {
throw new IllegalArgumentException("count >= 0 required but it was " + count);
}
if (count == 0L) {
return Observable.empty().delay(initialDelay, unit, scheduler);
}
long end = start + (count - 1);
if (start > 0 && end < 0) {
throw new IllegalArgumentException("Overflow! start + count is bigger than Long.MAX_VALUE");
}
Objects.requireNonNull(unit, "unit is null");
Objects.requireNonNull(scheduler, "scheduler is null");
return RxJavaPlugins.onAssembly(new ObservableIntervalRange(start, end, Math.max(0L, initialDelay), Math.max(0L, period), unit, scheduler));
}
/**
* Returns an {@code Observable} that signals the given (constant reference) item and then completes.
*
*
*
* Note that the item is taken and re-emitted as is and not computed by any means by {@code just}. Use {@link #fromCallable(Callable)}
* to generate a single item on demand (when {@link Observer}s subscribe to it).
*
* See the multi-parameter overloads of {@code just} to emit more than one (constant reference) items one after the other.
* Use {@link #fromArray(Object...)} to emit an arbitrary number of items that are known upfront.
*
* To emit the items of an {@link Iterable} sequence (such as a {@link java.util.List}), use {@link #fromIterable(Iterable)}.
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item
* the item to emit
* @param
* the type of that item
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item} is {@code null}
* @see ReactiveX operators documentation: Just
* @see #just(Object, Object)
* @see #fromCallable(Callable)
* @see #fromArray(Object...)
* @see #fromIterable(Iterable)
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item) {
Objects.requireNonNull(item, "item is null");
return RxJavaPlugins.onAssembly(new ObservableJust<>(item));
}
/**
* Converts two items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1} or {@code item2} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
return fromArray(item1, item2);
}
/**
* Converts three items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2} or {@code item3} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
return fromArray(item1, item2, item3);
}
/**
* Converts four items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3} or {@code item4} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3, @NonNull T item4) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
return fromArray(item1, item2, item3, item4);
}
/**
* Converts five items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4} or {@code item5} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3, @NonNull T item4, @NonNull T item5) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
return fromArray(item1, item2, item3, item4, item5);
}
/**
* Converts six items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5} or {@code item6} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3, @NonNull T item4, @NonNull T item5, @NonNull T item6) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
return fromArray(item1, item2, item3, item4, item5, item6);
}
/**
* Converts seven items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6}
* or {@code item7} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3, @NonNull T item4, @NonNull T item5, @NonNull T item6, @NonNull T item7) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7);
}
/**
* Converts eight items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param item8
* eighth item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6}
* {@code item7} or {@code item8} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3, @NonNull T item4, @NonNull T item5, @NonNull T item6, @NonNull T item7, @NonNull T item8) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
Objects.requireNonNull(item8, "item8 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7, item8);
}
/**
* Converts nine items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param item8
* eighth item
* @param item9
* ninth item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6}
* {@code item7}, {@code item8} or {@code item9} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3, @NonNull T item4, @NonNull T item5, @NonNull T item6, @NonNull T item7, @NonNull T item8, @NonNull T item9) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
Objects.requireNonNull(item8, "item8 is null");
Objects.requireNonNull(item9, "item9 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7, item8, item9);
}
/**
* Converts ten items into an {@code Observable} that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param item8
* eighth item
* @param item9
* ninth item
* @param item10
* tenth item
* @param
* the type of these items
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6}
* {@code item7}, {@code item8}, {@code item9}
* or {@code item10} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Observable just(@NonNull T item1, @NonNull T item2, @NonNull T item3, @NonNull T item4, @NonNull T item5, @NonNull T item6, @NonNull T item7, @NonNull T item8, @NonNull T item9, @NonNull T item10) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
Objects.requireNonNull(item8, "item8 is null");
Objects.requireNonNull(item9, "item9 is null");
Objects.requireNonNull(item10, "item10 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7, item8, item9, item10);
}
/**
* Flattens an {@link Iterable} of {@link ObservableSource}s into one {@code Observable}, without any transformation, while limiting the
* number of concurrent subscriptions to these {@code ObservableSource}s.
*
*
*
* You can combine the items emitted by multiple {@code ObservableSource}s so that they appear as a single {@code ObservableSource}, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the returned {@code ObservableSource}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Observable} terminates with that {@code Throwable} and all other source {@code ObservableSource}s are disposed.
* If more than one {@code ObservableSource} signals an error, the resulting {@code Observable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Observable} has been disposed or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeDelayError(Iterable, int, int)} to merge sources and terminate only when all source {@code ObservableSource}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the {@code Iterable} of {@code ObservableSource}s
* @param maxConcurrency
* the maximum number of {@code ObservableSource}s that may be subscribed to concurrently
* @param bufferSize
* the number of items expected from each inner {@code ObservableSource} to be buffered
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException
* if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: Merge
* @see #mergeDelayError(Iterable, int, int)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable merge(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources, int maxConcurrency, int bufferSize) {
return fromIterable(sources).flatMap((Function)Functions.identity(), false, maxConcurrency, bufferSize);
}
/**
* Flattens an array of {@link ObservableSource}s into one {@code Observable}, without any transformation, while limiting the
* number of concurrent subscriptions to these {@code ObservableSource}s.
*
*
*
* You can combine the items emitted by multiple {@code ObservableSource}s so that they appear as a single {@code ObservableSource}, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code mergeArray} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the {@code ObservableSource}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Observable} terminates with that {@code Throwable} and all other source {@code ObservableSource}s are disposed.
* If more than one {@code ObservableSource} signals an error, the resulting {@code Observable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Observable} has been disposed or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeArrayDelayError(int, int, ObservableSource...)} to merge sources and terminate only when all source {@code ObservableSource}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the array of {@code ObservableSource}s
* @param maxConcurrency
* the maximum number of {@code ObservableSource}s that may be subscribed to concurrently
* @param bufferSize
* the number of items expected from each inner {@code ObservableSource} to be buffered
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException
* if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: Merge
* @see #mergeArrayDelayError(int, int, ObservableSource...)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
@SafeVarargs
public static <@NonNull T> Observable mergeArray(int maxConcurrency, int bufferSize, @NonNull ObservableSource extends T>... sources) {
return fromArray(sources).flatMap((Function)Functions.identity(), false, maxConcurrency, bufferSize);
}
/**
* Flattens an {@link Iterable} of {@link ObservableSource}s into one {@code Observable}, without any transformation.
*
*
*
* You can combine the items emitted by multiple {@code ObservableSource}s so that they appear as a single {@code ObservableSource}, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the returned {@code ObservableSource}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Observable} terminates with that {@code Throwable} and all other source {@code ObservableSource}s are disposed.
* If more than one {@code ObservableSource} signals an error, the resulting {@code Observable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Observable} has been disposed or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeDelayError(Iterable)} to merge sources and terminate only when all source {@code ObservableSource}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the {@code Iterable} of {@code ObservableSource}s
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Merge
* @see #mergeDelayError(Iterable)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable merge(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources) {
return fromIterable(sources).flatMap((Function)Functions.identity());
}
/**
* Flattens an {@link Iterable} of {@link ObservableSource}s into one {@code Observable}, without any transformation, while limiting the
* number of concurrent subscriptions to these {@code ObservableSource}s.
*
*
*
* You can combine the items emitted by multiple {@code ObservableSource}s so that they appear as a single {@code ObservableSource}, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the returned {@code ObservableSource}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Observable} terminates with that {@code Throwable} and all other source {@code ObservableSource}s are disposed.
* If more than one {@code ObservableSource} signals an error, the resulting {@code Observable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Observable} has been disposed or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeDelayError(Iterable, int)} to merge sources and terminate only when all source {@code ObservableSource}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the {@code Iterable} of {@code ObservableSource}s
* @param maxConcurrency
* the maximum number of {@code ObservableSource}s that may be subscribed to concurrently
* @return the new {@code Observable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException
* if {@code maxConcurrency} is less than or equal to 0
* @see ReactiveX operators documentation: Merge
* @see #mergeDelayError(Iterable, int)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Observable merge(@NonNull Iterable<@NonNull ? extends ObservableSource extends T>> sources, int maxConcurrency) {
return fromIterable(sources).flatMap((Function)Functions.identity(), maxConcurrency);
}
/**
* Flattens an {@link ObservableSource} that emits {@code ObservableSource}s into a single {@code Observable} that emits the items emitted by
* those {@code ObservableSource}s, without any transformation.
*
*
*
* You can combine the items emitted by multiple {@code ObservableSource}s so that they appear as a single {@code ObservableSource}, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the returned {@code ObservableSource}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Observable} terminates with that {@code Throwable} and all other source {@code ObservableSource}s are disposed.
* If more than one {@code ObservableSource} signals an error, the resulting {@code Observable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Observable} has been disposed or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeDelayError(ObservableSource)} to merge sources and terminate only when all source {@code ObservableSource}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* an {@code ObservableSource} that emits {@code ObservableSource}s
* @return the new {@code Observable} instance
* @see ReactiveX operators documentation: Merge
* @throws NullPointerException if {@code sources} is {@code null}
* @see #mergeDelayError(ObservableSource)
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings({ "unchecked", "rawtypes" })
@NonNull
public static <@NonNull T> Observable merge(@NonNull ObservableSource extends ObservableSource extends T>> sources) {
Objects.requireNonNull(sources, "sources is null");
return RxJavaPlugins.onAssembly(new ObservableFlatMap(sources, Functions.identity(), false, Integer.MAX_VALUE, bufferSize()));
}
/**
* Flattens an {@link ObservableSource} that emits {@code ObservableSource}s into a single {@code Observable} that emits the items emitted by
* those {@code ObservableSource}s, without any transformation, while limiting the maximum number of concurrent
* subscriptions to these {@code ObservableSource}s.
*
*
*
* You can combine the items emitted by multiple {@code ObservableSource}s so that they appear as a single {@code ObservableSource}, by
* using the {@code merge} method.
*
*