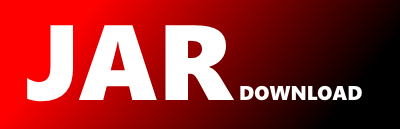
commonMain.io.realm.kotlin.mongodb.internal.AppConfigurationImpl.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of library-sync-jvm Show documentation
Show all versions of library-sync-jvm Show documentation
Sync Library code for Realm Kotlin. This artifact is not supposed to be consumed directly, but through 'io.realm.kotlin:gradle-plugin:1.5.2' instead.
/*
* Copyright 2021 Realm Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.realm.kotlin.mongodb.internal
import io.realm.kotlin.internal.RealmLog
import io.realm.kotlin.internal.interop.CoreLogLevel
import io.realm.kotlin.internal.interop.RealmAppConfigurationPointer
import io.realm.kotlin.internal.interop.RealmInterop
import io.realm.kotlin.internal.interop.RealmSyncClientConfigurationPointer
import io.realm.kotlin.internal.interop.SyncLogCallback
import io.realm.kotlin.internal.interop.sync.MetadataMode
import io.realm.kotlin.internal.interop.sync.NetworkTransport
import io.realm.kotlin.internal.platform.OS_NAME
import io.realm.kotlin.internal.platform.OS_VERSION
import io.realm.kotlin.internal.platform.RUNTIME
import io.realm.kotlin.internal.platform.createDefaultSystemLogger
import io.realm.kotlin.internal.platform.freeze
import io.realm.kotlin.log.LogLevel
import io.realm.kotlin.mongodb.AppConfiguration
import io.realm.kotlin.mongodb.AppConfiguration.Companion.DEFAULT_BASE_URL
// TODO Public due to being a transitive dependency to AppImpl
@Suppress("LongParameterList")
public class AppConfigurationImpl constructor(
override val appId: String,
override val baseUrl: String = DEFAULT_BASE_URL,
override val encryptionKey: ByteArray?,
override val metadataMode: MetadataMode,
override val networkTransport: NetworkTransport,
override val syncRootDirectory: String,
public val log: RealmLog
) : AppConfiguration {
public val nativePointer: RealmAppConfigurationPointer = initializeRealmAppConfig()
public val synClientConfig: RealmSyncClientConfigurationPointer = initializeSyncClientConfig()
override fun equals(other: Any?): Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AppConfigurationImpl
if (appId != (other.appId)) return false
if (baseUrl != (other.baseUrl)) return false
if (metadataMode != (other.metadataMode)) return false
return log == other.log
}
override fun hashCode(): Int {
var result = appId.hashCode()
result = 31 * result + baseUrl.hashCode()
result = 31 * result + metadataMode.hashCode()
result = 31 * result + log.hashCode()
return result
}
// Only freeze anything after all properties are setup as this triggers freezing the actual
// AppConfigurationImpl instance itself
private fun initializeRealmAppConfig(): RealmAppConfigurationPointer =
RealmInterop.realm_app_config_new(
appId = appId,
baseUrl = baseUrl,
networkTransport = RealmInterop.realm_network_transport_new(networkTransport),
platform = "$OS_NAME/$RUNTIME",
platformVersion = OS_VERSION,
sdkVersion = io.realm.kotlin.internal.SDK_VERSION
).freeze()
private fun initializeSyncClientConfig(): RealmSyncClientConfigurationPointer =
RealmInterop.realm_sync_client_config_new()
.also { syncClientConfig ->
// TODO use separate logger for sync or piggyback on config's?
val syncLogger = createDefaultSystemLogger("SYNC", log.logLevel)
// Initialize client configuration first
RealmInterop.realm_sync_client_config_set_log_callback(
syncClientConfig,
object : SyncLogCallback {
override fun log(logLevel: Short, message: String?) {
val coreLogLevel = CoreLogLevel.valueFromPriority(logLevel)
syncLogger.log(LogLevel.fromCoreLogLevel(coreLogLevel), message ?: "")
}
}
)
RealmInterop.realm_sync_client_config_set_log_level(
syncClientConfig,
CoreLogLevel.valueFromPriority(log.logLevel.priority.toShort())
)
RealmInterop.realm_sync_client_config_set_metadata_mode(
syncClientConfig,
metadataMode
)
RealmInterop.realm_sync_client_config_set_base_file_path(
syncClientConfig,
syncRootDirectory
)
if (encryptionKey != null) {
RealmInterop.realm_sync_client_config_set_metadata_encryption_key(
syncClientConfig,
encryptionKey
)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy