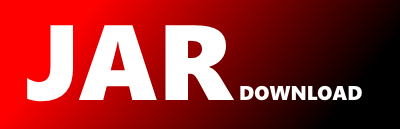
commonMain.io.realm.kotlin.notifications.ResultsChange.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of library-base-jvm Show documentation
Show all versions of library-base-jvm Show documentation
Library code for Realm Kotlin. This artifact is not supposed to be consumed directly, but through 'io.realm.kotlin:gradle-plugin:1.11.1' instead.
/*
* Copyright 2022 Realm Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.realm.kotlin.notifications
import io.realm.kotlin.query.RealmResults
import io.realm.kotlin.types.BaseRealmObject
/**
* This sealed interface describe the possible changes that can happen to a query results collection.
*
* The states are represented by the specific subclasses [InitialResults] and [UpdatedResults].
*
* Changes can thus be consumed in a number of ways:
*
* ```
* // Variant 1: Switch on the sealed interface
* realm.query().asFlow()
* .collect { resultsChange: ResultsChange ->
* when(resultsChange) {
* is InitialResults -> setUIResults(resultsChange.list)
* is UpdatedResults -> updateUIResults(resultsChange) // Android RecyclerView knows how to animate ranges
* }
* }
*
*
* // Variant 2: Just pass on the list
* realm.query().asFlow()
* .collect { resultsChange: ResultsChange ->
* handleChange(resultsChange.list)
* }
* ```
*
* When the query results change, extra information is provided describing the changes from the previous
* version. This information is formatted in a way that can be feed directly to drive animations on UI
* components like `RecyclerView`. In order to access this information, the [ResultsChange] must be cast
* to the appropriate subclass.
*
* ```
* realm.query().asFlow()
* .collect { resultsChange: ResultsChange ->
* when(resultsChange) {
* is InitialResults -> setList(resultsChange.list)
* is UpdatedResults -> { // Automatic cast to UpdatedResults
* updateResults(
* resultsChange.list,
* resultsChange.deletionRanges,
* resultsChange.insertionRanges,
* resultsChange.changeRanges
* )
* }
* }
* }
* ```
*/
public sealed interface ResultsChange {
public val list: RealmResults
}
/**
* Initial event to be emitted on a [RealmResults] flow. It contains a reference to the
* result of the query, the first time it runs.
*/
public interface InitialResults : ResultsChange
/**
* [RealmResults] flow event that describes that an update has happened to elements in the
* observed query. It provides a reference to the new query result and a set of properties that
* describes the changes that happened to the query result.
*/
public interface UpdatedResults : ResultsChange, ListChangeSet
© 2015 - 2024 Weber Informatics LLC | Privacy Policy