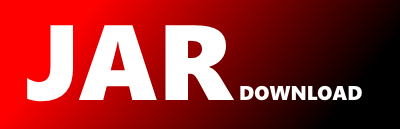
commonMain.io.realm.kotlin.internal.query.ObjectBoundQueries.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of library-base-jvm Show documentation
Show all versions of library-base-jvm Show documentation
Library code for Realm Kotlin. This artifact is not supposed to be consumed directly, but through 'io.realm.kotlin:gradle-plugin:1.11.1' instead.
/*
* Copyright 2022 Realm Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.realm.kotlin.internal.query
import io.realm.kotlin.internal.ObjectBoundRealmResults
import io.realm.kotlin.internal.RealmObjectReference
import io.realm.kotlin.internal.bind
import io.realm.kotlin.notifications.ResultsChange
import io.realm.kotlin.notifications.SingleQueryChange
import io.realm.kotlin.query.RealmQuery
import io.realm.kotlin.query.RealmResults
import io.realm.kotlin.query.RealmScalarNullableQuery
import io.realm.kotlin.query.RealmScalarQuery
import io.realm.kotlin.query.RealmSingleQuery
import io.realm.kotlin.query.Sort
import io.realm.kotlin.types.BaseRealmObject
import kotlinx.coroutines.flow.Flow
import kotlin.reflect.KClass
/**
* This set of classes wraps the queries around the lifecycle of a Realm object, once the
* object is deleted the flow would complete.
*/
internal class ObjectBoundQuery(
val targetObject: RealmObjectReference<*>,
val realmQuery: RealmQuery,
) : RealmQuery by realmQuery {
override fun find(): RealmResults = ObjectBoundRealmResults(
targetObject,
realmQuery.find()
)
override fun query(filter: String, vararg arguments: Any?): RealmQuery = ObjectBoundQuery(
targetObject,
realmQuery.query(filter, *arguments)
)
override fun asFlow(keyPaths: List?): Flow> = realmQuery.asFlow(keyPaths).bind(
targetObject
)
override fun sort(property: String, sortOrder: Sort): RealmQuery = ObjectBoundQuery(
targetObject,
realmQuery.sort(property, sortOrder)
)
override fun sort(
propertyAndSortOrder: Pair,
vararg additionalPropertiesAndOrders: Pair
): RealmQuery = ObjectBoundQuery(
targetObject,
realmQuery.sort(propertyAndSortOrder, *additionalPropertiesAndOrders)
)
override fun distinct(property: String, vararg extraProperties: String): RealmQuery =
ObjectBoundQuery(
targetObject,
realmQuery.distinct(property, *extraProperties)
)
override fun limit(limit: Int): RealmQuery = ObjectBoundQuery(
targetObject,
realmQuery.limit(limit)
)
override fun first(): RealmSingleQuery = ObjectBoundRealmSingleQuery(
targetObject,
realmQuery.first()
)
override fun min(property: String, type: KClass): RealmScalarNullableQuery =
ObjectBoundRealmScalarNullableQuery(
targetObject,
realmQuery.min(property, type)
)
override fun max(property: String, type: KClass): RealmScalarNullableQuery =
ObjectBoundRealmScalarNullableQuery(
targetObject,
realmQuery.max(property, type)
)
override fun sum(property: String, type: KClass): RealmScalarQuery =
ObjectBoundRealmScalarQuery(
targetObject,
realmQuery.sum(property, type)
)
override fun count(): RealmScalarQuery = ObjectBoundRealmScalarQuery(
targetObject,
realmQuery.count()
)
}
internal class ObjectBoundRealmSingleQuery(
val targetObject: RealmObjectReference<*>,
val realmQuery: RealmSingleQuery
) : RealmSingleQuery by realmQuery {
override fun asFlow(keyPaths: List?): Flow> = realmQuery.asFlow(keyPaths).bind(targetObject)
}
internal class ObjectBoundRealmScalarNullableQuery(
val targetObject: RealmObjectReference<*>,
val realmQuery: RealmScalarNullableQuery
) : RealmScalarNullableQuery by realmQuery {
override fun asFlow(): Flow = realmQuery.asFlow().bind(targetObject)
}
internal class ObjectBoundRealmScalarQuery(
val targetObject: RealmObjectReference<*>,
val realmQuery: RealmScalarQuery
) : RealmScalarQuery by realmQuery {
override fun asFlow(): Flow = realmQuery.asFlow().bind(targetObject)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy