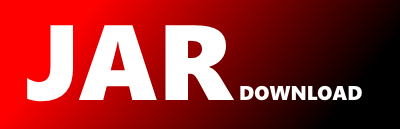
io.reactivex.rxjava3.core.Flowable Maven / Gradle / Ivy
Show all versions of rxjava Show documentation
/*
* Copyright (c) 2016-present, RxJava Contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package io.reactivex.rxjava3.core;
import java.util.*;
import java.util.concurrent.*;
import java.util.stream.*;
import org.reactivestreams.*;
import io.reactivex.rxjava3.annotations.*;
import io.reactivex.rxjava3.disposables.*;
import io.reactivex.rxjava3.exceptions.*;
import io.reactivex.rxjava3.flowables.*;
import io.reactivex.rxjava3.functions.*;
import io.reactivex.rxjava3.internal.functions.*;
import io.reactivex.rxjava3.internal.jdk8.*;
import io.reactivex.rxjava3.internal.operators.flowable.*;
import io.reactivex.rxjava3.internal.operators.maybe.MaybeToFlowable;
import io.reactivex.rxjava3.internal.operators.mixed.*;
import io.reactivex.rxjava3.internal.operators.observable.ObservableFromPublisher;
import io.reactivex.rxjava3.internal.operators.single.SingleToFlowable;
import io.reactivex.rxjava3.internal.schedulers.ImmediateThinScheduler;
import io.reactivex.rxjava3.internal.subscribers.*;
import io.reactivex.rxjava3.internal.util.*;
import io.reactivex.rxjava3.operators.ScalarSupplier;
import io.reactivex.rxjava3.parallel.ParallelFlowable;
import io.reactivex.rxjava3.plugins.RxJavaPlugins;
import io.reactivex.rxjava3.schedulers.*;
import io.reactivex.rxjava3.subscribers.*;
/**
* The {@code Flowable} class that implements the Reactive Streams {@link Publisher}
* Pattern and offers factory methods, intermediate operators and the ability to consume reactive dataflows.
*
* Reactive Streams operates with {@code Publisher}s which {@code Flowable} extends. Many operators
* therefore accept general {@code Publisher}s directly and allow direct interoperation with other
* Reactive Streams implementations.
*
* The {@code Flowable} hosts the default buffer size of 128 elements for operators, accessible via {@link #bufferSize()},
* that can be overridden globally via the system parameter {@code rx3.buffer-size}. Most operators, however, have
* overloads that allow setting their internal buffer size explicitly.
*
* The documentation for this class makes use of marble diagrams. The following legend explains these diagrams:
*
*
*
* The {@code Flowable} follows the protocol
*
* onSubscribe onNext* (onError | onComplete)?
*
* where the stream can be disposed through the {@link Subscription} instance provided to consumers through
* {@link Subscriber#onSubscribe(Subscription)}.
* Unlike the {@code Observable.subscribe()} of version 1.x, {@link #subscribe(Subscriber)} does not allow external cancellation
* of a subscription and the {@link Subscriber} instance is expected to expose such capability if needed.
*
* {@code Flowable}s support backpressure and require {@code Subscriber}s to signal demand via {@link Subscription#request(long)}.
*
* Example:
*
* Disposable d = Flowable.just("Hello world!")
* .delay(1, TimeUnit.SECONDS)
* .subscribeWith(new DisposableSubscriber<String>() {
* @Override public void onStart() {
* System.out.println("Start!");
* request(1);
* }
* @Override public void onNext(String t) {
* System.out.println(t);
* request(1);
* }
* @Override public void onError(Throwable t) {
* t.printStackTrace();
* }
* @Override public void onComplete() {
* System.out.println("Done!");
* }
* });
*
* Thread.sleep(500);
* // the sequence can now be cancelled via dispose()
* d.dispose();
*
*
* The Reactive Streams specification is relatively strict when defining interactions between {@code Publisher}s and {@code Subscriber}s, so much so
* that there is a significant performance penalty due certain timing requirements and the need to prepare for invalid
* request amounts via {@link Subscription#request(long)}.
* Therefore, RxJava has introduced the {@link FlowableSubscriber} interface that indicates the consumer can be driven with relaxed rules.
* All RxJava operators are implemented with these relaxed rules in mind.
* If the subscribing {@code Subscriber} does not implement this interface, for example, due to it being from another Reactive Streams compliant
* library, the {@code Flowable} will automatically apply a compliance wrapper around it.
*
* {@code Flowable} is an abstract class, but it is not advised to implement sources and custom operators by extending the class directly due
* to the large amounts of Reactive Streams
* rules to be followed to the letter. See the wiki for
* some guidance if such custom implementations are necessary.
*
* The recommended way of creating custom {@code Flowable}s is by using the {@link #create(FlowableOnSubscribe, BackpressureStrategy)} factory method:
*
* Flowable<String> source = Flowable.create(new FlowableOnSubscribe<String>() {
* @Override
* public void subscribe(FlowableEmitter<String> emitter) throws Exception {
*
* // signal an item
* emitter.onNext("Hello");
*
* // could be some blocking operation
* Thread.sleep(1000);
*
* // the consumer might have cancelled the flow
* if (emitter.isCancelled()) {
* return;
* }
*
* emitter.onNext("World");
*
* Thread.sleep(1000);
*
* // the end-of-sequence has to be signaled, otherwise the
* // consumers may never finish
* emitter.onComplete();
* }
* }, BackpressureStrategy.BUFFER);
*
* System.out.println("Subscribe!");
*
* source.subscribe(System.out::println);
*
* System.out.println("Done!");
*
*
* RxJava reactive sources, such as {@code Flowable}, are generally synchronous and sequential in nature. In the ReactiveX design, the location (thread)
* where operators run is orthogonal to when the operators can work with data. This means that asynchrony and parallelism
* has to be explicitly expressed via operators such as {@link #subscribeOn(Scheduler)}, {@link #observeOn(Scheduler)} and {@link #parallel()}. In general,
* operators featuring a {@link Scheduler} parameter are introducing this type of asynchrony into the flow.
*
* For more information see the ReactiveX documentation.
*
* @param
* the type of the items emitted by the {@code Flowable}
* @see Observable
* @see ParallelFlowable
* @see io.reactivex.rxjava3.subscribers.DisposableSubscriber
*/
public abstract class Flowable<@NonNull T> implements Publisher {
/** The default buffer size. */
static final int BUFFER_SIZE;
static {
BUFFER_SIZE = Math.max(1, Integer.getInteger("rx3.buffer-size", 128));
}
/**
* Mirrors the one {@link Publisher} in an {@link Iterable} of several {@code Publisher}s that first either emits an item or sends
* a termination notification.
*
*
*
* When one of the {@code Publisher}s signal an item or terminates first, all subscriptions to the other
* {@code Publisher}s are canceled.
*
* - Backpressure:
* - The operator itself doesn't interfere with backpressure which is determined by the winning
* {@code Publisher}'s backpressure behavior.
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* -
* If any of the losing {@code Publisher}s signals an error, the error is routed to the global
* error handler via {@link RxJavaPlugins#onError(Throwable)}.
*
*
*
* @param the common element type
* @param sources
* an {@code Iterable} of {@code Publisher}s sources competing to react first. A subscription to each {@code Publisher} will
* occur in the same order as in this {@code Iterable}.
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Amb
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable amb(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources) {
Objects.requireNonNull(sources, "sources is null");
return RxJavaPlugins.onAssembly(new FlowableAmb<>(null, sources));
}
/**
* Mirrors the one {@link Publisher} in an array of several {@code Publisher}s that first either emits an item or sends
* a termination notification.
*
*
*
* When one of the {@code Publisher}s signal an item or terminates first, all subscriptions to the other
* {@code Publisher}s are canceled.
*
* - Backpressure:
* - The operator itself doesn't interfere with backpressure which is determined by the winning
* {@code Publisher}'s backpressure behavior.
* - Scheduler:
* - {@code ambArray} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* -
* If any of the losing {@code Publisher}s signals an error, the error is routed to the global
* error handler via {@link RxJavaPlugins#onError(Throwable)}.
*
*
*
* @param the common element type
* @param sources
* an array of {@code Publisher} sources competing to react first. A subscription to each {@code Publisher} will
* occur in the same order as in this array.
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Amb
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
public static <@NonNull T> Flowable ambArray(@NonNull Publisher extends T>... sources) {
Objects.requireNonNull(sources, "sources is null");
int len = sources.length;
if (len == 0) {
return empty();
} else
if (len == 1) {
return fromPublisher(sources[0]);
}
return RxJavaPlugins.onAssembly(new FlowableAmb<>(sources, null));
}
/**
* Returns the default internal buffer size used by most async operators.
* The value can be overridden via system parameter {@code rx3.buffer-size}
* before the {@code Flowable} class is loaded.
* @return the default internal buffer size.
*/
@CheckReturnValue
public static int bufferSize() {
return BUFFER_SIZE;
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatestArray} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@NonNull
public static <@NonNull T, @NonNull R> Flowable combineLatestArray(@NonNull Publisher extends T>[] sources, @NonNull Function super Object[], ? extends R> combiner) {
return combineLatestArray(sources, combiner, bufferSize());
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatestArray} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @param bufferSize
* the internal buffer size and prefetch amount applied to every source {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
public static <@NonNull T, @NonNull R> Flowable combineLatestArray(@NonNull Publisher extends T>[] sources, @NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
if (sources.length == 0) {
return empty();
}
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
return RxJavaPlugins.onAssembly(new FlowableCombineLatest<>(sources, combiner, bufferSize, false));
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided iterable of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@NonNull
public static <@NonNull T, @NonNull R> Flowable combineLatest(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner) {
return combineLatest(sources, combiner, bufferSize());
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided iterable of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting any items and
* without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @param bufferSize
* the internal buffer size and prefetch amount applied to every source {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
public static <@NonNull T, @NonNull R> Flowable combineLatest(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
return RxJavaPlugins.onAssembly(new FlowableCombineLatest<>(sources, combiner, bufferSize, false));
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatestArrayDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@NonNull
public static <@NonNull T, @NonNull R> Flowable combineLatestArrayDelayError(@NonNull Publisher extends T>[] sources,
@NonNull Function super Object[], ? extends R> combiner) {
return combineLatestArrayDelayError(sources, combiner, bufferSize());
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function and delays any error from the sources until
* all source {@code Publisher}s terminate.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided array of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatestArrayDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @param bufferSize
* the internal buffer size and prefetch amount applied to every source {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
public static <@NonNull T, @NonNull R> Flowable combineLatestArrayDelayError(@NonNull Publisher extends T>[] sources,
@NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
if (sources.length == 0) {
return empty();
}
return RxJavaPlugins.onAssembly(new FlowableCombineLatest<>(sources, combiner, bufferSize, true));
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function and delays any error from the sources until
* all source {@code Publisher}s terminate.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided iterable of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatestDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@NonNull
public static <@NonNull T, @NonNull R> Flowable combineLatestDelayError(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner) {
return combineLatestDelayError(sources, combiner, bufferSize());
}
/**
* Combines a collection of source {@link Publisher}s by emitting an item that aggregates the latest values of each of
* the source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function and delays any error from the sources until
* all source {@code Publisher}s terminate.
*
* Note on method signature: since Java doesn't allow creating a generic array with {@code new T[]}, the
* implementation of this operator has to create an {@code Object[]} instead. Unfortunately, a
* {@code Function} passed to the method would trigger a {@link ClassCastException}.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
* If the provided iterable of source {@code Publisher}s is empty, the resulting sequence completes immediately without emitting
* any items and without any calls to the combiner function.
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatestDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the collection of source {@code Publisher}s
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @param bufferSize
* the internal buffer size and prefetch amount applied to every source {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} or {@code combiner} is {@code null}
* @throws IllegalArgumentException if {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: CombineLatest
*/
@SchedulerSupport(SchedulerSupport.NONE)
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@NonNull
public static <@NonNull T, @NonNull R> Flowable combineLatestDelayError(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources,
@NonNull Function super Object[], ? extends R> combiner, int bufferSize) {
Objects.requireNonNull(sources, "sources is null");
Objects.requireNonNull(combiner, "combiner is null");
ObjectHelper.verifyPositive(bufferSize, "bufferSize");
return RxJavaPlugins.onAssembly(new FlowableCombineLatest<>(sources, combiner, bufferSize, true));
}
/**
* Combines two source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from either of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T1, @NonNull T2, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull BiFunction super T1, ? super T2, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines three source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param source3
* the third source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull Publisher extends T3> source3,
@NonNull Function3 super T1, ? super T2, ? super T3, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2, source3 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines four source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param source3
* the third source {@code Publisher}
* @param source4
* the fourth source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull Publisher extends T3> source3, @NonNull Publisher extends T4> source4,
@NonNull Function4 super T1, ? super T2, ? super T3, ? super T4, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2, source3, source4 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines five source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param source3
* the third source {@code Publisher}
* @param source4
* the fourth source {@code Publisher}
* @param source5
* the fifth source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull Publisher extends T3> source3, @NonNull Publisher extends T4> source4,
@NonNull Publisher extends T5> source5,
@NonNull Function5 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2, source3, source4, source5 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines six source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param source3
* the third source {@code Publisher}
* @param source4
* the fourth source {@code Publisher}
* @param source5
* the fifth source {@code Publisher}
* @param source6
* the sixth source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull Publisher extends T3> source3, @NonNull Publisher extends T4> source4,
@NonNull Publisher extends T5> source5, @NonNull Publisher extends T6> source6,
@NonNull Function6 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2, source3, source4, source5, source6 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines seven source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the element type of the seventh source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param source3
* the third source {@code Publisher}
* @param source4
* the fourth source {@code Publisher}
* @param source5
* the fifth source {@code Publisher}
* @param source6
* the sixth source {@code Publisher}
* @param source7
* the seventh source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6},
* {@code source7} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull T7, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull Publisher extends T3> source3, @NonNull Publisher extends T4> source4,
@NonNull Publisher extends T5> source5, @NonNull Publisher extends T6> source6,
@NonNull Publisher extends T7> source7,
@NonNull Function7 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(source7, "source7 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2, source3, source4, source5, source6, source7 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines eight source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the element type of the seventh source
* @param the element type of the eighth source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param source3
* the third source {@code Publisher}
* @param source4
* the fourth source {@code Publisher}
* @param source5
* the fifth source {@code Publisher}
* @param source6
* the sixth source {@code Publisher}
* @param source7
* the seventh source {@code Publisher}
* @param source8
* the eighth source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6},
* {@code source7}, {@code source8} or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull T7, @NonNull T8, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull Publisher extends T3> source3, @NonNull Publisher extends T4> source4,
@NonNull Publisher extends T5> source5, @NonNull Publisher extends T6> source6,
@NonNull Publisher extends T7> source7, @NonNull Publisher extends T8> source8,
@NonNull Function8 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(source7, "source7 is null");
Objects.requireNonNull(source8, "source8 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2, source3, source4, source5, source6, source7, source8 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Combines nine source {@link Publisher}s by emitting an item that aggregates the latest values of each of the
* source {@code Publisher}s each time an item is received from any of the source {@code Publisher}s, where this
* aggregation is defined by a specified function.
*
* If any of the sources never produces an item but only terminates (normally or with an error), the
* resulting sequence terminates immediately (normally or with all the errors accumulated until that point).
* If that input source is also synchronous, other sources after it will not be subscribed to.
*
*
*
* - Backpressure:
* - The returned {@code Publisher} honors backpressure from downstream. The source {@code Publisher}s
* are requested in a bounded manner, however, their backpressure is not enforced (the operator won't signal
* {@link MissingBackpressureException}) and may lead to {@link OutOfMemoryError} due to internal buffer bloat.
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the first source
* @param the element type of the second source
* @param the element type of the third source
* @param the element type of the fourth source
* @param the element type of the fifth source
* @param the element type of the sixth source
* @param the element type of the seventh source
* @param the element type of the eighth source
* @param the element type of the ninth source
* @param the combined output type
* @param source1
* the first source {@code Publisher}
* @param source2
* the second source {@code Publisher}
* @param source3
* the third source {@code Publisher}
* @param source4
* the fourth source {@code Publisher}
* @param source5
* the fifth source {@code Publisher}
* @param source6
* the sixth source {@code Publisher}
* @param source7
* the seventh source {@code Publisher}
* @param source8
* the eighth source {@code Publisher}
* @param source9
* the ninth source {@code Publisher}
* @param combiner
* the aggregation function used to combine the items emitted by the source {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3},
* {@code source4}, {@code source5}, {@code source6},
* {@code source7}, {@code source8}, {@code source9}
* or {@code combiner} is {@code null}
* @see ReactiveX operators documentation: CombineLatest
*/
@SuppressWarnings("unchecked")
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T1, @NonNull T2, @NonNull T3, @NonNull T4, @NonNull T5, @NonNull T6, @NonNull T7, @NonNull T8, @NonNull T9, @NonNull R> Flowable combineLatest(
@NonNull Publisher extends T1> source1, @NonNull Publisher extends T2> source2,
@NonNull Publisher extends T3> source3, @NonNull Publisher extends T4> source4,
@NonNull Publisher extends T5> source5, @NonNull Publisher extends T6> source6,
@NonNull Publisher extends T7> source7, @NonNull Publisher extends T8> source8,
@NonNull Publisher extends T9> source9,
@NonNull Function9 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? extends R> combiner) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
Objects.requireNonNull(source5, "source5 is null");
Objects.requireNonNull(source6, "source6 is null");
Objects.requireNonNull(source7, "source7 is null");
Objects.requireNonNull(source8, "source8 is null");
Objects.requireNonNull(source9, "source9 is null");
Objects.requireNonNull(combiner, "combiner is null");
return combineLatestArray(new Publisher[] { source1, source2, source3, source4, source5, source6, source7, source8, source9 }, Functions.toFunction(combiner), bufferSize());
}
/**
* Concatenates elements of each {@link Publisher} provided via an {@link Iterable} sequence into a single sequence
* of elements without interleaving them.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, it may throw an
* {@link IllegalStateException} when that {@code Publisher} completes.
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
* @param the common value type of the sources
* @param sources the {@code Iterable} sequence of {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable concat(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources) {
Objects.requireNonNull(sources, "sources is null");
// unlike general sources, fromIterable can only throw on a boundary because it is consumed only there
return fromIterable(sources).concatMapDelayError((Function)Functions.identity(), false, 2);
}
/**
* Returns a {@code Flowable} that emits the items emitted by each of the {@link Publisher}s emitted by the source
* {@code Publisher}, one after the other, without interleaving them.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. Both the outer and inner {@code Publisher}
* sources are expected to honor backpressure as well. If the outer violates this, a
* {@link MissingBackpressureException} is signaled. If any of the inner {@code Publisher}s violates
* this, it may throw an {@link IllegalStateException} when an inner {@code Publisher} completes.
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources
* a {@code Publisher} that emits {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concat(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources) {
return concat(sources, bufferSize());
}
/**
* Returns a {@code Flowable} that emits the items emitted by each of the {@link Publisher}s emitted by the source
* {@code Publisher}, one after the other, without interleaving them.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. Both the outer and inner {@code Publisher}
* sources are expected to honor backpressure as well. If the outer violates this, a
* {@link MissingBackpressureException} is signaled. If any of the inner {@code Publisher}s violates
* this, it may throw an {@link IllegalStateException} when an inner {@code Publisher} completes.
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources
* a {@code Publisher} that emits {@code Publisher}s
* @param prefetch
* the number of {@code Publisher}s to prefetch from the sources sequence.
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code prefetch} is non-positive
* @see ReactiveX operators documentation: Concat
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concat(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources, int prefetch) {
return fromPublisher(sources).concatMap((Function)Functions.identity(), prefetch);
}
/**
* Returns a {@code Flowable} that emits the items emitted by two {@link Publisher}s, one after the other, without
* interleaving them.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, it may throw an
* {@link IllegalStateException} when that source {@code Publisher} completes.
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param source1
* a {@code Publisher} to be concatenated
* @param source2
* a {@code Publisher} to be concatenated
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1} or {@code source2} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable concat(@NonNull Publisher extends T> source1, @NonNull Publisher extends T> source2) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
return concatArray(source1, source2);
}
/**
* Returns a {@code Flowable} that emits the items emitted by three {@link Publisher}s, one after the other, without
* interleaving them.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, it may throw an
* {@link IllegalStateException} when that source {@code Publisher} completes.
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param source1
* a {@code Publisher} to be concatenated
* @param source2
* a {@code Publisher} to be concatenated
* @param source3
* a {@code Publisher} to be concatenated
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2} or {@code source3} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable concat(
@NonNull Publisher extends T> source1, @NonNull Publisher extends T> source2,
@NonNull Publisher extends T> source3) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
return concatArray(source1, source2, source3);
}
/**
* Returns a {@code Flowable} that emits the items emitted by four {@link Publisher}s, one after the other, without
* interleaving them.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, it may throw an
* {@link IllegalStateException} when that source {@code Publisher} completes.
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param source1
* a {@code Publisher} to be concatenated
* @param source2
* a {@code Publisher} to be concatenated
* @param source3
* a {@code Publisher} to be concatenated
* @param source4
* a {@code Publisher} to be concatenated
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source1}, {@code source2}, {@code source3} or {@code source4} is {@code null}
* @see ReactiveX operators documentation: Concat
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable concat(
@NonNull Publisher extends T> source1, @NonNull Publisher extends T> source2,
@NonNull Publisher extends T> source3, @NonNull Publisher extends T> source4) {
Objects.requireNonNull(source1, "source1 is null");
Objects.requireNonNull(source2, "source2 is null");
Objects.requireNonNull(source3, "source3 is null");
Objects.requireNonNull(source4, "source4 is null");
return concatArray(source1, source2, source3, source4);
}
/**
* Concatenates a variable number of {@link Publisher} sources.
*
* Note: named this way because of overload conflict with {@code concat(Publisher>}).
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, it may throw an
* {@link IllegalStateException} when that source {@code Publisher} completes.
* - Scheduler:
* - {@code concatArray} does not operate by default on a particular {@link Scheduler}.
*
* @param sources the array of source {@code Publisher}s
* @param the common base value type
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
@NonNull
public static <@NonNull T> Flowable concatArray(@NonNull Publisher extends T>... sources) {
Objects.requireNonNull(sources, "sources is null");
if (sources.length == 0) {
return empty();
} else
if (sources.length == 1) {
return fromPublisher(sources[0]);
}
return RxJavaPlugins.onAssembly(new FlowableConcatArray<>(sources, false));
}
/**
* Concatenates a variable number of {@link Publisher} sources and delays errors from any of them
* till all terminate.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, it may throw an
* {@link IllegalStateException} when that source {@code Publisher} completes.
* - Scheduler:
* - {@code concatArrayDelayError} does not operate by default on a particular {@link Scheduler}.
*
* @param sources the array of source {@code Publisher}s
* @param the common base value type
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
@NonNull
public static <@NonNull T> Flowable concatArrayDelayError(@NonNull Publisher extends T>... sources) {
Objects.requireNonNull(sources, "sources is null");
if (sources.length == 0) {
return empty();
} else
if (sources.length == 1) {
return fromPublisher(sources[0]);
}
return RxJavaPlugins.onAssembly(new FlowableConcatArray<>(sources, true));
}
/**
* Concatenates an array of {@link Publisher}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s and then drains them
* in order, each one after the previous one completes.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, the operator will signal a
* {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code Publisher}s that need to be eagerly concatenated
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.0
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
@NonNull
public static <@NonNull T> Flowable concatArrayEager(@NonNull Publisher extends T>... sources) {
return concatArrayEager(bufferSize(), bufferSize(), sources);
}
/**
* Concatenates an array of {@link Publisher}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s and then drains them
* in order, each one after the previous one completes.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, the operator will signal a
* {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code Publisher}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrent subscriptions at a time, {@link Integer#MAX_VALUE}
* is interpreted as an indication to subscribe to all sources at once
* @param prefetch the number of elements to prefetch from each {@code Publisher} source
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code prefetch} is non-positive
* @since 2.0
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings({ "rawtypes", "unchecked" })
@SafeVarargs
public static <@NonNull T> Flowable concatArrayEager(int maxConcurrency, int prefetch, @NonNull Publisher extends T>... sources) {
Objects.requireNonNull(sources, "sources is null");
ObjectHelper.verifyPositive(maxConcurrency, "maxConcurrency");
ObjectHelper.verifyPositive(prefetch, "prefetch");
return RxJavaPlugins.onAssembly(new FlowableConcatMapEager(new FlowableFromArray(sources), Functions.identity(), maxConcurrency, prefetch, ErrorMode.IMMEDIATE));
}
/**
* Concatenates an array of {@link Publisher}s eagerly into a single stream of values
* and delaying any errors until all sources terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s
* and then drains them in order, each one after the previous one completes.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, the operator will signal a
* {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code Publisher}s that need to be eagerly concatenated
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.2.1 - experimental
*/
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@BackpressureSupport(BackpressureKind.FULL)
@SafeVarargs
@NonNull
public static <@NonNull T> Flowable concatArrayEagerDelayError(@NonNull Publisher extends T>... sources) {
return concatArrayEagerDelayError(bufferSize(), bufferSize(), sources);
}
/**
* Concatenates an array of {@link Publisher}s eagerly into a single stream of values
* and delaying any errors until all sources terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s
* and then drains them in order, each one after the previous one completes.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The {@code Publisher}
* sources are expected to honor backpressure as well.
* If any of the source {@code Publisher}s violate this, the operator will signal a
* {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources an array of {@code Publisher}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrent subscriptions at a time, {@link Integer#MAX_VALUE}
* is interpreted as indication to subscribe to all sources at once
* @param prefetch the number of elements to prefetch from each {@code Publisher} source
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code prefetch} is non-positive
* @since 2.2.1 - experimental
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@BackpressureSupport(BackpressureKind.FULL)
@SafeVarargs
@NonNull
public static <@NonNull T> Flowable concatArrayEagerDelayError(int maxConcurrency, int prefetch, @NonNull Publisher extends T>... sources) {
return fromArray(sources).concatMapEagerDelayError((Function)Functions.identity(), true, maxConcurrency, prefetch);
}
/**
* Concatenates the {@link Iterable} sequence of {@link Publisher}s into a single sequence by subscribing to each {@code Publisher},
* one after the other, one at a time and delays any errors till the all inner {@code Publisher}s terminate.
*
*
* - Backpressure:
* - The operator honors backpressure from downstream. Both the outer and inner {@code Publisher}
* sources are expected to honor backpressure as well. If the outer violates this, a
* {@link MissingBackpressureException} is signaled. If any of the inner {@code Publisher}s violates
* this, it may throw an {@link IllegalStateException} when an inner {@code Publisher} completes.
* - Scheduler:
* - {@code concatDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources the {@code Iterable} sequence of {@code Publisher}s
* @return the new {@code Flowable} with the concatenating behavior
* @throws NullPointerException if {@code sources} is {@code null}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable concatDelayError(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources) {
Objects.requireNonNull(sources, "sources is null");
return fromIterable(sources).concatMapDelayError((Function)Functions.identity());
}
/**
* Concatenates the {@link Publisher} sequence of {@code Publisher}s into a single sequence by subscribing to each inner {@code Publisher},
* one after the other, one at a time and delays any errors till the all inner and the outer {@code Publisher}s terminate.
*
*
* - Backpressure:
* - {@code concatDelayError} fully supports backpressure.
* - Scheduler:
* - {@code concatDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources the {@code Publisher} sequence of {@code Publisher}s
* @return the new {@code Flowable} with the concatenating behavior
* @throws NullPointerException if {@code sources} is {@code null}
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concatDelayError(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources) {
return concatDelayError(sources, bufferSize(), true);
}
/**
* Concatenates the {@link Publisher} sequence of {@code Publisher}s into a single sequence by subscribing to each inner {@code Publisher},
* one after the other, one at a time and delays any errors till the all inner and the outer {@code Publisher}s terminate.
*
*
* - Backpressure:
* - {@code concatDelayError} fully supports backpressure.
* - Scheduler:
* - {@code concatDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the common element base type
* @param sources the {@code Publisher} sequence of {@code Publisher}s
* @param prefetch the number of elements to prefetch from the outer {@code Publisher}
* @param tillTheEnd if {@code true}, exceptions from the outer and all inner {@code Publisher}s are delayed to the end
* if {@code false}, exception from the outer {@code Publisher} is delayed till the current inner {@code Publisher} terminates
* @return the new {@code Flowable} with the concatenating behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code prefetch} is {@code null}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concatDelayError(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources, int prefetch, boolean tillTheEnd) {
return fromPublisher(sources).concatMapDelayError((Function)Functions.identity(), tillTheEnd, prefetch);
}
/**
* Concatenates a sequence of {@link Publisher}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s and then drains them
* in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and the inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.0
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concatEager(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources) {
return concatEager(sources, bufferSize(), bufferSize());
}
/**
* Concatenates a sequence of {@link Publisher}s eagerly into a single stream of values and
* runs a limited number of inner sequences at once.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s and then drains them
* in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and both the outer and inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code Publisher}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code Publisher}s can be active at the same time
* @param prefetch the number of elements to prefetch from each inner {@code Publisher} source
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code prefetch} is non-positive
* @since 2.0
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings({ "rawtypes", "unchecked" })
public static <@NonNull T> Flowable concatEager(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources, int maxConcurrency, int prefetch) {
Objects.requireNonNull(sources, "sources is null");
ObjectHelper.verifyPositive(maxConcurrency, "maxConcurrency");
ObjectHelper.verifyPositive(prefetch, "prefetch");
return RxJavaPlugins.onAssembly(new FlowableConcatMapEager(new FlowableFromIterable(sources), Functions.identity(), maxConcurrency, prefetch, ErrorMode.BOUNDARY));
}
/**
* Concatenates a {@link Publisher} sequence of {@code Publisher}s eagerly into a single stream of values.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code Publisher}s as they are observed. The operator buffers the values emitted by these
* {@code Publisher}s and then drains them in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and both the outer and inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 2.0
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concatEager(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources) {
return concatEager(sources, bufferSize(), bufferSize());
}
/**
* Concatenates a {@link Publisher} sequence of {@code Publisher}s eagerly into a single stream of values and
* runs a limited number of inner sequences at once.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code Publisher}s as they are observed. The operator buffers the values emitted by these
* {@code Publisher}s and then drains them in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and both the outer and inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code Publisher}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code Publisher}s can be active at the same time
* @param prefetch the number of elements to prefetch from each inner {@code Publisher} source
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code prefetch} is non-positive
* @since 2.0
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings({ "rawtypes", "unchecked" })
public static <@NonNull T> Flowable concatEager(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources, int maxConcurrency, int prefetch) {
Objects.requireNonNull(sources, "sources is null");
ObjectHelper.verifyPositive(maxConcurrency, "maxConcurrency");
ObjectHelper.verifyPositive(prefetch, "prefetch");
return RxJavaPlugins.onAssembly(new FlowableConcatMapEagerPublisher(sources, Functions.identity(), maxConcurrency, prefetch, ErrorMode.IMMEDIATE));
}
/**
* Concatenates a sequence of {@link Publisher}s eagerly into a single stream of values,
* delaying errors until all the inner sequences terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s and then drains them
* in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and the inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concatEagerDelayError(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources) {
return concatEagerDelayError(sources, bufferSize(), bufferSize());
}
/**
* Concatenates a sequence of {@link Publisher}s eagerly into a single stream of values,
* delaying errors until all the inner sequences terminate and runs a limited number
* of inner sequences at once.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* source {@code Publisher}s. The operator buffers the values emitted by these {@code Publisher}s and then drains them
* in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and both the outer and inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code Publisher}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code Publisher}s can be active at the same time
* @param prefetch the number of elements to prefetch from each inner {@code Publisher} source
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code prefetch} is non-positive
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings({ "rawtypes", "unchecked" })
public static <@NonNull T> Flowable concatEagerDelayError(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources, int maxConcurrency, int prefetch) {
Objects.requireNonNull(sources, "sources is null");
ObjectHelper.verifyPositive(maxConcurrency, "maxConcurrency");
ObjectHelper.verifyPositive(prefetch, "prefetch");
return RxJavaPlugins.onAssembly(new FlowableConcatMapEager(new FlowableFromIterable(sources), Functions.identity(), maxConcurrency, prefetch, ErrorMode.END));
}
/**
* Concatenates a {@link Publisher} sequence of {@code Publisher}s eagerly into a single stream of values,
* delaying errors until all the inner and the outer sequences terminate.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code Publisher}s as they are observed. The operator buffers the values emitted by these
* {@code Publisher}s and then drains them in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and both the outer and inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable concatEagerDelayError(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources) {
return concatEagerDelayError(sources, bufferSize(), bufferSize());
}
/**
* Concatenates a {@link Publisher} sequence of {@code Publisher}s eagerly into a single stream of values,
* delaying errors until all the inner and outer sequences terminate and runs a limited number of inner
* sequences at once.
*
*
*
* Eager concatenation means that once a subscriber subscribes, this operator subscribes to all of the
* emitted source {@code Publisher}s as they are observed. The operator buffers the values emitted by these
* {@code Publisher}s and then drains them in order, each one after the previous one completes.
*
* - Backpressure:
* - Backpressure is honored towards the downstream and both the outer and inner {@code Publisher}s are
* expected to support backpressure. Violating this assumption, the operator will
* signal {@link MissingBackpressureException}.
* - Scheduler:
* - This method does not operate by default on a particular {@link Scheduler}.
*
* @param the value type
* @param sources a sequence of {@code Publisher}s that need to be eagerly concatenated
* @param maxConcurrency the maximum number of concurrently running inner {@code Publisher}s; {@link Integer#MAX_VALUE}
* is interpreted as all inner {@code Publisher}s can be active at the same time
* @param prefetch the number of elements to prefetch from each inner {@code Publisher} source
* @return the new {@code Flowable} instance with the specified concatenation behavior
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException if {@code maxConcurrency} or {@code prefetch} is non-positive
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings({ "rawtypes", "unchecked" })
public static <@NonNull T> Flowable concatEagerDelayError(@NonNull Publisher<@NonNull ? extends Publisher extends T>> sources, int maxConcurrency, int prefetch) {
Objects.requireNonNull(sources, "sources is null");
ObjectHelper.verifyPositive(maxConcurrency, "maxConcurrency");
ObjectHelper.verifyPositive(prefetch, "prefetch");
return RxJavaPlugins.onAssembly(new FlowableConcatMapEagerPublisher(sources, Functions.identity(), maxConcurrency, prefetch, ErrorMode.END));
}
/**
* Provides an API (via a cold {@code Flowable}) that bridges the reactive world with the callback-style,
* generally non-backpressured world.
*
* Example:
*
* Flowable.<Event>create(emitter -> {
* Callback listener = new Callback() {
* @Override
* public void onEvent(Event e) {
* emitter.onNext(e);
* if (e.isLast()) {
* emitter.onComplete();
* }
* }
*
* @Override
* public void onFailure(Exception e) {
* emitter.onError(e);
* }
* };
*
* AutoCloseable c = api.someMethod(listener);
*
* emitter.setCancellable(c::close);
*
* }, BackpressureStrategy.BUFFER);
*
*
* Whenever a {@link Subscriber} subscribes to the returned {@code Flowable}, the provided
* {@link FlowableOnSubscribe} callback is invoked with a fresh instance of a {@link FlowableEmitter}
* that will interact only with that specific {@code Subscriber}. If this {@code Subscriber}
* cancels the flow (making {@link FlowableEmitter#isCancelled} return {@code true}),
* other observers subscribed to the same returned {@code Flowable} are not affected.
*
* You should call the {@link FlowableEmitter#onNext(Object)}, {@link FlowableEmitter#onError(Throwable)}
* and {@link FlowableEmitter#onComplete()} methods in a serialized fashion. The
* rest of its methods are thread-safe.
*
* - Backpressure:
* - The backpressure behavior is determined by the {@code mode} parameter.
* - Scheduler:
* - {@code create} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type
* @param source the emitter that is called when a {@code Subscriber} subscribes to the returned {@code Flowable}
* @param mode the backpressure mode to apply if the downstream {@code Subscriber} doesn't request (fast) enough
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source} or {@code mode} is {@code null}
* @see FlowableOnSubscribe
* @see BackpressureStrategy
* @see Cancellable
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.SPECIAL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable create(@NonNull FlowableOnSubscribe source, @NonNull BackpressureStrategy mode) {
Objects.requireNonNull(source, "source is null");
Objects.requireNonNull(mode, "mode is null");
return RxJavaPlugins.onAssembly(new FlowableCreate<>(source, mode));
}
/**
* Returns a {@code Flowable} that calls a {@link Publisher} factory to create a {@code Publisher} for each new {@link Subscriber}
* that subscribes. That is, for each subscriber, the actual {@code Publisher} that subscriber observes is
* determined by the factory function.
*
*
*
* The defer {@code Subscriber} allows you to defer or delay emitting items from a {@code Publisher} until such time as a
* {@code Subscriber} subscribes to the {@code Publisher}. This allows a {@code Subscriber} to easily obtain updates or a
* refreshed version of the sequence.
*
* - Backpressure:
* - The operator itself doesn't interfere with backpressure which is determined by the {@code Publisher}
* returned by the {@code supplier}.
* - Scheduler:
* - {@code defer} does not operate by default on a particular {@link Scheduler}.
*
*
* @param supplier
* the {@code Publisher} factory function to invoke for each {@code Subscriber} that subscribes to the
* resulting {@code Flowable}
* @param
* the type of the items emitted by the {@code Publisher}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code supplier} is {@code null}
* @see ReactiveX operators documentation: Defer
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable defer(@NonNull Supplier extends @NonNull Publisher extends T>> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return RxJavaPlugins.onAssembly(new FlowableDefer<>(supplier));
}
/**
* Returns a {@code Flowable} that emits no items to the {@link Subscriber} and immediately invokes its
* {@link Subscriber#onComplete onComplete} method.
*
*
*
* - Backpressure:
* - This source doesn't produce any elements and effectively ignores downstream backpressure.
* - Scheduler:
* - {@code empty} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the type of the items (ostensibly) emitted by the {@link Publisher}
* @return the shared {@code Flowable} instance
* @see ReactiveX operators documentation: Empty
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings("unchecked")
@NonNull
public static <@NonNull T> Flowable empty() {
return RxJavaPlugins.onAssembly((Flowable) FlowableEmpty.INSTANCE);
}
/**
* Returns a {@code Flowable} that invokes a {@link Subscriber}'s {@link Subscriber#onError onError} method when the
* {@code Subscriber} subscribes to it.
*
*
*
* - Backpressure:
* - This source doesn't produce any elements and effectively ignores downstream backpressure.
* - Scheduler:
* - {@code error} does not operate by default on a particular {@link Scheduler}.
*
*
* @param supplier
* a {@link Supplier} factory to return a {@link Throwable} for each individual {@code Subscriber}
* @param
* the type of the items (ostensibly) emitted by the resulting {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code supplier} is {@code null}
* @see ReactiveX operators documentation: Throw
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable error(@NonNull Supplier extends @NonNull Throwable> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return RxJavaPlugins.onAssembly(new FlowableError<>(supplier));
}
/**
* Returns a {@code Flowable} that invokes a {@link Subscriber}'s {@link Subscriber#onError onError} method when the
* {@code Subscriber} subscribes to it.
*
*
*
* - Backpressure:
* - This source doesn't produce any elements and effectively ignores downstream backpressure.
* - Scheduler:
* - {@code error} does not operate by default on a particular {@link Scheduler}.
*
*
* @param throwable
* the particular {@link Throwable} to pass to {@link Subscriber#onError onError}
* @param
* the type of the items (ostensibly) emitted by the resulting {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code throwable} is {@code null}
* @see ReactiveX operators documentation: Throw
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable error(@NonNull Throwable throwable) {
Objects.requireNonNull(throwable, "throwable is null");
return error(Functions.justSupplier(throwable));
}
/**
* Returns a {@code Flowable} instance that runs the given {@link Action} for each {@link Subscriber} and
* emits either its exception or simply completes.
*
*
*
* - Backpressure:
* - This source doesn't produce any elements and effectively ignores downstream backpressure.
* - Scheduler:
* - {@code fromAction} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@code Action} throws an exception, the respective {@link Throwable} is
* delivered to the downstream via {@link Subscriber#onError(Throwable)},
* except when the downstream has canceled the resulting {@code Flowable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link io.reactivex.rxjava3.exceptions.UndeliverableException UndeliverableException}.
*
*
* @param the target type
* @param action the {@code Action} to run for each {@code Subscriber}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code action} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
public static <@NonNull T> Flowable fromAction(@NonNull Action action) {
Objects.requireNonNull(action, "action is null");
return RxJavaPlugins.onAssembly(new FlowableFromAction<>(action));
}
/**
* Converts an array into a {@link Publisher} that emits the items in the array.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and iterates the given {@code array}
* on demand (i.e., when requested).
* - Scheduler:
* - {@code fromArray} does not operate by default on a particular {@link Scheduler}.
*
*
* @param items
* the array of elements
* @param
* the type of items in the array and the type of items to be emitted by the resulting {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code items} is {@code null}
* @see ReactiveX operators documentation: From
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
public static <@NonNull T> Flowable fromArray(@NonNull T... items) {
Objects.requireNonNull(items, "items is null");
if (items.length == 0) {
return empty();
}
if (items.length == 1) {
return just(items[0]);
}
return RxJavaPlugins.onAssembly(new FlowableFromArray<>(items));
}
/**
* Returns a {@code Flowable} that, when a {@link Subscriber} subscribes to it, invokes a function you specify and then
* emits the value returned from that function.
*
*
*
* This allows you to defer the execution of the function you specify until a {@code Subscriber} subscribes to the
* {@link Publisher}. That is to say, it makes the function "lazy."
*
* - Backpressure:
* - The operator honors backpressure from downstream.
* - Scheduler:
* - {@code fromCallable} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@link Callable} throws an exception, the respective {@link Throwable} is
* delivered to the downstream via {@link Subscriber#onError(Throwable)},
* except when the downstream has canceled this {@code Flowable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link io.reactivex.rxjava3.exceptions.UndeliverableException UndeliverableException}.
*
*
*
* @param callable
* a function, the execution of which should be deferred; {@code fromCallable} will invoke this
* function only when a {@code Subscriber} subscribes to the {@code Publisher} that {@code fromCallable} returns
* @param
* the type of the item emitted by the {@code Publisher}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code callable} is {@code null}
* @see #defer(Supplier)
* @see #fromSupplier(Supplier)
* @since 2.0
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable fromCallable(@NonNull Callable extends T> callable) {
Objects.requireNonNull(callable, "callable is null");
return RxJavaPlugins.onAssembly(new FlowableFromCallable<>(callable));
}
/**
* Wraps a {@link CompletableSource} into a {@code Flowable}.
*
*
*
* - Backpressure:
* - This source doesn't produce any elements and effectively ignores downstream backpressure.
* - Scheduler:
* - {@code fromCompletable} does not operate by default on a particular {@link Scheduler}.
*
* @param the target type
* @param completableSource the {@code CompletableSource} to convert from
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code completableSource} is {@code null}
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
public static <@NonNull T> Flowable fromCompletable(@NonNull CompletableSource completableSource) {
Objects.requireNonNull(completableSource, "completableSource is null");
return RxJavaPlugins.onAssembly(new FlowableFromCompletable<>(completableSource));
}
/**
* Converts a {@link Future} into a {@link Publisher}.
*
*
*
* The operator calls {@link Future#get()}, which is a blocking method, on the subscription thread.
* It is recommended applying {@link #subscribeOn(Scheduler)} to move this blocking wait to a
* background thread, and if the {@link Scheduler} supports it, interrupt the wait when the flow
* is disposed.
*
* Also note that this operator will consume a {@link CompletionStage}-based {@code Future} subclass (such as
* {@link CompletableFuture}) in a blocking manner as well. Use the {@link #fromCompletionStage(CompletionStage)}
* operator to convert and consume such sources in a non-blocking fashion instead.
*
* Unlike 1.x, canceling the {@code Flowable} won't cancel the future. If necessary, one can use composition to achieve the
* cancellation effect: {@code futurePublisher.doOnCancel(() -> future.cancel(true));}.
*
* - Backpressure:
* - The operator honors backpressure from downstream.
* - Scheduler:
* - {@code fromFuture} does not operate by default on a particular {@code Scheduler}.
*
*
* @param future
* the source {@code Future}
* @param
* the type of object that the {@code Future} returns, and also the type of item to be emitted by
* the resulting {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code future} is {@code null}
* @see ReactiveX operators documentation: From
* @see #fromCompletionStage(CompletionStage)
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable fromFuture(@NonNull Future extends T> future) {
Objects.requireNonNull(future, "future is null");
return RxJavaPlugins.onAssembly(new FlowableFromFuture<>(future, 0L, null));
}
/**
* Converts a {@link Future} into a {@link Publisher}, with a timeout on the {@code Future}.
*
*
*
* The operator calls {@link Future#get(long, TimeUnit)}, which is a blocking method, on the subscription thread.
* It is recommended applying {@link #subscribeOn(Scheduler)} to move this blocking wait to a
* background thread, and if the {@link Scheduler} supports it, interrupt the wait when the flow
* is disposed.
*
* Unlike 1.x, canceling the {@code Flowable} won't cancel the future. If necessary, one can use composition to achieve the
* cancellation effect: {@code futurePublisher.doOnCancel(() -> future.cancel(true));}.
*
* Also note that this operator will consume a {@link CompletionStage}-based {@code Future} subclass (such as
* {@link CompletableFuture}) in a blocking manner as well. Use the {@link #fromCompletionStage(CompletionStage)}
* operator to convert and consume such sources in a non-blocking fashion instead.
*
* - Backpressure:
* - The operator honors backpressure from downstream.
* - Scheduler:
* - {@code fromFuture} does not operate by default on a particular {@code Scheduler}.
*
*
* @param future
* the source {@code Future}
* @param timeout
* the maximum time to wait before calling {@code get}
* @param unit
* the {@link TimeUnit} of the {@code timeout} argument
* @param
* the type of object that the {@code Future} returns, and also the type of item to be emitted by
* the resulting {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code future} or {@code unit} is {@code null}
* @see ReactiveX operators documentation: From
* @see #fromCompletionStage(CompletionStage)
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable fromFuture(@NonNull Future extends T> future, long timeout, @NonNull TimeUnit unit) {
Objects.requireNonNull(future, "future is null");
Objects.requireNonNull(unit, "unit is null");
return RxJavaPlugins.onAssembly(new FlowableFromFuture<>(future, timeout, unit));
}
/**
* Converts an {@link Iterable} sequence into a {@link Publisher} that emits the items in the sequence.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and iterates the given {@code iterable}
* on demand (i.e., when requested).
* - Scheduler:
* - {@code fromIterable} does not operate by default on a particular {@link Scheduler}.
*
*
* @param source
* the source {@code Iterable} sequence
* @param
* the type of items in the {@code Iterable} sequence and the type of items to be emitted by the
* resulting {@code Flowable}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source} is {@code null}
* @see ReactiveX operators documentation: From
* @see #fromStream(Stream)
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable fromIterable(@NonNull Iterable extends T> source) {
Objects.requireNonNull(source, "source is null");
return RxJavaPlugins.onAssembly(new FlowableFromIterable<>(source));
}
/**
* Returns a {@code Flowable} instance that when subscribed to, subscribes to the {@link MaybeSource} instance and
* emits {@code onSuccess} as a single item or forwards any {@code onComplete} or
* {@code onError} signal.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream.
* - Scheduler:
* - {@code fromMaybe} does not operate by default on a particular {@link Scheduler}.
*
* @param the value type of the {@code MaybeSource} element
* @param maybe the {@code MaybeSource} instance to subscribe to, not {@code null}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code maybe} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
@BackpressureSupport(BackpressureKind.FULL)
public static <@NonNull T> Flowable fromMaybe(@NonNull MaybeSource maybe) {
Objects.requireNonNull(maybe, "maybe is null");
return RxJavaPlugins.onAssembly(new MaybeToFlowable<>(maybe));
}
/**
* Converts the given {@link ObservableSource} into a {@code Flowable} by applying the specified backpressure strategy.
*
* Marble diagrams for the various backpressure strategies are as follows:
*
* - {@link BackpressureStrategy#BUFFER}
*
*
*
* - {@link BackpressureStrategy#DROP}
*
*
*
* - {@link BackpressureStrategy#LATEST}
*
*
*
* - {@link BackpressureStrategy#ERROR}
*
*
*
* - {@link BackpressureStrategy#MISSING}
*
*
*
*
*
* - Backpressure:
* - The operator applies the chosen backpressure strategy of {@link BackpressureStrategy} enum.
* - Scheduler:
* - {@code fromObservable} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the element type of the source and resulting sequence
* @param source the {@code ObservableSource} to convert
* @param strategy the backpressure strategy to apply
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source} or {@code strategy} is {@code null}
*/
@BackpressureSupport(BackpressureKind.SPECIAL)
@CheckReturnValue
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable fromObservable(@NonNull ObservableSource source, @NonNull BackpressureStrategy strategy) {
Objects.requireNonNull(source, "source is null");
Objects.requireNonNull(strategy, "strategy is null");
Flowable f = new FlowableFromObservable<>(source);
switch (strategy) {
case DROP:
return f.onBackpressureDrop();
case LATEST:
return f.onBackpressureLatest();
case MISSING:
return f;
case ERROR:
return RxJavaPlugins.onAssembly(new FlowableOnBackpressureError<>(f));
default:
return f.onBackpressureBuffer();
}
}
/**
* Converts an arbitrary Reactive Streams {@link Publisher} into a {@code Flowable} if not already a
* {@code Flowable}.
*
* The {@code Publisher} must follow the
* Reactive-Streams specification.
* Violating the specification may result in undefined behavior.
*
* If possible, use {@link #create(FlowableOnSubscribe, BackpressureStrategy)} to create a
* source-like {@code Flowable} instead.
*
* Note that even though {@code Publisher} appears to be a functional interface, it
* is not recommended to implement it through a lambda as the specification requires
* state management that is not achievable with a stateless lambda.
*
* - Backpressure:
* - The operator is a pass-through for backpressure and its behavior is determined by the
* backpressure behavior of the wrapped publisher.
* - Scheduler:
* - {@code fromPublisher} does not operate by default on a particular {@link Scheduler}.
*
* @param the value type of the flow
* @param publisher the {@code Publisher} to convert
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code publisher} is {@code null}
* @see #create(FlowableOnSubscribe, BackpressureStrategy)
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
@SchedulerSupport(SchedulerSupport.NONE)
@SuppressWarnings("unchecked")
public static <@NonNull T> Flowable fromPublisher(@NonNull Publisher extends T> publisher) {
if (publisher instanceof Flowable) {
return RxJavaPlugins.onAssembly((Flowable)publisher);
}
Objects.requireNonNull(publisher, "publisher is null");
return RxJavaPlugins.onAssembly(new FlowableFromPublisher<>(publisher));
}
/**
* Returns a {@code Flowable} instance that runs the given {@link Runnable} for each {@link Subscriber} and
* emits either its unchecked exception or simply completes.
*
*
*
* If the code to be wrapped needs to throw a checked or more broader {@link Throwable} exception, that
* exception has to be converted to an unchecked exception by the wrapped code itself. Alternatively,
* use the {@link #fromAction(Action)} method which allows the wrapped code to throw any {@code Throwable}
* exception and will signal it to observers as-is.
*
* - Backpressure:
* - This source doesn't produce any elements and effectively ignores downstream backpressure.
* - Scheduler:
* - {@code fromRunnable} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@code Runnable} throws an exception, the respective {@code Throwable} is
* delivered to the downstream via {@link Subscriber#onError(Throwable)},
* except when the downstream has canceled the resulting {@code Flowable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link io.reactivex.rxjava3.exceptions.UndeliverableException UndeliverableException}.
*
*
* @param the target type
* @param run the {@code Runnable} to run for each {@code Subscriber}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code run} is {@code null}
* @since 3.0.0
* @see #fromAction(Action)
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
@BackpressureSupport(BackpressureKind.PASS_THROUGH)
public static <@NonNull T> Flowable fromRunnable(@NonNull Runnable run) {
Objects.requireNonNull(run, "run is null");
return RxJavaPlugins.onAssembly(new FlowableFromRunnable<>(run));
}
/**
* Returns a {@code Flowable} instance that when subscribed to, subscribes to the {@link SingleSource} instance and
* emits {@code onSuccess} as a single item or forwards the {@code onError} signal.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream.
* - Scheduler:
* - {@code fromSingle} does not operate by default on a particular {@link Scheduler}.
*
* @param the value type of the {@code SingleSource} element
* @param source the {@code SingleSource} instance to subscribe to, not {@code null}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code source} is {@code null}
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@SchedulerSupport(SchedulerSupport.NONE)
@BackpressureSupport(BackpressureKind.FULL)
public static <@NonNull T> Flowable fromSingle(@NonNull SingleSource source) {
Objects.requireNonNull(source, "source is null");
return RxJavaPlugins.onAssembly(new SingleToFlowable<>(source));
}
/**
* Returns a {@code Flowable} that, when a {@link Subscriber} subscribes to it, invokes a supplier function you specify and then
* emits the value returned from that function.
*
*
*
* This allows you to defer the execution of the function you specify until a {@code Subscriber} subscribes to the
* {@link Publisher}. That is to say, it makes the function "lazy."
*
* - Backpressure:
* - The operator honors backpressure from downstream.
* - Scheduler:
* - {@code fromSupplier} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If the {@link Supplier} throws an exception, the respective {@link Throwable} is
* delivered to the downstream via {@link Subscriber#onError(Throwable)},
* except when the downstream has canceled this {@code Flowable} source.
* In this latter case, the {@code Throwable} is delivered to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} as an {@link io.reactivex.rxjava3.exceptions.UndeliverableException UndeliverableException}.
*
*
*
* @param supplier
* a function, the execution of which should be deferred; {@code fromSupplier} will invoke this
* function only when a {@code Subscriber} subscribes to the {@code Publisher} that {@code fromSupplier} returns
* @param
* the type of the item emitted by the {@code Publisher}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code supplier} is {@code null}
* @see #defer(Supplier)
* @see #fromCallable(Callable)
* @since 3.0.0
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable fromSupplier(@NonNull Supplier extends T> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return RxJavaPlugins.onAssembly(new FlowableFromSupplier<>(supplier));
}
/**
* Returns a cold, synchronous, stateless and backpressure-aware generator of values.
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Backpressure:
* - The operator honors downstream backpressure.
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the generated value type
* @param generator the {@link Consumer} called whenever a particular downstream {@link Subscriber} has
* requested a value. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code generator} is {@code null}
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable generate(@NonNull Consumer<@NonNull Emitter> generator) {
Objects.requireNonNull(generator, "generator is null");
return generate(Functions.nullSupplier(),
FlowableInternalHelper.simpleGenerator(generator),
Functions.emptyConsumer());
}
/**
* Returns a cold, synchronous, stateful and backpressure-aware generator of values.
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Backpressure:
* - The operator honors downstream backpressure.
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Subscriber} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Subscriber}
* @param generator the {@link Consumer} called with the current state whenever a particular downstream {@code Subscriber} has
* requested a value. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code initialState} or {@code generator} is {@code null}
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull S> Flowable generate(@NonNull Supplier initialState, @NonNull BiConsumer> generator) {
Objects.requireNonNull(generator, "generator is null");
return generate(initialState, FlowableInternalHelper.simpleBiGenerator(generator),
Functions.emptyConsumer());
}
/**
* Returns a cold, synchronous, stateful and backpressure-aware generator of values.
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Backpressure:
* - The operator honors downstream backpressure.
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Subscriber} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Subscriber}
* @param generator the {@link Consumer} called with the current state whenever a particular downstream {@code Subscriber} has
* requested a value. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @param disposeState the {@code Consumer} that is called with the current state when the generator
* terminates the sequence or it gets canceled
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code initialState}, {@code generator} or {@code disposeState} is {@code null}
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull S> Flowable generate(@NonNull Supplier initialState, @NonNull BiConsumer> generator,
@NonNull Consumer super S> disposeState) {
Objects.requireNonNull(generator, "generator is null");
return generate(initialState, FlowableInternalHelper.simpleBiGenerator(generator), disposeState);
}
/**
* Returns a cold, synchronous, stateful and backpressure-aware generator of values.
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Backpressure:
* - The operator honors downstream backpressure.
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Subscriber} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Subscriber}
* @param generator the {@link Function} called with the current state whenever a particular downstream {@code Subscriber} has
* requested a value. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event and should return a (new) state for
* the next invocation. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code initialState} or {@code generator} is {@code null}
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T, @NonNull S> Flowable generate(@NonNull Supplier initialState, @NonNull BiFunction, S> generator) {
return generate(initialState, generator, Functions.emptyConsumer());
}
/**
* Returns a cold, synchronous, stateful and backpressure-aware generator of values.
*
* Note that the {@link Emitter#onNext}, {@link Emitter#onError} and
* {@link Emitter#onComplete} methods provided to the function via the {@link Emitter} instance should be called synchronously,
* never concurrently and only while the function body is executing. Calling them from multiple threads
* or outside the function call is not supported and leads to an undefined behavior.
*
* - Backpressure:
* - The operator honors downstream backpressure.
* - Scheduler:
* - {@code generate} does not operate by default on a particular {@link Scheduler}.
*
*
* @param the type of the per-{@link Subscriber} state
* @param the generated value type
* @param initialState the {@link Supplier} to generate the initial state for each {@code Subscriber}
* @param generator the {@link Function} called with the current state whenever a particular downstream {@code Subscriber} has
* requested a value. The callback then should call {@code onNext}, {@code onError} or
* {@code onComplete} to signal a value or a terminal event and should return a (new) state for
* the next invocation. Signaling multiple {@code onNext}
* in a call will make the operator signal {@link IllegalStateException}.
* @param disposeState the {@link Consumer} that is called with the current state when the generator
* terminates the sequence or it gets canceled
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code initialState}, {@code generator} or {@code disposeState} is {@code null}
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T, @NonNull S> Flowable generate(@NonNull Supplier initialState, @NonNull BiFunction, S> generator, @NonNull Consumer super S> disposeState) {
Objects.requireNonNull(initialState, "initialState is null");
Objects.requireNonNull(generator, "generator is null");
Objects.requireNonNull(disposeState, "disposeState is null");
return RxJavaPlugins.onAssembly(new FlowableGenerate<>(initialState, generator, disposeState));
}
/**
* Returns a {@code Flowable} that emits a {@code 0L} after the {@code initialDelay} and ever-increasing numbers
* after each {@code period} of time thereafter.
*
*
*
* - Backpressure:
* - The operator generates values based on time and ignores downstream backpressure which
* may lead to {@link MissingBackpressureException} at some point in the chain.
* Downstream consumers should consider applying one of the {@code onBackpressureXXX} operators as well.
* - Scheduler:
* - {@code interval} operates by default on the {@code computation} {@link Scheduler}.
*
*
* @param initialDelay
* the initial delay time to wait before emitting the first value of 0L
* @param period
* the period of time between emissions of the subsequent numbers
* @param unit
* the time unit for both {@code initialDelay} and {@code period}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code unit} is {@code null}
* @see ReactiveX operators documentation: Interval
* @since 1.0.12
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.ERROR)
@SchedulerSupport(SchedulerSupport.COMPUTATION)
@NonNull
public static Flowable interval(long initialDelay, long period, @NonNull TimeUnit unit) {
return interval(initialDelay, period, unit, Schedulers.computation());
}
/**
* Returns a {@code Flowable} that emits a {@code 0L} after the {@code initialDelay} and ever-increasing numbers
* after each {@code period} of time thereafter, on a specified {@link Scheduler}.
*
*
*
* - Backpressure:
* - The operator generates values based on time and ignores downstream backpressure which
* may lead to {@link MissingBackpressureException} at some point in the chain.
* Downstream consumers should consider applying one of the {@code onBackpressureXXX} operators as well.
* - Scheduler:
* - You specify which {@code Scheduler} this operator will use.
*
*
* @param initialDelay
* the initial delay time to wait before emitting the first value of 0L
* @param period
* the period of time between emissions of the subsequent numbers
* @param unit
* the time unit for both {@code initialDelay} and {@code period}
* @param scheduler
* the {@code Scheduler} on which the waiting happens and items are emitted
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code unit} or {@code scheduler} is {@code null}
* @see ReactiveX operators documentation: Interval
* @since 1.0.12
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.ERROR)
@SchedulerSupport(SchedulerSupport.CUSTOM)
public static Flowable interval(long initialDelay, long period, @NonNull TimeUnit unit, @NonNull Scheduler scheduler) {
Objects.requireNonNull(unit, "unit is null");
Objects.requireNonNull(scheduler, "scheduler is null");
return RxJavaPlugins.onAssembly(new FlowableInterval(Math.max(0L, initialDelay), Math.max(0L, period), unit, scheduler));
}
/**
* Returns a {@code Flowable} that emits a sequential number every specified interval of time.
*
*
*
* - Backpressure:
* - The operator signals a {@link MissingBackpressureException} if the downstream
* is not ready to receive the next value.
* - Scheduler:
* - {@code interval} operates by default on the {@code computation} {@link Scheduler}.
*
*
* @param period
* the period size in time units (see below)
* @param unit
* time units to use for the interval size
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code unit} is {@code null}
* @see ReactiveX operators documentation: Interval
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.ERROR)
@SchedulerSupport(SchedulerSupport.COMPUTATION)
@NonNull
public static Flowable interval(long period, @NonNull TimeUnit unit) {
return interval(period, period, unit, Schedulers.computation());
}
/**
* Returns a {@code Flowable} that emits a sequential number every specified interval of time, on a
* specified {@link Scheduler}.
*
*
*
* - Backpressure:
* - The operator generates values based on time and ignores downstream backpressure which
* may lead to {@link MissingBackpressureException} at some point in the chain.
* Downstream consumers should consider applying one of the {@code onBackpressureXXX} operators as well.
* - Scheduler:
* - You specify which {@code Scheduler} this operator will use.
*
*
* @param period
* the period size in time units (see below)
* @param unit
* time units to use for the interval size
* @param scheduler
* the {@code Scheduler} to use for scheduling the items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code unit} or {@code scheduler} is {@code null}
* @see ReactiveX operators documentation: Interval
*/
@CheckReturnValue
@BackpressureSupport(BackpressureKind.ERROR)
@SchedulerSupport(SchedulerSupport.CUSTOM)
@NonNull
public static Flowable interval(long period, @NonNull TimeUnit unit, @NonNull Scheduler scheduler) {
return interval(period, period, unit, scheduler);
}
/**
* Signals a range of long values, the first after some initial delay and the rest periodically after.
*
* The sequence completes immediately after the last value {@code (start + count - 1)} has been reached.
*
* - Backpressure:
* - The operator signals a {@link MissingBackpressureException} if the downstream can't keep up.
* - Scheduler:
* - {@code intervalRange} by default operates on the {@link Schedulers#computation() computation} {@link Scheduler}.
*
* @param start that start value of the range
* @param count the number of values to emit in total, if zero, the operator emits an {@code onComplete} after the initial delay.
* @param initialDelay the initial delay before signaling the first value (the start)
* @param period the period between subsequent values
* @param unit the unit of measure of the {@code initialDelay} and {@code period} amounts
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code unit} is {@code null}
* @throws IllegalArgumentException
* if {@code count} is less than zero, or if {@code start} + {@code count} − 1 exceeds
* {@link Long#MAX_VALUE}
* @see #range(int, int)
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.ERROR)
@SchedulerSupport(SchedulerSupport.COMPUTATION)
public static Flowable intervalRange(long start, long count, long initialDelay, long period, @NonNull TimeUnit unit) {
return intervalRange(start, count, initialDelay, period, unit, Schedulers.computation());
}
/**
* Signals a range of long values, the first after some initial delay and the rest periodically after.
*
* The sequence completes immediately after the last value (start + count - 1) has been reached.
*
* - Backpressure:
* - The operator signals a {@link MissingBackpressureException} if the downstream can't keep up.
* - Scheduler:
* - you provide the {@link Scheduler}.
*
* @param start that start value of the range
* @param count the number of values to emit in total, if zero, the operator emits an {@code onComplete} after the initial delay.
* @param initialDelay the initial delay before signaling the first value (the start)
* @param period the period between subsequent values
* @param unit the unit of measure of the {@code initialDelay} and {@code period} amounts
* @param scheduler the target {@code Scheduler} where the values and terminal signals will be emitted
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code unit} or {@code scheduler} is {@code null}
* @throws IllegalArgumentException
* if {@code count} is less than zero, or if {@code start} + {@code count} − 1 exceeds
* {@link Long#MAX_VALUE}
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.ERROR)
@SchedulerSupport(SchedulerSupport.CUSTOM)
public static Flowable intervalRange(long start, long count, long initialDelay, long period, @NonNull TimeUnit unit, @NonNull Scheduler scheduler) {
if (count < 0L) {
throw new IllegalArgumentException("count >= 0 required but it was " + count);
}
if (count == 0L) {
return Flowable.empty().delay(initialDelay, unit, scheduler);
}
long end = start + (count - 1);
if (start > 0 && end < 0) {
throw new IllegalArgumentException("Overflow! start + count is bigger than Long.MAX_VALUE");
}
Objects.requireNonNull(unit, "unit is null");
Objects.requireNonNull(scheduler, "scheduler is null");
return RxJavaPlugins.onAssembly(new FlowableIntervalRange(start, end, Math.max(0L, initialDelay), Math.max(0L, period), unit, scheduler));
}
/**
* Returns a {@code Flowable} that signals the given (constant reference) item and then completes.
*
*
*
* Note that the item is taken and re-emitted as is and not computed by any means by {@code just}. Use {@link #fromCallable(Callable)}
* to generate a single item on demand (when {@link Subscriber}s subscribe to it).
*
* See the multi-parameter overloads of {@code just} to emit more than one (constant reference) items one after the other.
* Use {@link #fromArray(Object...)} to emit an arbitrary number of items that are known upfront.
*
* To emit the items of an {@link Iterable} sequence (such as a {@link java.util.List}), use {@link #fromIterable(Iterable)}.
*
* - Backpressure:
* - The operator honors backpressure from downstream.
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item
* the item to emit
* @param
* the type of that item
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item} is {@code null}
* @see ReactiveX operators documentation: Just
* @see #just(Object, Object)
* @see #fromCallable(Callable)
* @see #fromArray(Object...)
* @see #fromIterable(Iterable)
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item) {
Objects.requireNonNull(item, "item is null");
return RxJavaPlugins.onAssembly(new FlowableJust<>(item));
}
/**
* Converts two items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1} or {@code item2} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
return fromArray(item1, item2);
}
/**
* Converts three items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2} or {@code item3} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
return fromArray(item1, item2, item3);
}
/**
* Converts four items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* or {@code item4} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3, T item4) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
return fromArray(item1, item2, item3, item4);
}
/**
* Converts five items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4} or {@code item5} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3, T item4, T item5) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
return fromArray(item1, item2, item3, item4, item5);
}
/**
* Converts six items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5} or {@code item6} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3, T item4, T item5, T item6) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
return fromArray(item1, item2, item3, item4, item5, item6);
}
/**
* Converts seven items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6}
* or {@code item7} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3, T item4, T item5, T item6, T item7) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7);
}
/**
* Converts eight items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param item8
* eighth item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6},
* {@code item7} or {@code item8} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3, T item4, T item5, T item6, T item7, T item8) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
Objects.requireNonNull(item8, "item8 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7, item8);
}
/**
* Converts nine items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param item8
* eighth item
* @param item9
* ninth item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6},
* {@code item7}, {@code item8} or {@code item9} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3, T item4, T item5, T item6, T item7, T item8, T item9) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
Objects.requireNonNull(item8, "item8 is null");
Objects.requireNonNull(item9, "item9 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7, item8, item9);
}
/**
* Converts ten items into a {@link Publisher} that emits those items.
*
*
*
* - Backpressure:
* - The operator honors backpressure from downstream and signals each value on-demand (i.e., when requested).
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param item1
* first item
* @param item2
* second item
* @param item3
* third item
* @param item4
* fourth item
* @param item5
* fifth item
* @param item6
* sixth item
* @param item7
* seventh item
* @param item8
* eighth item
* @param item9
* ninth item
* @param item10
* tenth item
* @param
* the type of these items
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code item1}, {@code item2}, {@code item3},
* {@code item4}, {@code item5}, {@code item6},
* {@code item7}, {@code item8}, {@code item9},
* or {@code item10} is {@code null}
* @see ReactiveX operators documentation: Just
*/
@CheckReturnValue
@NonNull
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
public static <@NonNull T> Flowable just(T item1, T item2, T item3, T item4, T item5, T item6, T item7, T item8, T item9, T item10) {
Objects.requireNonNull(item1, "item1 is null");
Objects.requireNonNull(item2, "item2 is null");
Objects.requireNonNull(item3, "item3 is null");
Objects.requireNonNull(item4, "item4 is null");
Objects.requireNonNull(item5, "item5 is null");
Objects.requireNonNull(item6, "item6 is null");
Objects.requireNonNull(item7, "item7 is null");
Objects.requireNonNull(item8, "item8 is null");
Objects.requireNonNull(item9, "item9 is null");
Objects.requireNonNull(item10, "item10 is null");
return fromArray(item1, item2, item3, item4, item5, item6, item7, item8, item9, item10);
}
/**
* Flattens an {@link Iterable} of {@link Publisher}s into one {@code Publisher}, without any transformation, while limiting the
* number of concurrent subscriptions to these {@code Publisher}s.
*
*
*
* You can combine the items emitted by multiple {@code Publisher}s so that they appear as a single {@code Publisher}, by
* using the {@code merge} method.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The source {@code Publisher}s are expected to honor
* backpressure; if violated, the operator may signal {@link MissingBackpressureException}.
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the source {@code Publisher}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Flowable} terminates with that {@code Throwable} and all other source {@code Publisher}s are canceled.
* If more than one {@code Publisher} signals an error, the resulting {@code Flowable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Flowable} has been canceled or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeDelayError(Iterable, int, int)} to merge sources and terminate only when all source {@code Publisher}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the {@code Iterable} of {@code Publisher}s
* @param maxConcurrency
* the maximum number of {@code Publisher}s that may be subscribed to concurrently
* @param bufferSize
* the number of items to prefetch from each inner {@code Publisher}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException
* if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: Merge
* @see #mergeDelayError(Iterable, int, int)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable merge(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources, int maxConcurrency, int bufferSize) {
return fromIterable(sources).flatMap((Function)Functions.identity(), false, maxConcurrency, bufferSize);
}
/**
* Flattens an array of {@link Publisher}s into one {@code Publisher}, without any transformation, while limiting the
* number of concurrent subscriptions to these {@code Publisher}s.
*
*
*
* You can combine the items emitted by multiple {@code Publisher}s so that they appear as a single {@code Publisher}, by
* using the {@code merge} method.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The source {@code Publisher}s are expected to honor
* backpressure; if violated, the operator may signal {@link MissingBackpressureException}.
* - Scheduler:
* - {@code mergeArray} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the source {@code Publisher}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Flowable} terminates with that {@code Throwable} and all other source {@code Publisher}s are canceled.
* If more than one {@code Publisher} signals an error, the resulting {@code Flowable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Flowable} has been canceled or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeArrayDelayError(int, int, Publisher[])} to merge sources and terminate only when all source {@code Publisher}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the array of {@code Publisher}s
* @param maxConcurrency
* the maximum number of {@code Publisher}s that may be subscribed to concurrently
* @param bufferSize
* the number of items to prefetch from each inner {@code Publisher}
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException
* if {@code maxConcurrency} or {@code bufferSize} is non-positive
* @see ReactiveX operators documentation: Merge
* @see #mergeArrayDelayError(int, int, Publisher...)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@SafeVarargs
@NonNull
public static <@NonNull T> Flowable mergeArray(int maxConcurrency, int bufferSize, @NonNull Publisher extends T>... sources) {
return fromArray(sources).flatMap((Function)Functions.identity(), false, maxConcurrency, bufferSize);
}
/**
* Flattens an {@link Iterable} of {@link Publisher}s into one {@code Publisher}, without any transformation.
*
*
*
* You can combine the items emitted by multiple {@code Publisher}s so that they appear as a single {@code Publisher}, by
* using the {@code merge} method.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The source {@code Publisher}s are expected to honor
* backpressure; if violated, the operator may signal {@link MissingBackpressureException}.
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the source {@code Publisher}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Flowable} terminates with that {@code Throwable} and all other source {@code Publisher}s are canceled.
* If more than one {@code Publisher} signals an error, the resulting {@code Flowable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Flowable} has been canceled or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeDelayError(Iterable)} to merge sources and terminate only when all source {@code Publisher}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the {@code Iterable} of {@code Publisher}s
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @see ReactiveX operators documentation: Merge
* @see #mergeDelayError(Iterable)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable merge(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources) {
return fromIterable(sources).flatMap((Function)Functions.identity());
}
/**
* Flattens an {@link Iterable} of {@link Publisher}s into one {@code Publisher}, without any transformation, while limiting the
* number of concurrent subscriptions to these {@code Publisher}s.
*
*
*
* You can combine the items emitted by multiple {@code Publisher}s so that they appear as a single {@code Publisher}, by
* using the {@code merge} method.
*
* - Backpressure:
* - The operator honors backpressure from downstream. The source {@code Publisher}s are expected to honor
* backpressure; if violated, the operator may signal {@link MissingBackpressureException}.
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
* - Error handling:
* - If any of the source {@code Publisher}s signal a {@link Throwable} via {@code onError}, the resulting
* {@code Flowable} terminates with that {@code Throwable} and all other source {@code Publisher}s are canceled.
* If more than one {@code Publisher} signals an error, the resulting {@code Flowable} may terminate with the
* first one's error or, depending on the concurrency of the sources, may terminate with a
* {@link CompositeException} containing two or more of the various error signals.
* {@code Throwable}s that didn't make into the composite will be sent (individually) to the global error handler via
* {@link RxJavaPlugins#onError(Throwable)} method as {@link UndeliverableException} errors. Similarly, {@code Throwable}s
* signaled by source(s) after the returned {@code Flowable} has been canceled or terminated with a
* (composite) error will be sent to the same global error handler.
* Use {@link #mergeDelayError(Iterable, int)} to merge sources and terminate only when all source {@code Publisher}s
* have completed or failed with an error.
*
*
*
* @param the common element base type
* @param sources
* the {@code Iterable} of {@code Publisher}s
* @param maxConcurrency
* the maximum number of {@code Publisher}s that may be subscribed to concurrently
* @return the new {@code Flowable} instance
* @throws NullPointerException if {@code sources} is {@code null}
* @throws IllegalArgumentException
* if {@code maxConcurrency} is less than or equal to 0
* @see ReactiveX operators documentation: Merge
* @see #mergeDelayError(Iterable, int)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@CheckReturnValue
@BackpressureSupport(BackpressureKind.FULL)
@SchedulerSupport(SchedulerSupport.NONE)
@NonNull
public static <@NonNull T> Flowable merge(@NonNull Iterable<@NonNull ? extends Publisher extends T>> sources, int maxConcurrency) {
return fromIterable(sources).flatMap((Function)Functions.identity(), maxConcurrency);
}
/**
* Flattens a {@link Publisher} that emits {@code Publisher}s into a single {@code Publisher} that emits the items emitted by
* thos {@code Publisher}s , without any transformation.
*
*
*
* You can combine the items emitted by multiple {@code Publisher}s so that they appear as a single {@code Publisher}, by
* using the {@code merge} method.
*
*