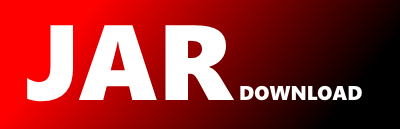
rx.internal.operators.OperatorMapNotification Maven / Gradle / Ivy
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.internal.operators;
import java.util.Queue;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.atomic.AtomicLong;
import rx.*;
import rx.Observable.Operator;
import rx.exceptions.*;
import rx.functions.*;
import rx.internal.producers.ProducerArbiter;
import rx.internal.util.unsafe.*;
/**
* Applies a function of your choosing to every item emitted by an {@code Observable}, and emits the results of
* this transformation as a new {@code Observable}.
*
*
*/
public final class OperatorMapNotification implements Operator {
private final Func1 super T, ? extends R> onNext;
private final Func1 super Throwable, ? extends R> onError;
private final Func0 extends R> onCompleted;
public OperatorMapNotification(Func1 super T, ? extends R> onNext, Func1 super Throwable, ? extends R> onError, Func0 extends R> onCompleted) {
this.onNext = onNext;
this.onError = onError;
this.onCompleted = onCompleted;
}
@Override
public Subscriber super T> call(final Subscriber super R> o) {
final ProducerArbiter pa = new ProducerArbiter();
MapNotificationSubscriber subscriber = new MapNotificationSubscriber(pa, o);
o.add(subscriber);
subscriber.init();
return subscriber;
}
final class MapNotificationSubscriber extends Subscriber {
private final Subscriber super R> o;
private final ProducerArbiter pa;
final SingleEmitter emitter;
private MapNotificationSubscriber(ProducerArbiter pa, Subscriber super R> o) {
this.pa = pa;
this.o = o;
this.emitter = new SingleEmitter(o, pa, this);
}
void init() {
o.setProducer(emitter);
}
@Override
public void setProducer(Producer producer) {
pa.setProducer(producer);
}
@Override
public void onCompleted() {
try {
emitter.offerAndComplete(onCompleted.call());
} catch (Throwable e) {
Exceptions.throwOrReport(e, o);
}
}
@Override
public void onError(Throwable e) {
try {
emitter.offerAndComplete(onError.call(e));
} catch (Throwable e2) {
Exceptions.throwOrReport(e2, o);
}
}
@Override
public void onNext(T t) {
try {
emitter.offer(onNext.call(t));
} catch (Throwable e) {
Exceptions.throwOrReport(e, o, t);
}
}
}
static final class SingleEmitter extends AtomicLong implements Producer, Subscription {
/** */
private static final long serialVersionUID = -249869671366010660L;
final NotificationLite nl;
final Subscriber super T> child;
final Producer producer;
final Subscription cancel;
final Queue