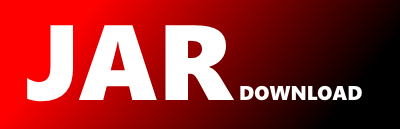
io.redlink.utils.logging.LoggingContext Maven / Gradle / Ivy
/*
* Copyright (c) 2019 Redlink GmbH.
*/
package io.redlink.utils.logging;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import org.slf4j.MDC;
/**
* Encapsulates a MDC logging context.
* When {@link #close() closing} a LoggingContext, the MDC is reset to the state it was when the
* LoggingContext was created.
*/
public class LoggingContext implements AutoCloseable {
private final Map previousContext;
/**
* Crates a new LoggingContext
*/
public LoggingContext() {
this(false);
}
/**
* Creates a new LoggingContext
* @param clear if {@code true} the new context will be empty.
*/
public LoggingContext(boolean clear) {
previousContext = MDC.getCopyOfContextMap();
if (clear) {
MDC.clear();
}
}
@Override
public void close() {
if (previousContext != null) {
MDC.setContextMap(previousContext);
} else {
MDC.clear();
}
}
/**
* Create a new, empty {@link LoggingContext}
*/
public static LoggingContext empty() {
return new LoggingContext(true);
}
/**
* Create a new {@link LoggingContext} populated with the current MDC entries
*/
public static LoggingContext create() {
return new LoggingContext(false);
}
public static Runnable wrap(final Runnable runnable) {
return wrap(runnable, false);
}
public static Runnable wrap(final Runnable runnable, boolean clear) {
return () -> {
try (LoggingContext ctx = new LoggingContext(clear)) {
runnable.run();
}
};
}
public static Callable wrap(final Callable callable) {
return wrap(callable, false);
}
public static Callable wrap(final Callable callable, boolean clear) {
return () -> {
try (LoggingContext ctx = new LoggingContext(clear)) {
return callable.call();
}
};
}
public static Function wrap(final Function function) {
return wrap(function, false);
}
public static Function wrap(final Function function, boolean clear) {
return (T t) -> {
try (LoggingContext ctx = new LoggingContext(clear)) {
return function.apply(t);
}
};
}
public static Supplier wrap(final Supplier supplier) {
return wrap(supplier, false);
}
public static Supplier wrap(final Supplier supplier, boolean clear) {
return () -> {
try (LoggingContext ctx = new LoggingContext(clear)) {
return supplier.get();
}
};
}
public static Consumer wrap(final Consumer consumer) {
return wrap(consumer, false);
}
public static Consumer wrap(final Consumer consumer, boolean clear) {
return (T t) -> {
try (LoggingContext ctx = new LoggingContext(clear)) {
consumer.accept(t);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy