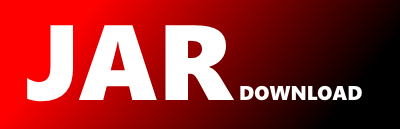
rx.internal.util.atomic.MpscLinkedAtomicQueue Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Original License: https://github.com/JCTools/JCTools/blob/master/LICENSE
* Original location: https://github.com/JCTools/JCTools/blob/master/jctools-core/src/main/java/org/jctools/queues/atomic/MpscLinkedAtomicQueue.java
*/
package rx.internal.util.atomic;
/**
* This is a direct Java port of the MPSC algorithm as presented on 1024
* Cores by D. Vyukov. The original has been adapted to Java and it's quirks with regards to memory model and
* layout:
*
* - Use XCHG functionality provided by AtomicReference (which is better in JDK 8+).
*
* The queue is initialized with a stub node which is set to both the producer and consumer node references. From this
* point follow the notes on offer/poll.
*
* @author nitsanw
*
* @param
*/
public final class MpscLinkedAtomicQueue extends BaseLinkedAtomicQueue {
public MpscLinkedAtomicQueue() {
super();
LinkedQueueNode node = new LinkedQueueNode();
spConsumerNode(node);
xchgProducerNode(node);// this ensures correct construction: StoreLoad
}
/**
* {@inheritDoc}
*
* IMPLEMENTATION NOTES:
* Offer is allowed from multiple threads.
* Offer allocates a new node and:
*
* - Swaps it atomically with current producer node (only one producer 'wins')
*
- Sets the new node as the node following from the swapped producer node
*
* This works because each producer is guaranteed to 'plant' a new node and link the old node. No 2 producers can
* get the same producer node as part of XCHG guarantee.
*
* @see java.util.Queue#offer(java.lang.Object)
*/
@Override
public boolean offer(final E nextValue) {
if (nextValue == null) {
throw new NullPointerException("null elements not allowed");
}
final LinkedQueueNode nextNode = new LinkedQueueNode(nextValue);
final LinkedQueueNode prevProducerNode = xchgProducerNode(nextNode);
// Should a producer thread get interrupted here the chain WILL be broken until that thread is resumed
// and completes the store in prev.next.
prevProducerNode.soNext(nextNode); // StoreStore
return true;
}
/**
* {@inheritDoc}
*
* IMPLEMENTATION NOTES:
* Poll is allowed from a SINGLE thread.
* Poll reads the next node from the consumerNode and:
*
* - If it is null, the queue is assumed empty (though it might not be).
*
- If it is not null set it as the consumer node and return it's now evacuated value.
*
* This means the consumerNode.value is always null, which is also the starting point for the queue. Because null
* values are not allowed to be offered this is the only node with it's value set to null at any one time.
*
* @see java.util.Queue#poll()
*/
@Override
public E poll() {
LinkedQueueNode currConsumerNode = lpConsumerNode(); // don't load twice, it's alright
LinkedQueueNode nextNode = currConsumerNode.lvNext();
if (nextNode != null) {
// we have to null out the value because we are going to hang on to the node
final E nextValue = nextNode.getAndNullValue();
spConsumerNode(nextNode);
return nextValue;
}
else if (currConsumerNode != lvProducerNode()) {
// spin, we are no longer wait free
while ((nextNode = currConsumerNode.lvNext()) == null) { } // NOPMD
// got the next node...
// we have to null out the value because we are going to hang on to the node
final E nextValue = nextNode.getAndNullValue();
spConsumerNode(nextNode);
return nextValue;
}
return null;
}
@Override
public E peek() {
LinkedQueueNode currConsumerNode = lpConsumerNode(); // don't load twice, it's alright
LinkedQueueNode nextNode = currConsumerNode.lvNext();
if (nextNode != null) {
return nextNode.lpValue();
}
else if (currConsumerNode != lvProducerNode()) {
// spin, we are no longer wait free
while ((nextNode = currConsumerNode.lvNext()) == null) { } // NOPMD
// got the next node...
return nextNode.lpValue();
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy