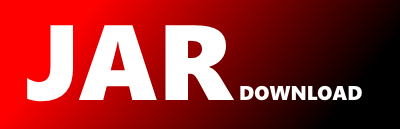
rx.internal.operators.OperatorTakeLastTimed Maven / Gradle / Ivy
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.internal.operators;
import java.util.ArrayDeque;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicLong;
import rx.*;
import rx.Observable.Operator;
import rx.functions.Func1;
/**
* Returns an Observable that emits the last count
items emitted by the source Observable.
*
*
*
* @param the value type
*/
public final class OperatorTakeLastTimed implements Operator {
final long ageMillis;
final Scheduler scheduler;
final int count;
public OperatorTakeLastTimed(long time, TimeUnit unit, Scheduler scheduler) {
this.ageMillis = unit.toMillis(time);
this.scheduler = scheduler;
this.count = -1;
}
public OperatorTakeLastTimed(int count, long time, TimeUnit unit, Scheduler scheduler) {
if (count < 0) {
throw new IndexOutOfBoundsException("count could not be negative");
}
this.ageMillis = unit.toMillis(time);
this.scheduler = scheduler;
this.count = count;
}
@Override
public Subscriber super T> call(final Subscriber super T> subscriber) {
final TakeLastTimedSubscriber parent = new TakeLastTimedSubscriber(subscriber, count, ageMillis, scheduler);
subscriber.add(parent);
subscriber.setProducer(new Producer() {
@Override
public void request(long n) {
parent.requestMore(n);
}
});
return parent;
}
static final class TakeLastTimedSubscriber extends Subscriber implements Func1