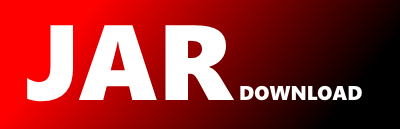
commonMain.io.realm.kotlin.internal.dynamic.DynamicUnmanagedRealmObject.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of library-base-jvm Show documentation
Show all versions of library-base-jvm Show documentation
Library code for Realm Kotlin. This artifact is not supposed to be consumed directly, but through 'io.realm.kotlin:gradle-plugin:1.11.1' instead.
@file:Suppress("UNCHECKED_CAST")
package io.realm.kotlin.internal.dynamic
import io.realm.kotlin.dynamic.DynamicMutableRealmObject
import io.realm.kotlin.dynamic.DynamicRealmObject
import io.realm.kotlin.ext.realmDictionaryOf
import io.realm.kotlin.ext.realmListOf
import io.realm.kotlin.ext.realmSetOf
import io.realm.kotlin.internal.RealmObjectInternal
import io.realm.kotlin.internal.RealmObjectReference
import io.realm.kotlin.query.RealmResults
import io.realm.kotlin.types.BaseRealmObject
import io.realm.kotlin.types.RealmDictionary
import io.realm.kotlin.types.RealmList
import io.realm.kotlin.types.RealmSet
import kotlin.reflect.KClass
internal class DynamicUnmanagedRealmObject(
override val type: String,
properties: Map
) : DynamicMutableRealmObject, RealmObjectInternal {
@Suppress("SpreadOperator")
constructor(type: String, vararg properties: Pair) : this(
type,
mapOf(*properties)
)
val properties: MutableMap = properties.toMutableMap()
override fun getValue(propertyName: String, clazz: KClass): T =
properties[propertyName] as T
override fun getNullableValue(propertyName: String, clazz: KClass): T? =
properties[propertyName] as T?
override fun getObject(propertyName: String): DynamicMutableRealmObject? =
properties[propertyName] as DynamicMutableRealmObject?
override fun getValueList(propertyName: String, clazz: KClass): RealmList =
properties.getOrPut(propertyName) { realmListOf() } as RealmList
override fun getNullableValueList(
propertyName: String,
clazz: KClass
): RealmList = properties.getOrPut(propertyName) { realmListOf() } as RealmList
override fun getObjectList(propertyName: String): RealmList =
properties.getOrPut(propertyName) { realmListOf() }
as RealmList
override fun getValueSet(propertyName: String, clazz: KClass): RealmSet =
properties.getOrPut(propertyName) { realmSetOf() } as RealmSet
override fun getNullableValueSet(
propertyName: String,
clazz: KClass
): RealmSet = properties.getOrPut(propertyName) { realmSetOf() } as RealmSet
override fun getValueDictionary(
propertyName: String,
clazz: KClass
): RealmDictionary =
properties.getOrPut(propertyName) { realmDictionaryOf() } as RealmDictionary
override fun getNullableValueDictionary(
propertyName: String,
clazz: KClass
): RealmDictionary =
properties.getOrPut(propertyName) { realmDictionaryOf() } as RealmDictionary
override fun getBacklinks(propertyName: String): RealmResults =
throw IllegalStateException("Unmanaged dynamic realm objects do not support backlinks.")
override fun getObjectSet(propertyName: String): RealmSet =
properties.getOrPut(propertyName) { realmSetOf() }
as RealmSet
override fun getObjectDictionary(
propertyName: String
): RealmDictionary =
properties.getOrPut(propertyName) { realmDictionaryOf() }
as RealmDictionary
override fun set(propertyName: String, value: T): DynamicMutableRealmObject {
properties[propertyName] = value as Any
return this
}
override var io_realm_kotlin_objectReference: RealmObjectReference? = null
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy