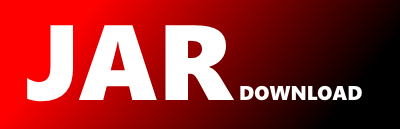
rx.internal.operators.OperatorTimeoutWithSelector Maven / Gradle / Ivy
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.internal.operators;
import rx.*;
import rx.exceptions.Exceptions;
import rx.functions.*;
import rx.schedulers.Schedulers;
import rx.subscriptions.Subscriptions;
/**
* Returns an Observable that mirrors the source Observable. If either the first
* item emitted by the source Observable or any subsequent item don't arrive
* within time windows defined by provided Observables, switch to the
* other
Observable if provided, or emit a TimeoutException .
* @param the value type of the main Observable
* @param the value type of the first timeout Observable
* @param the value type of the subsequent timeout Observable
*/
public class OperatorTimeoutWithSelector extends
OperatorTimeoutBase {
public OperatorTimeoutWithSelector(
final Func0 extends Observable> firstTimeoutSelector,
final Func1 super T, ? extends Observable> timeoutSelector,
Observable extends T> other) {
super(new FirstTimeoutStub() {
@Override
public Subscription call(
final TimeoutSubscriber timeoutSubscriber,
final Long seqId, Scheduler.Worker inner) {
if (firstTimeoutSelector != null) {
Observable o;
try {
o = firstTimeoutSelector.call();
} catch (Throwable t) {
Exceptions.throwOrReport(t, timeoutSubscriber);
return Subscriptions.unsubscribed();
}
return o.unsafeSubscribe(new Subscriber() {
@Override
public void onCompleted() {
timeoutSubscriber.onTimeout(seqId);
}
@Override
public void onError(Throwable e) {
timeoutSubscriber.onError(e);
}
@Override
public void onNext(U t) {
timeoutSubscriber.onTimeout(seqId);
}
});
} else {
return Subscriptions.unsubscribed();
}
}
}, new TimeoutStub() {
@Override
public Subscription call(
final TimeoutSubscriber timeoutSubscriber,
final Long seqId, T value, Scheduler.Worker inner) {
Observable o;
try {
o = timeoutSelector.call(value);
} catch (Throwable t) {
Exceptions.throwOrReport(t, timeoutSubscriber);
return Subscriptions.unsubscribed();
}
return o.unsafeSubscribe(new Subscriber() {
@Override
public void onCompleted() {
timeoutSubscriber.onTimeout(seqId);
}
@Override
public void onError(Throwable e) {
timeoutSubscriber.onError(e);
}
@Override
public void onNext(V t) {
timeoutSubscriber.onTimeout(seqId);
}
});
}
}, other, Schedulers.immediate());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy